please write in C++ using #include using namespace std Define a class called DateYear, that represents any year like 2023 in addition to the day and month (03/34/2023). Your class will have one member variable of type int to represent the year. This class is a child of the DayOf Year class where the code is given in lecture14 (Lecture14_Code.cpp). Your DateYear class should have the following member functions: a constructor and a default constructor that sets the year to this year, month to 1 and day to 1. an input function that reads the date (day, month, and year). Make sure to check for inaccurate dates. -getter and setters for the year. an output function that outputs the date in form of xx/xx/xxxx. It can display to the screen or the file. A friend function DateCompare that compares two years and returns true if they are the same. A member function BDCountdown, which calculates and returns how many days till your birthday. This function take the birthday as the input parameter and returns the number of days left to the birthday which should be less than 365. Take note of the month in Gregorian calendar. The Gregorian calendar consists of the following 12 months: January 31 days February-28 days in a common year and 29 days in leap years March 31 days April - 30 days May 31 days June - 30 days July-31 days August 31 days September - 30 days October 31 days November 30 days December - 31 days You can put the days in an array to access them for computing the number of days. Embed your class definition in a test program. The test program should test all the attributes (every single function and all the data should be tested). All your inputs and outputs can be done using the keyboard and the screen. Even though your input/output functions can stream through files. So, how many days is left to your birthday? Note1: friend functions are not inheritable (the friend functions in lecture 14 code cannot be inherited by DateYear class) Note2: you can reuse function names and tailor them to the needs of the derived class.
please write in C++ using #include <iostream> using namespace std
Define a class called DateYear, that represents any year like 2023 in addition to the day and month (03/34/2023). Your class will have one member variable of type int to represent the year.
This class is a child of the DayOf Year class where the code is given in lecture14 (Lecture14_Code.cpp).
Your DateYear class should have the following member functions:
a constructor and a default constructor that sets the year to this year, month to 1 and day to 1.
an input function that reads the date (day, month, and year). Make sure to check for inaccurate dates.
-getter and setters for the year.
an output function that outputs the date in form of xx/xx/xxxx. It can display to the screen or the file.
A friend function DateCompare that compares two years and returns true if they are the same.
A member function BDCountdown, which calculates and returns how many days till your birthday. This function take the birthday as the input parameter and returns the number of days left to the birthday which should be less than 365. Take note of the month in Gregorian calendar. The Gregorian calendar consists of the following 12 months:
January 31 days
February-28 days in a common year and 29 days in leap years
March 31 days
April - 30 days
May 31 days
June - 30 days
July-31 days
August 31 days
September - 30 days
October 31 days
November 30 days
December - 31 days
You can put the days in an array to access them for computing the number of days. Embed your class definition in a test program. The test program should test all the attributes (every single function and all the data should be tested).
All your inputs and outputs can be done using the keyboard and the screen. Even though your input/output functions can stream through files.
So, how many days is left to your birthday?
Note1: friend functions are not inheritable (the friend functions in lecture 14 code cannot be inherited by DateYear class)
Note2: you can reuse function names and tailor them to the needs of the derived class.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

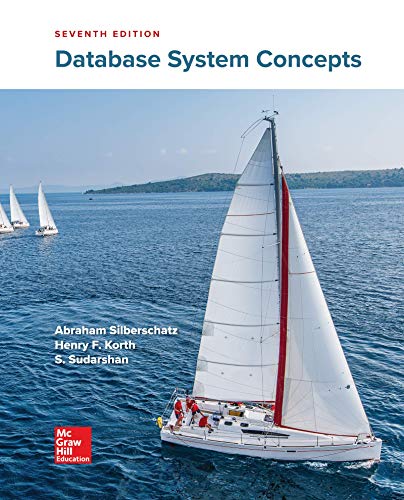
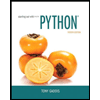
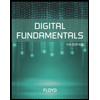
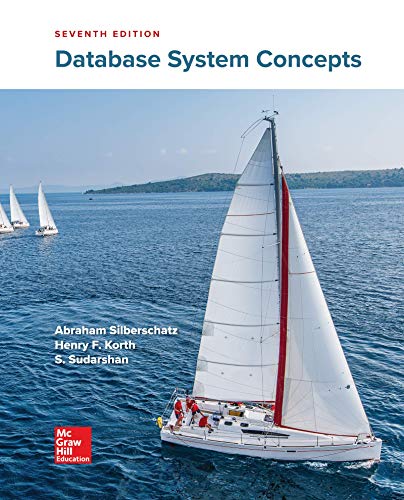
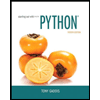
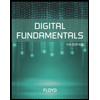
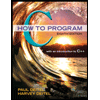
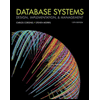
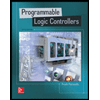