Problem Definition The factorial of a number n (written n!) is the number times the factorial of itself minus one; and the factorial of 1 is, by definition, equals to 1. For example, the factorial of 2 is 2*1! = 2*1, and the factorial of 3 is 3*2! = 3*2*1 =6, and so on. Implementing this definition using a computer program is relatively slow, instead Sterling's formula is used as an approximation of the factorial for large values. Sterling's formula is defined as follows: n! = e-n n" V2 n n The exp(x) function in the math module gives the value ofe raised to the power of x Write a Python program that: 1. Requests the user to enter 5 integer values of n greater than 10. It should check: a. if all entered values are greater than 10, then your program should: output the message: "Thanks for entering 5 valid numbers greater than 10" calculate their factorial using sterling's formula allow the users to enter one of the following format types, which should be used to print the factorials of the numbers Default representation Scientific representation with default precision Non Scientific representation with 2 decimal precisions S N validate the format type: > if the format type is "Default representation", then it should v display the results in a tabular format using "Default representation" as shown in Figurel. > else if the format type is "Scientific representation with default precision", then it should V display the results in a tabular format using “Scientific representation with default precision" as shown in Figure2. > else if the format type is "Non Scientific representation", then it should V display the results in a tabular format using "Non Scientific representation with 2 decimal precisions" as shown in Figure3. otherwise, your program should display the following message as shown in Figure4: "You entered an invalid format type" b. otherwise, your program should output the following message: "Sorry you entered wrong numbers === ==> BYE"
Problem Definition The factorial of a number n (written n!) is the number times the factorial of itself minus one; and the factorial of 1 is, by definition, equals to 1. For example, the factorial of 2 is 2*1! = 2*1, and the factorial of 3 is 3*2! = 3*2*1 =6, and so on. Implementing this definition using a computer program is relatively slow, instead Sterling's formula is used as an approximation of the factorial for large values. Sterling's formula is defined as follows: n! = e-n n" V2 n n The exp(x) function in the math module gives the value ofe raised to the power of x Write a Python program that: 1. Requests the user to enter 5 integer values of n greater than 10. It should check: a. if all entered values are greater than 10, then your program should: output the message: "Thanks for entering 5 valid numbers greater than 10" calculate their factorial using sterling's formula allow the users to enter one of the following format types, which should be used to print the factorials of the numbers Default representation Scientific representation with default precision Non Scientific representation with 2 decimal precisions S N validate the format type: > if the format type is "Default representation", then it should v display the results in a tabular format using "Default representation" as shown in Figurel. > else if the format type is "Scientific representation with default precision", then it should V display the results in a tabular format using “Scientific representation with default precision" as shown in Figure2. > else if the format type is "Non Scientific representation", then it should V display the results in a tabular format using "Non Scientific representation with 2 decimal precisions" as shown in Figure3. otherwise, your program should display the following message as shown in Figure4: "You entered an invalid format type" b. otherwise, your program should output the following message: "Sorry you entered wrong numbers === ==> BYE"
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Write a problem, input, output, algorithm , comments and design a python program.

Transcribed Image Text:The purpose of this assignment is to practice the use of Python input/output, modules and selection
control structures to develop a solution to the given problem. Your solution will consist of two parts:
(i) your name, university id, section number and your program design (written as comments at the
beginning of your program by defining the problem statement, input/output data and the algorithm in
pseudocode), (ii) The corresponding Python program.
Problem Definition
The factorial of a number n (written n!) is the number times the factorial of itself minus one; and the
factorial of 1 is, by definition, equals to 1. For example, the factorial of 2 is 2*1! = 2*1, and the factorial
of 3 is 3*2! = 3*2*1=6, and so on. Implementing this definition using a computer program is relatively
slow, instead Sterling's formula is used as an approximation of the factorial for large values. Sterling's
formula is defined as follows:
n! = e-n
n" V2 πη
The exp(x) function in the math module gives the value of e raised to the power of x
Write a Python program that:
1. Requests the user to enter 5 integer values of n greater than 10. It should check:
a. if all entered values are greater than 10, then your program should:
• output the message: "Thanks for entering 5 valid numbers greater than 10"
calculate their factorial using sterling's formula
allow the users to enter one of the following format types, which should be used to
print the factorials of the numbers
Default representation
Scientific representation with default precision
Non Scientific representation with 2 decimal precisions
D
S
validate the format type:
> if the format type is "Default representation", then it should
V display the results in a tabular format using "Default representation" as
shown in Figurel.
else if the format type is "Scientific representation with default precision",
then it should
V display the results in a tabular format using "Scientific representation with
default precision" as shown in Figure2.
> else if the format type is "Non Scientific representation", then it should
V display the results in a tabular format using "Non Scientific representation
with 2 decimal precisions" as shown in Figure3.
otherwise, your program should display the following message as shown in
Figure4:
"You entered an invalid format type"
b. otherwise, your program should output the following message:
"Sorry you entered wrong numbers ===
=> BYE"
![Figurel shows a sample run of the program with valid numbers and Default representation format
type. Figure2 shows a sample run of the program with valid numbers and Scientific representation
format type. Figure3 shows a sample run of the program with valid numbers and Non Scientific
representation format type. Figure4 shows a sample run of an invalid format type and Figure5 shows
a sample run of the program with invalid numbers.
O Console 1/A D
O Console 1/AD
Please enter 5 integer numbers greater than 10: 11 12 13 14 15
Thanks for entering 5 valid numbers greater than 10
Please enter 5 integer numbers greater than 10: 11 12 13 14 15
Thanks for entering 5 valid numbers greater than 10
Please Enter the format type:
Please Enter the format type:
Defalut representation
Scientific representation and default precision
Non Scientific representation and 2 decimal precision
Defalut representation
Scientific representation and default precision
Non Scientific representation and 2 decimal precision
N
Output format:
Output format:
Default representation
Scientific representation
Precision - default:
Num
Sterling Value
Num
Sterling Value
======
3.961563e+07
11
12
13
14
15
39615625
475687486
6187239475
11
12
13
14
15
4.756875e+08
6.187239e+09
8.666100e+10
1.300431e•12
86661001740
1300430722199
In (2): |
Figurel: Sample run of the program with valid
numbers and Default representation format type.
In [24):
Figure2: Sample run of the program with valid
numbers and Scientific representation format type.
O Cansole 1/A O
O Console 1/A
Please enter 5 integer numbers greater than 10: 11 12 13 14 15
Thanks for entering 5 valid numbers greater than 10
In [21]: runfile('C:/zuhoor_Hard disk/Zuhoor 2020/COMP2101/Python
COMP2101_Spring2021/H\s/H2/H«2 Sample Solution SP2021_zuhoor.py', wdir='C:/
zuhoor_Hard disk/Zuhoor 2820/COMP2101/Python COMP2101_Spring2021/Hls/HN2" )
Please Enter the format type:
Defalut representation
Scientific representation and default precision
Non Scientific representation and 2 decimal precision
D
Please enter 5 integer numbers greater than 10: 11 12 13 14 15
Thanks for entering 5 valid numbers greater than 10
In
Output format:
Please Enter the format type:
Non-Scientific representation
Precision = 2:
Defalut represe
Scientific representation and default precision
Non Scientific representation and 2 decimal precision
D
Num
Sterling Value
11
12
13
14
15
39615625.05
k9
475687486.47
6187239475.19
86661001740. 60
1300430722199.47
You entered an invalid format type
In [22]:
**.. .. ..
Figure3: Sample run of the program with valid
numbers and Non Scientific representation format format type.
Figure4: Sample run of the program with invalid
type.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa4a47bf4-27e0-40d5-a5cd-5050e39c9f02%2Fecd419c5-cde2-407a-a3ca-318598458fd0%2Fr7wdfvf_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Figurel shows a sample run of the program with valid numbers and Default representation format
type. Figure2 shows a sample run of the program with valid numbers and Scientific representation
format type. Figure3 shows a sample run of the program with valid numbers and Non Scientific
representation format type. Figure4 shows a sample run of an invalid format type and Figure5 shows
a sample run of the program with invalid numbers.
O Console 1/A D
O Console 1/AD
Please enter 5 integer numbers greater than 10: 11 12 13 14 15
Thanks for entering 5 valid numbers greater than 10
Please enter 5 integer numbers greater than 10: 11 12 13 14 15
Thanks for entering 5 valid numbers greater than 10
Please Enter the format type:
Please Enter the format type:
Defalut representation
Scientific representation and default precision
Non Scientific representation and 2 decimal precision
Defalut representation
Scientific representation and default precision
Non Scientific representation and 2 decimal precision
N
Output format:
Output format:
Default representation
Scientific representation
Precision - default:
Num
Sterling Value
Num
Sterling Value
======
3.961563e+07
11
12
13
14
15
39615625
475687486
6187239475
11
12
13
14
15
4.756875e+08
6.187239e+09
8.666100e+10
1.300431e•12
86661001740
1300430722199
In (2): |
Figurel: Sample run of the program with valid
numbers and Default representation format type.
In [24):
Figure2: Sample run of the program with valid
numbers and Scientific representation format type.
O Cansole 1/A O
O Console 1/A
Please enter 5 integer numbers greater than 10: 11 12 13 14 15
Thanks for entering 5 valid numbers greater than 10
In [21]: runfile('C:/zuhoor_Hard disk/Zuhoor 2020/COMP2101/Python
COMP2101_Spring2021/H\s/H2/H«2 Sample Solution SP2021_zuhoor.py', wdir='C:/
zuhoor_Hard disk/Zuhoor 2820/COMP2101/Python COMP2101_Spring2021/Hls/HN2" )
Please Enter the format type:
Defalut representation
Scientific representation and default precision
Non Scientific representation and 2 decimal precision
D
Please enter 5 integer numbers greater than 10: 11 12 13 14 15
Thanks for entering 5 valid numbers greater than 10
In
Output format:
Please Enter the format type:
Non-Scientific representation
Precision = 2:
Defalut represe
Scientific representation and default precision
Non Scientific representation and 2 decimal precision
D
Num
Sterling Value
11
12
13
14
15
39615625.05
k9
475687486.47
6187239475.19
86661001740. 60
1300430722199.47
You entered an invalid format type
In [22]:
**.. .. ..
Figure3: Sample run of the program with valid
numbers and Non Scientific representation format format type.
Figure4: Sample run of the program with invalid
type.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
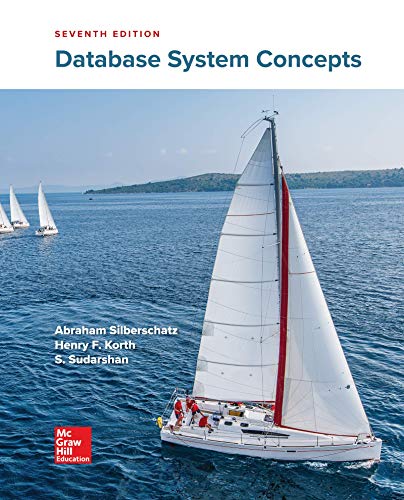
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
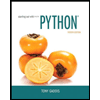
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
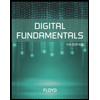
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
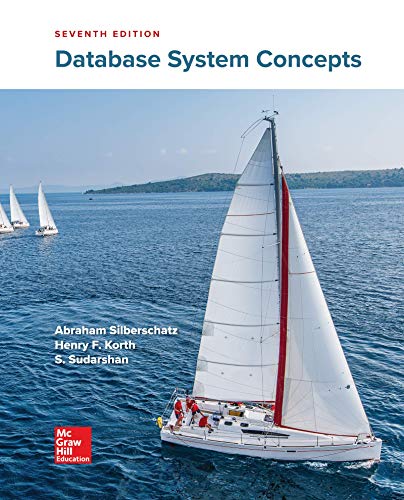
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
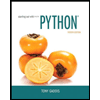
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
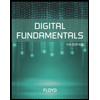
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
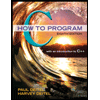
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
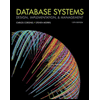
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
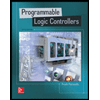
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education