Problem 8 We have seen the benefits of the 'fold' function for list data structures. In a similar fashion, write a function In [ ]: fold_inorder : 'a > 'b> 'a) -> 'a -> 'b tree -> 'a That does an inorder fold of the tree. The function should traverse the left subtree, visit the root, and then traverse the right subtree. For example, fold_inorder (fun acc x -> acc @ [x]) [] (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf,3,Leaf))) = [1;2;3] In [] let rec fold inorder f acc t = (* YOUR CODE HERE *) assert (fold_inorder (fun acc x -> acc @[x]) [] (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf, 3, Leaf))) = [1;2;3]); assert (fold_inorder (fun acc x -> acc + x) 0 (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf, 3, Leaf))) = 6)
Problem 8 We have seen the benefits of the 'fold' function for list data structures. In a similar fashion, write a function In [ ]: fold_inorder : 'a > 'b> 'a) -> 'a -> 'b tree -> 'a That does an inorder fold of the tree. The function should traverse the left subtree, visit the root, and then traverse the right subtree. For example, fold_inorder (fun acc x -> acc @ [x]) [] (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf,3,Leaf))) = [1;2;3] In [] let rec fold inorder f acc t = (* YOUR CODE HERE *) assert (fold_inorder (fun acc x -> acc @[x]) [] (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf, 3, Leaf))) = [1;2;3]); assert (fold_inorder (fun acc x -> acc + x) 0 (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf, 3, Leaf))) = 6)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
OCAML Problem
![### Problem 8
We have seen the benefits of the 'fold' function for list data structures. In a similar fashion, write a function:
```ocaml
fold_inorder : ('a -> 'b -> 'a) -> 'a -> 'b tree -> 'a
```
That does an inorder fold of the tree. The function should traverse the left subtree, visit the root, and then traverse the right subtree. For example,
```ocaml
fold_inorder (fun acc x -> acc @ [x]) [] (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf, 3, Leaf))) = [1; 2; 3]
```
```ocaml
let rec fold_inorder f acc t =
(* YOUR CODE HERE *)
```
```ocaml
assert (fold_inorder (fun acc x -> acc @ [x]) [] (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf, 3, Leaf))) = [1; 2; 3]);
assert (fold_inorder (fun acc x -> acc + x) 0 (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf, 3, Leaf))) = 6)
```
### Explanation
This problem involves implementing a higher-order function `fold_inorder` that performs an inorder traversal on a binary tree data structure. The function uses a fold operation, commonly employed to accumulate results across data structures like lists or trees. The snippet provides an OCaml function definition and two test assertions:
1. **Function Definition:**
- `fold_inorder` function takes three parameters:
- A function `f` of type `('a -> 'b -> 'a)`.
- An accumulator `acc` of type `'a`.
- A binary tree `t` of type `'b tree`.
- It recursively traverses the tree, applying the function `f` to accumulate a result using the inorder traversal pattern (left subtree, root, right subtree).
2. **Assertions:**
- The first assertion applies `fold_inorder` with a function that appends each tree element to an accumulator list, producing the list `[1; 2; 3]` from the given tree.
- The second assertion sums the values of the tree nodes, resulting in a total of `6`.
### Diagram Description
There](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F95a918cc-648a-4ba1-8333-a4ee16085cbc%2Fb7e220d9-ef9b-415a-af9b-301fea2b8baa%2Fgbss7pa_processed.png&w=3840&q=75)
Transcribed Image Text:### Problem 8
We have seen the benefits of the 'fold' function for list data structures. In a similar fashion, write a function:
```ocaml
fold_inorder : ('a -> 'b -> 'a) -> 'a -> 'b tree -> 'a
```
That does an inorder fold of the tree. The function should traverse the left subtree, visit the root, and then traverse the right subtree. For example,
```ocaml
fold_inorder (fun acc x -> acc @ [x]) [] (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf, 3, Leaf))) = [1; 2; 3]
```
```ocaml
let rec fold_inorder f acc t =
(* YOUR CODE HERE *)
```
```ocaml
assert (fold_inorder (fun acc x -> acc @ [x]) [] (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf, 3, Leaf))) = [1; 2; 3]);
assert (fold_inorder (fun acc x -> acc + x) 0 (Node (Node (Leaf, 1, Leaf), 2, Node (Leaf, 3, Leaf))) = 6)
```
### Explanation
This problem involves implementing a higher-order function `fold_inorder` that performs an inorder traversal on a binary tree data structure. The function uses a fold operation, commonly employed to accumulate results across data structures like lists or trees. The snippet provides an OCaml function definition and two test assertions:
1. **Function Definition:**
- `fold_inorder` function takes three parameters:
- A function `f` of type `('a -> 'b -> 'a)`.
- An accumulator `acc` of type `'a`.
- A binary tree `t` of type `'b tree`.
- It recursively traverses the tree, applying the function `f` to accumulate a result using the inorder traversal pattern (left subtree, root, right subtree).
2. **Assertions:**
- The first assertion applies `fold_inorder` with a function that appends each tree element to an accumulator list, producing the list `[1; 2; 3]` from the given tree.
- The second assertion sums the values of the tree nodes, resulting in a total of `6`.
### Diagram Description
There
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
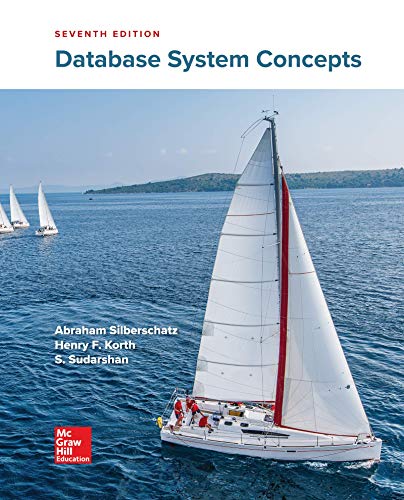
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
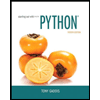
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
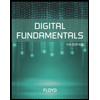
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
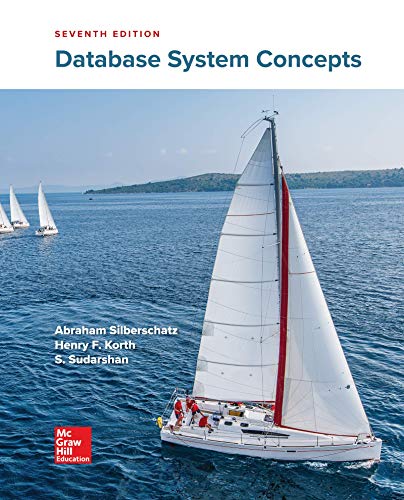
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
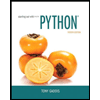
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
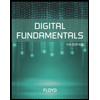
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
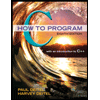
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
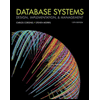
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
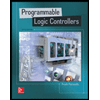
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education