PrintMeFirst.h o SavingsAccount.h SavingsAccount.cpp CheckingAccount.h CheckingAccount.cpp BankAccount.h BankAccount.cpp Makefile 1 P/* CheckingAccount.h 2 * Header file for CheckingAccount class 3 * efile CheckingAccount.h @author Javaria Basit 5 6 Q#ifndef CHECKINGACCOUNT_H #define CHECKINGACCOUNT H #include "BankAccount.h" 9 10 using nanespace std; 11 eclass CheckingAccount :public BankAccount { 12 13 14 15 private: int transactions; void check_for_fee(); public: 16 17 18 19 20 21 CheckingAccount(string, double); void withdraw(double); void deposit(double); void nonth_end(); }; 22 23 24 #endif rectory: /home/cnet/Lab 7)
PrintMeFirst.h o SavingsAccount.h SavingsAccount.cpp CheckingAccount.h CheckingAccount.cpp BankAccount.h BankAccount.cpp Makefile 1 P/* CheckingAccount.h 2 * Header file for CheckingAccount class 3 * efile CheckingAccount.h @author Javaria Basit 5 6 Q#ifndef CHECKINGACCOUNT_H #define CHECKINGACCOUNT H #include "BankAccount.h" 9 10 using nanespace std; 11 eclass CheckingAccount :public BankAccount { 12 13 14 15 private: int transactions; void check_for_fee(); public: 16 17 18 19 20 21 CheckingAccount(string, double); void withdraw(double); void deposit(double); void nonth_end(); }; 22 23 24 #endif rectory: /home/cnet/Lab 7)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help with documentation for my header files
I need documentation for each line.

Transcribed Image Text:```cpp
/*
* CheckingAccount.h
* Header file for CheckingAccount class
* @file CheckingAccount.h
* @author Javaria Basit
*/
#ifndef CHECKINGACCOUNT_H
#define CHECKINGACCOUNT_H
#include "BankAccount.h"
using namespace std;
class CheckingAccount : public BankAccount {
private:
int transactions;
void check_for_fee();
public:
CheckingAccount();
CheckingAccount(string, double);
void withdraw(double);
void deposit(double);
void month_end();
};
#endif
```
### Explanation
This C++ header file, `CheckingAccount.h`, defines a `CheckingAccount` class which inherits from a `BankAccount` class. Here are the main components:
- **Include Guards**:
- `#ifndef CHECKINGACCOUNT_H` and `#define CHECKINGACCOUNT_H` are used to prevent multiple inclusions of this file.
- **Includes**:
- `#include "BankAccount.h"`: This includes the header file for the base class `BankAccount`.
- **Namespace**:
- `using namespace std;` allows usage of the standard namespace.
- **Class Structure**:
- **Private Members**:
- `int transactions;` — to hold the number of transactions.
- `void check_for_fee();` — a method to apply fees based on transactions.
- **Public Members**:
- `CheckingAccount();` — default constructor.
- `CheckingAccount(string, double);` — constructor with parameters for account initialization.
- `void withdraw(double);` — method to withdraw funds.
- `void deposit(double);` — method to deposit funds.
- `void month_end();` — method to handle end of month processes.
- **End of File**:
- `#endif` closes the include guard.
### Compilation Information
- The compiler output shows that various object files were generated for the different classes and linked into an executable named `bankTest`.
- The compilation finished successfully, indicating no syntax errors were present.

Transcribed Image Text:```
/* SavingsAccount.h
* Header file for SavingsAccount class
* @file SavingsAccount.h
* @author Javaria Basit
* This file SavingsAccount.h consist of class SavingsAccount and it is a private class
* This is also a derived class for the base class BankAccount
*/
#ifndef SAVINGSACCOUNT_H
#define SAVINGSACCOUNT_H
#include "BankAccount.h"
using namespace std;
class SavingsAccount : public BankAccount {
private:
double interest_rate;
double min_balance;
public:
SavingsAccount();
SavingsAccount(string, double, double);
void withdraw(double);
void set_interest_rate(double);
double get_interest_rate();
void display_balance();
void month_end();
};
#endif
```
**Explanation:**
- The code is a header file in C++ defining the `SavingsAccount` class.
- It inherits from a base class `BankAccount`.
- It includes private member variables `interest_rate` and `min_balance`.
- Public member functions include constructors, methods to withdraw, set and get interest rate, display balance, and handle end-of-month operations.
- The code uses preprocessor directives to avoid multiple inclusions.
- The compilation in the bottom indicates no errors: "Compilation finished successfully."
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
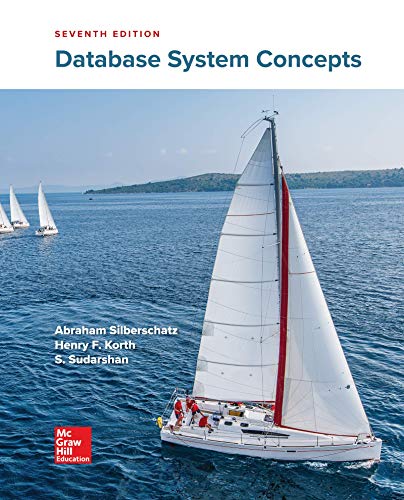
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
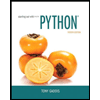
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
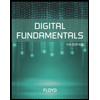
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
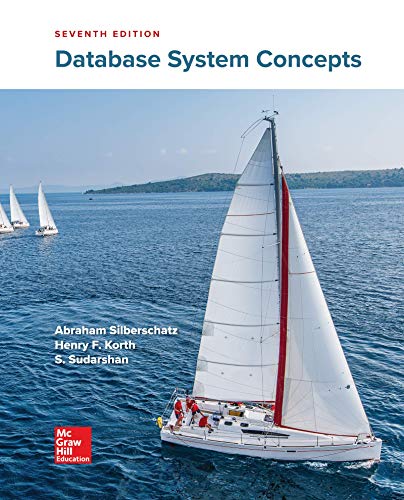
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
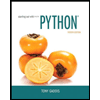
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
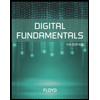
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
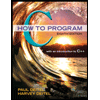
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
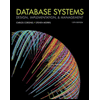
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
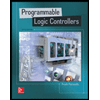
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education