Problem 2 Write a program in a language of your choice (preferably Python, or R). Using the following algorithm. procedure multiply(a, b: positive Integers) {the binary expansions of a and bare (an-1an-2...10) and (bn-bn-2...bibo)2, respectively) for j = 0 ton-1 if bj = 1 then c; := a shifted j places else c; := 0 (co, C1..... Cn-1 are the partial products} P:=0 for j:= 0 ton-1 P:=p+Cj return p (p is the value of ab}
Problem 2 Write a program in a language of your choice (preferably Python, or R). Using the following algorithm. procedure multiply(a, b: positive Integers) {the binary expansions of a and bare (an-1an-2...10) and (bn-bn-2...bibo)2, respectively) for j = 0 ton-1 if bj = 1 then c; := a shifted j places else c; := 0 (co, C1..... Cn-1 are the partial products} P:=0 for j:= 0 ton-1 P:=p+Cj return p (p is the value of ab}
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
python

Transcribed Image Text:## Problem 2: Multiplying Two Base 2 Numbers using an Algorithm in Python or R
### Task Description:
Write a program in a language of your choice (preferably Python or R) to multiply two positive integers using the following algorithm.
### Algorithm to Perform Multiplication:
```plaintext
procedure multiply(a, b: positive integers)
{the binary expansions of a and b are (a_n-1 a_n-2 ... a1 a0)₂ and (b_n-1 b_n-2 ... b1 b0)₂, respectively}
for j := 0 to n - 1
if b_j = 1 then c_j := a shifted j places
else c_j := 0
{c₀, c₁, ..., c_n-1 are the partial products}
p := 0
for j := 0 to n - 1
p := p + c_j
return p {p is the value of ab}
```
### Explanation:
1. **Binary Expansion**: The numbers `a` and `b` are represented in their binary formats.
2. **Iterate through Digits of b**:
- For each bit `b_j` of `b`:
- If `b_j` is 1, then calculate the partial product `c_j` by shifting `a` left by `j` positions.
- If `b_j` is 0, then the partial product `c_j` is 0.
3. **Sum Partial Products**: The final product `p` is obtained by summing all the partial products `c_j`.
4. **Return the Result**: The result `p` is the multiplication of `a` and `b`.
### Diagrammatic Representation:
Figure 2: Multiplying two base 2 numbers.
This algorithm effectively leverages the properties of binary numbers to perform the multiplication by bitwise shifts and accumulation.
By following this algorithm, you can implement a program to perform binary multiplication in any programming language of your choice, such as Python or R.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
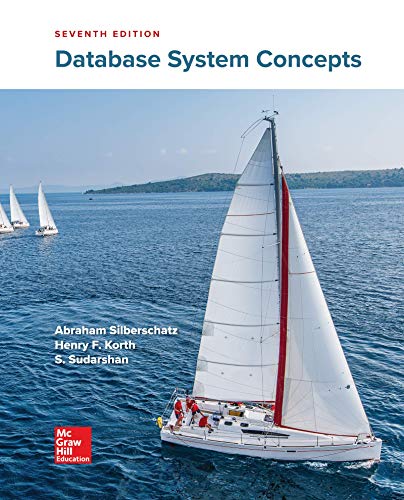
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
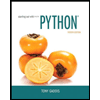
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
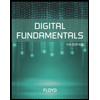
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
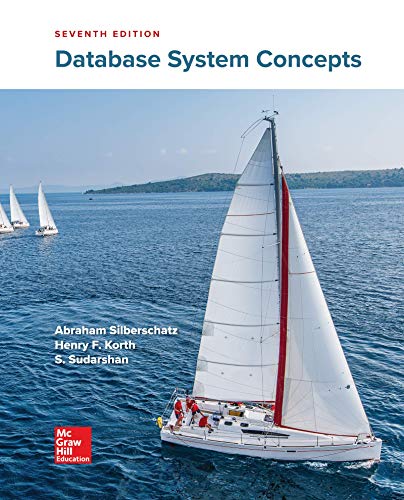
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
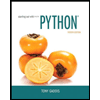
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
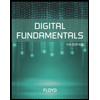
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
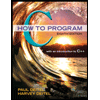
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
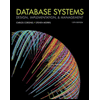
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
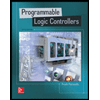
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education