Problem 7 Write a Python function to sort numbers in decreasing order, based on the following recursive strategy: Note: this strategy returns a list, which is in decreasing order. . Check the smallest case(s), and return the proper sorted output. The smallest cases is when you cannot reduce the input list in your recursive strategy. • Recursive strategy when the input L is still reducible in size: ▪ Find the largest number in the input list, L. Use Python's built-in max function to do this. ▪ Remove the largest number. Use Python's remove method of lists to do this. ▪ After you remove the largest, L has one item fewer, i.e. you've "reduced" the input size of L. ▪ Use "the same recursive strategy" to get a sorted list of the remaining numbers in L. ▪ Assemble the largest number and the sorted list of the remaining numbers to construct a sorted list of L. Examples: ● sort_max([10, 5, 7, 12]) returns [12, 10, 7, 5] sort_max([5]) returns [5] sort_max([]) returns []
Problem 7 Write a Python function to sort numbers in decreasing order, based on the following recursive strategy: Note: this strategy returns a list, which is in decreasing order. . Check the smallest case(s), and return the proper sorted output. The smallest cases is when you cannot reduce the input list in your recursive strategy. • Recursive strategy when the input L is still reducible in size: ▪ Find the largest number in the input list, L. Use Python's built-in max function to do this. ▪ Remove the largest number. Use Python's remove method of lists to do this. ▪ After you remove the largest, L has one item fewer, i.e. you've "reduced" the input size of L. ▪ Use "the same recursive strategy" to get a sorted list of the remaining numbers in L. ▪ Assemble the largest number and the sorted list of the remaining numbers to construct a sorted list of L. Examples: ● sort_max([10, 5, 7, 12]) returns [12, 10, 7, 5] sort_max([5]) returns [5] sort_max([]) returns []
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please written by computer source
![Examples of Python's functions:
• L = [1,4,10,3]
• max(L) returns 10
• L.remove(10) removes 10 from L. This method returns None.
• List concatenation: [1] + [2, 3] --> [1, 2, 3]
Note: this problem is not meant to produce the best way to sort a list. It's meant to orient you toward a recursive
mindset, which is helpful for more complex problems.
# Input: a list of numbers
# Output: the same list of numbers, but in decreasing order
#
def sort_max (L):
pass
Problem 8
Write down the running time equation, T(n), of the recursive strategy in Problem 7.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fed3c2e14-fffa-4a76-b24c-f02f86266ed7%2F560bc8ee-6082-444e-9527-09203f6cea8b%2Fw7mxaf_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Examples of Python's functions:
• L = [1,4,10,3]
• max(L) returns 10
• L.remove(10) removes 10 from L. This method returns None.
• List concatenation: [1] + [2, 3] --> [1, 2, 3]
Note: this problem is not meant to produce the best way to sort a list. It's meant to orient you toward a recursive
mindset, which is helpful for more complex problems.
# Input: a list of numbers
# Output: the same list of numbers, but in decreasing order
#
def sort_max (L):
pass
Problem 8
Write down the running time equation, T(n), of the recursive strategy in Problem 7.
![Problem 7
Write a Python function to sort numbers in decreasing order, based on the following recursive strategy:
• Note: this strategy returns a list, which is in decreasing order.
• Check the smallest case(s), and return the proper sorted output. The smallest cases is when you cannot reduce
the input list in your recursive strategy.
• Recursive strategy when the input L is still reducible in size:
▪ Find the largest number in the input list, L. Use Python's built-in max function to do this.
▪ Remove the largest number. Use Python's remove method of lists to do this.
▪ After you remove the largest, L has one item fewer, i.e. you've "reduced" the input size of L.
▪ Use "the same recursive strategy" to get a sorted list of the remaining numbers in L..
▪ Assemble the largest number and the sorted list of the remaining numbers to construct a sorted list of L.
Examples:
• sort_max([10, 5, 7, 12]) returns [12, 10, 7, 5]
• sort_max([5]) returns [5]
• sort_max([]) returns []](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fed3c2e14-fffa-4a76-b24c-f02f86266ed7%2F560bc8ee-6082-444e-9527-09203f6cea8b%2Fwo0f9zn_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Problem 7
Write a Python function to sort numbers in decreasing order, based on the following recursive strategy:
• Note: this strategy returns a list, which is in decreasing order.
• Check the smallest case(s), and return the proper sorted output. The smallest cases is when you cannot reduce
the input list in your recursive strategy.
• Recursive strategy when the input L is still reducible in size:
▪ Find the largest number in the input list, L. Use Python's built-in max function to do this.
▪ Remove the largest number. Use Python's remove method of lists to do this.
▪ After you remove the largest, L has one item fewer, i.e. you've "reduced" the input size of L.
▪ Use "the same recursive strategy" to get a sorted list of the remaining numbers in L..
▪ Assemble the largest number and the sorted list of the remaining numbers to construct a sorted list of L.
Examples:
• sort_max([10, 5, 7, 12]) returns [12, 10, 7, 5]
• sort_max([5]) returns [5]
• sort_max([]) returns []
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
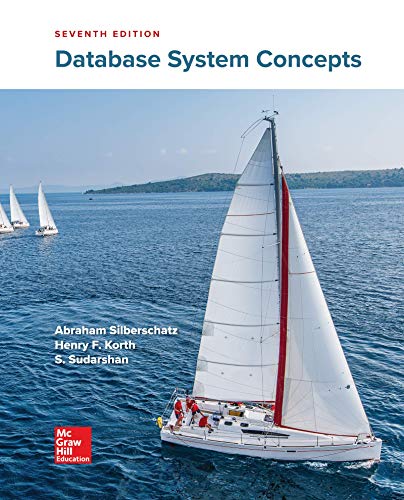
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
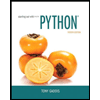
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
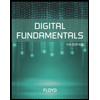
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
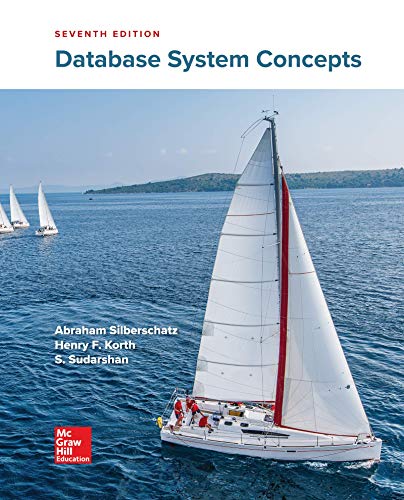
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
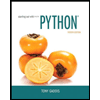
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
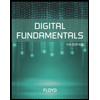
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
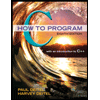
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
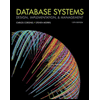
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
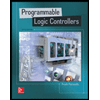
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education