PROBLEM 1 The United States census estimates the population of the US every decade. These population estimates are recorded in the file population.csv. You can load this file with the code data = readmatrix('population.csv'); t data (1, :); N= data (2, ); in MATLAB, or data np.genfromtxt ('population.csv', delimiter=',') t = data[0, :] Ndata [1, :] in python. The variables t and N will be 1 × 24 vectors in MATLAB and 1D arrays with 24 entries in python. The entries of t represent time in years since 1900. For example, t = 0 represents the year 1900, t = −110 represents the year 1790 and t = 120 represents the year 2020. The times are all evenly spaced. The entries of N represent the population of the United States in millions at the corresponding year. (That is, the first entry in N is the population at the first time in t, the second entry in N is the population at the second time in t, etc.). Presumably, the population of the US is a function of time N(t), but we do not know the formula. All we know is the data in N. In this problem, we are going to estimate the growth rate of the US population by approximating dN/dt at different times. You should use a O(h2) approximation for all of these derivatives. (you can find the list of formulas in the theory notes). You should be able to determine the correct At from the data. Notice that you can't just apply these methods blindly. Think about whether you need a forward, backward, or central scheme - I realize I didn't explicitly say when to use what scheme, but that "aha!" moment is a crucial part of learning. (1) Estimated at the year 2020 and save your approximation in a variable named A1. dt dN dt dN (2) Estimate at the year 1880 and save your approximation in a variable named A2. (3) Estimate at the year 1790 and save your approximation in a variable named A3. (4) Estimate dN dt at every year in t. Save your answers in a 1 × 24 row vector (don't forget to use reshape in python) named A4. Your answers should be in the same order as the data, so the kth entry of A4 should be the derivative at the time in the kth entry of t. Notice that you already calculated the derivative for 1790 and 2020 above, so those will be your first and last entries. N' (t) (5) The per capita growth rate at time t is given by N. Use your approximations of the derivative to calculate the per capita growth rate at every year in t. Save your answers in a 1×24 row vector (don't forget to use reshape in python) named A5. Your answers should be in the same order as the data, so the kth entry of A5 should be the growth rate at the time in the kth entry of t. (6) Find the mean of all of these per capita growth rates and save it in a variable named A6. 1
PROBLEM 1 The United States census estimates the population of the US every decade. These population estimates are recorded in the file population.csv. You can load this file with the code data = readmatrix('population.csv'); t data (1, :); N= data (2, ); in MATLAB, or data np.genfromtxt ('population.csv', delimiter=',') t = data[0, :] Ndata [1, :] in python. The variables t and N will be 1 × 24 vectors in MATLAB and 1D arrays with 24 entries in python. The entries of t represent time in years since 1900. For example, t = 0 represents the year 1900, t = −110 represents the year 1790 and t = 120 represents the year 2020. The times are all evenly spaced. The entries of N represent the population of the United States in millions at the corresponding year. (That is, the first entry in N is the population at the first time in t, the second entry in N is the population at the second time in t, etc.). Presumably, the population of the US is a function of time N(t), but we do not know the formula. All we know is the data in N. In this problem, we are going to estimate the growth rate of the US population by approximating dN/dt at different times. You should use a O(h2) approximation for all of these derivatives. (you can find the list of formulas in the theory notes). You should be able to determine the correct At from the data. Notice that you can't just apply these methods blindly. Think about whether you need a forward, backward, or central scheme - I realize I didn't explicitly say when to use what scheme, but that "aha!" moment is a crucial part of learning. (1) Estimated at the year 2020 and save your approximation in a variable named A1. dt dN dt dN (2) Estimate at the year 1880 and save your approximation in a variable named A2. (3) Estimate at the year 1790 and save your approximation in a variable named A3. (4) Estimate dN dt at every year in t. Save your answers in a 1 × 24 row vector (don't forget to use reshape in python) named A4. Your answers should be in the same order as the data, so the kth entry of A4 should be the derivative at the time in the kth entry of t. Notice that you already calculated the derivative for 1790 and 2020 above, so those will be your first and last entries. N' (t) (5) The per capita growth rate at time t is given by N. Use your approximations of the derivative to calculate the per capita growth rate at every year in t. Save your answers in a 1×24 row vector (don't forget to use reshape in python) named A5. Your answers should be in the same order as the data, so the kth entry of A5 should be the growth rate at the time in the kth entry of t. (6) Find the mean of all of these per capita growth rates and save it in a variable named A6. 1
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Don't use ai to answer I will report your answer Solve it Asap with explanation and calculation
![PROBLEM 1
The United States census estimates the population of the US every decade. These population estimates
are recorded in the file population.csv. You can load this file with the code
data = readmatrix('population.csv');
t data (1, :);
N= data (2, );
in MATLAB, or
data
np.genfromtxt ('population.csv', delimiter=',')
t = data[0, :]
Ndata [1, :]
in python. The variables t and N will be 1 × 24 vectors in MATLAB and 1D arrays with 24 entries in python.
The entries of t represent time in years since 1900. For example, t = 0 represents the year 1900, t = −110
represents the year 1790 and t = 120 represents the year 2020. The times are all evenly spaced. The entries
of N represent the population of the United States in millions at the corresponding year. (That is, the first
entry in N is the population at the first time in t, the second entry in N is the population at the second time
in t, etc.).
Presumably, the population of the US is a function of time N(t), but we do not know the formula. All
we know is the data in N. In this problem, we are going to estimate the growth rate of the US population
by approximating dN/dt at different times. You should use a O(h2) approximation for all of these
derivatives. (you can find the list of formulas in the theory notes). You should be able to determine
the correct At from the data. Notice that you can't just apply these methods blindly. Think about whether
you need a forward, backward, or central scheme - I realize I didn't explicitly say when to use what scheme,
but that "aha!" moment is a crucial part of learning.
(1) Estimated at the year 2020 and save your approximation in a variable named A1.
dt
dN
dt
dN
(2) Estimate at the year 1880 and save your approximation in a variable named A2.
(3) Estimate at the year 1790 and save your approximation in a variable named A3.
(4) Estimate
dN
dt
at every year in t. Save your answers in a 1 × 24 row vector (don't forget to use reshape
in python) named A4. Your answers should be in the same order as the data, so the kth entry of A4
should be the derivative at the time in the kth entry of t. Notice that you already calculated the
derivative for 1790 and 2020 above, so those will be your first and last entries.
N' (t)
(5) The per capita growth rate at time t is given by N. Use your approximations of the derivative to
calculate the per capita growth rate at every year in t. Save your answers in a 1×24 row vector (don't
forget to use reshape in python) named A5. Your answers should be in the same order as the data, so
the kth entry of A5 should be the growth rate at the time in the kth entry of t.
(6) Find the mean of all of these per capita growth rates and save it in a variable named A6.
1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F729f6c88-7e1b-4867-9048-5dc4d327d39c%2F6561adc4-45f9-4c0d-99fc-cb74c0b44a66%2Fvobba8p_processed.jpeg&w=3840&q=75)
Transcribed Image Text:PROBLEM 1
The United States census estimates the population of the US every decade. These population estimates
are recorded in the file population.csv. You can load this file with the code
data = readmatrix('population.csv');
t data (1, :);
N= data (2, );
in MATLAB, or
data
np.genfromtxt ('population.csv', delimiter=',')
t = data[0, :]
Ndata [1, :]
in python. The variables t and N will be 1 × 24 vectors in MATLAB and 1D arrays with 24 entries in python.
The entries of t represent time in years since 1900. For example, t = 0 represents the year 1900, t = −110
represents the year 1790 and t = 120 represents the year 2020. The times are all evenly spaced. The entries
of N represent the population of the United States in millions at the corresponding year. (That is, the first
entry in N is the population at the first time in t, the second entry in N is the population at the second time
in t, etc.).
Presumably, the population of the US is a function of time N(t), but we do not know the formula. All
we know is the data in N. In this problem, we are going to estimate the growth rate of the US population
by approximating dN/dt at different times. You should use a O(h2) approximation for all of these
derivatives. (you can find the list of formulas in the theory notes). You should be able to determine
the correct At from the data. Notice that you can't just apply these methods blindly. Think about whether
you need a forward, backward, or central scheme - I realize I didn't explicitly say when to use what scheme,
but that "aha!" moment is a crucial part of learning.
(1) Estimated at the year 2020 and save your approximation in a variable named A1.
dt
dN
dt
dN
(2) Estimate at the year 1880 and save your approximation in a variable named A2.
(3) Estimate at the year 1790 and save your approximation in a variable named A3.
(4) Estimate
dN
dt
at every year in t. Save your answers in a 1 × 24 row vector (don't forget to use reshape
in python) named A4. Your answers should be in the same order as the data, so the kth entry of A4
should be the derivative at the time in the kth entry of t. Notice that you already calculated the
derivative for 1790 and 2020 above, so those will be your first and last entries.
N' (t)
(5) The per capita growth rate at time t is given by N. Use your approximations of the derivative to
calculate the per capita growth rate at every year in t. Save your answers in a 1×24 row vector (don't
forget to use reshape in python) named A5. Your answers should be in the same order as the data, so
the kth entry of A5 should be the growth rate at the time in the kth entry of t.
(6) Find the mean of all of these per capita growth rates and save it in a variable named A6.
1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
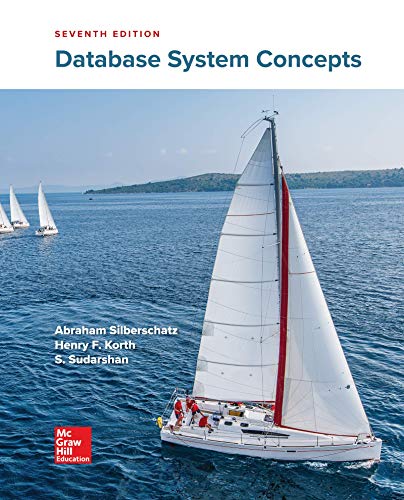
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
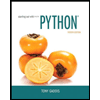
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
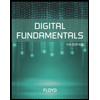
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
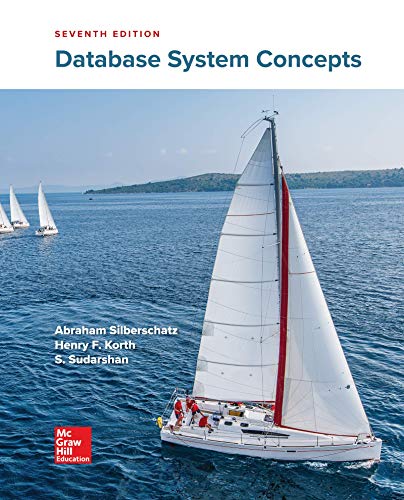
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
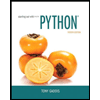
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
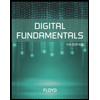
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
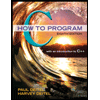
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
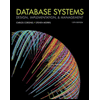
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
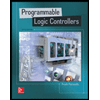
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education