Problem 1. Consider the following code example for allocating and releasing, which can be concurrently called by multiple processes to fork new processes. #define MAX_PROCESSES 255 int number_of_processes = 0; // a shared variable /* the implementation of fork() calls this function */ int allocate_process() { int new_pid; if (number_of_processes == MAX_PROCESSES) return -1; else { /* code to allocate necessary process resources */ ++number_of_processes; return new_pid; } } /* the implementation of exit() calls this function */ void release_process() { /* code to release process resources */ --number_of_processes; } Using the Semaphore structure and its atomic wait() and signal() operations to solve a critical section (mutual exclusion) problem. To prevent race condition(s) and solve the critical section problem in the code given above, (Hints: 1. The code here is NOT all the same as an example used in class; 2. In this problem, the Semaphore software tool needs to be used as a Binary Semaphore (Case 1) and the use of a Binary Semaphore (Case 1) to solve a critical section (mutual exclusion) problem is illustrated in the in-class lecture notes.) 1. WRITE C statements to declare and initialize a Semaphore variable named proc_S using the Semaphore structure defined in Slide 3.25 2. RE-WRITE the allocate_process() function by calling wait() and/or signal()defined in Slide 3.26 3. RE-WRITE the release_process() function by calling wait() and/or signal() defined in Slide 3.26
Problem 1. Consider the following code example for allocating and releasing, which can be concurrently called by multiple processes
to fork new processes.
#define MAX_PROCESSES 255
int number_of_processes = 0; // a shared variable
/* the implementation of fork() calls this function */
int allocate_process() {
int new_pid;
if (number_of_processes == MAX_PROCESSES)
return -1;
else {
/* code to allocate necessary process resources */
++number_of_processes;
return new_pid;
}
}
/* the implementation of exit() calls this function */
void release_process() {
/* code to release process resources */
--number_of_processes;
}
Using the Semaphore structure and its atomic wait() and signal() operations to solve a critical section (mutual exclusion)
problem. To prevent race condition(s) and solve the critical section problem in the code given above,
(Hints: 1. The code here is NOT all the same as an example used in class; 2. In this problem, the Semaphore software tool needs to be
used as a Binary Semaphore (Case 1) and the use of a Binary Semaphore (Case 1) to solve a critical section (mutual exclusion)
problem is illustrated in the in-class lecture notes.)
1. WRITE C statements to declare and initialize a Semaphore variable named proc_S using the Semaphore structure defined in
Slide 3.25
2. RE-WRITE the allocate_process() function by calling wait() and/or signal()defined in Slide 3.26
3. RE-WRITE the release_process() function by calling wait() and/or signal() defined in Slide 3.26
![Semaphore: a software tool
■ Synchronization tool that provides more sophisticated ways (than Mutex
locks) for process to synchronize their activities.
■A semaphore Scontains an integer variable
■S, apart from initialization, can only be accessed via two indivisible
(atomic) operations
25
● wait () and signal(): its execution cannot be interrupted
> Originally called P() and v() (by Dutch computer scientists)
• s: its value indicates the number of available identical instances of a
shared resource
To control access to a shared variable, the value of s is normally
initialized as 1 since there is only one copy of this variable in memory
Definition of the semaphore
typedef struct {
int value: //if neg, [value] is # of waiting processes
struct process list; // NULL for an empty list
// a list of sleeping processes while waiting
semaphore:
Operating System Concepts - 10-
Semaphore (cont.)
Definition of the wait () operation
wait (semaphore *S) {
S->value--;
if (8->value < 0) {
add this process to S->list;
sleep(); //suspend the process that invokes it
//greatly reduce busy waiting
Definition of the signal () operation
signal (semaphore *S) {
S->value++;
if (8->value <= 0) (//1 or more processes were waiting
remove a process P from S->list;
wakeup (P); //move P back to ready queue/running state
Operating System Concepts-10-Ein
3.36](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc50c96ee-5887-48c2-8667-c783798420fc%2Fe5fbb3a6-b879-4481-8c14-bf40c4ab73d3%2Fe8caxrm_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

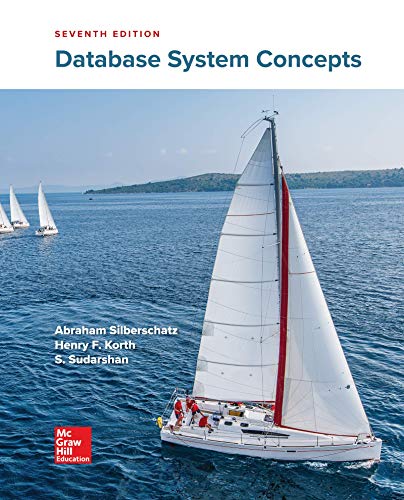
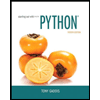
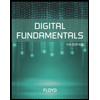
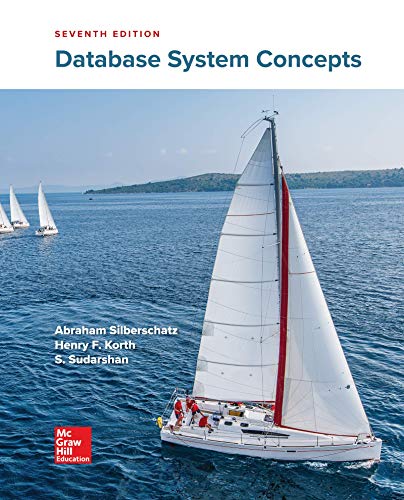
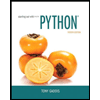
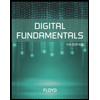
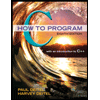
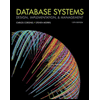
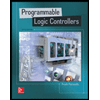