PLEASE TRACE BY HAND OR SHOW WHAT EACH VALUE IS ASSIGNED TO and SHOW the OUTPUT for the following code: THE USER INPUT IS: 87 45 for the CODE THAT FOLLOWS: #include using namespace std; class twoNums { public: void setOne (int); // sets the private member one to the value of the parameter void setTwo (int); // sets the private member two to the value of the parameter void nicePrint(); // prints the values of the both private members, one first followed by two, // with 2 blank-spaces between then moves to a new line private: int one; int two; }; int main( ) { cout << "==========\n"; twoNums *ptr; int numONE, numTWO; ptr = new twoNums; ptr->setOne(numTWO); ptr->setTwo(numONE); ptr->nicePrint(); cout << "Please input two numbers separated by a space." << endl; cin >> numTWO >> numONE; ptr->setOne(numTWO); ptr->setTwo(numONE); ptr->nicePrint(); system (“Pause”); return 0; }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
PLEASE TRACE BY HAND OR SHOW WHAT EACH VALUE IS ASSIGNED TO and SHOW the OUTPUT for the following code:
THE USER INPUT IS: 87 45 for the CODE THAT FOLLOWS:
#include<iostream>
using namespace std;
class twoNums
{
public:
void setOne (int); // sets the private member one to the value of the parameter
void setTwo (int); // sets the private member two to the value of the parameter
void nicePrint(); // prints the values of the both private members, one first followed by two,
// with 2 blank-spaces between then moves to a new line
private:
int one;
int two;
};
int main( )
{ cout << "==========\n";
twoNums *ptr;
int numONE, numTWO;
ptr = new twoNums;
ptr->setOne(numTWO);
ptr->setTwo(numONE);
ptr->nicePrint();
cout << "Please input two numbers separated by a space." << endl;
cin >> numTWO >> numONE;
ptr->setOne(numTWO);
ptr->setTwo(numONE);
ptr->nicePrint();
system (“Pause”);
return 0; }

Step by step
Solved in 4 steps with 1 images

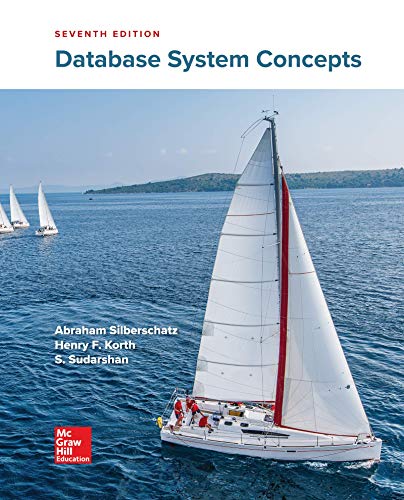
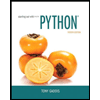
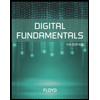
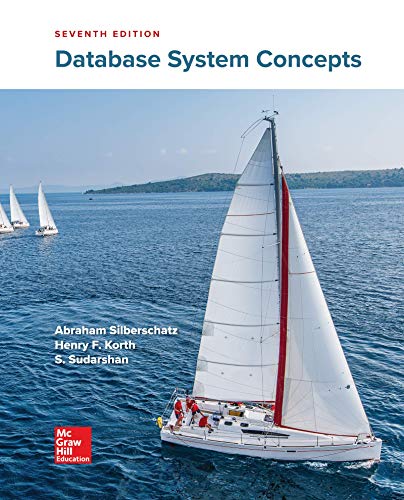
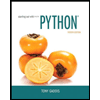
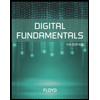
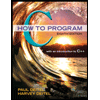
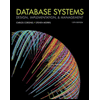
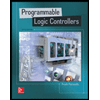