Please provide correct solution. Task1: Solve the producer and consumer problem with inter thread communication (join(), wait(), sleep() etc.) modifying the given C code.
Please provide correct solution.
Task1:
Solve the producer and consumer problem with inter thread communication (join(), wait(), sleep() etc.) modifying the given C code.
#include <pthread.h>
#include <stdio.h>
#include <string.h>
#define MAX 10 //producers and consumers can produce and consume upto MAX
#define BUFLEN 6
#define NUMTHREAD 2 /* number of threads */
void * consumer(int *id);
void * producer(int *id);
char buffer[BUFLEN];
char source[BUFLEN]; //from this array producer will store it's production into buffer
int pCount = 0;
int cCount = 0;
int buflen;
//initializing pthread mutex and condition variables
pthread_mutex_t count_mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t nonEmpty = PTHREAD_COND_INITIALIZER;
pthread_cond_t full = PTHREAD_COND_INITIALIZER;
int thread_id[NUMTHREAD] = {0,1};
int i = 0;
int j = 0;
main()
{
int i;
/* define the type to be pthread */
pthread_t thread[NUMTHREAD];
strcpy(source,"abcdef");
buflen = strlen(source);
/* create 2 threads*/
/* create one consumer and one producer */
/* define the properties of multi threads for both threads */
//Write Code Here
}
void * producer(int *id)
{
/*
- Producer stores the values in the buffer (Here copies values from source[] to buffer[]).
- Use mutex and thread communication (wait(), sleep() etc.) for the critical section.
- Print which producer is storing which values using which thread inside the critical section.
- Producer can produce up to MAX
*/
//Write code here
}
void * consumer(int *id)
{
/*
- Consumer takes out the value from the buffer and makes it empty.
- Use mutex and thread communication (wait(), sleep() etc.) for critical section
- Print which consumer is taking which values using which thread inside the critical section.
- Consumer can consume up to MAX
*/
//Write code here
}
Example Output:
0 produced a by Thread 0
1 produced b by Thread 0
0 consumed a by Thread 1
2 produced c by Thread 0
1 consumed b by Thread 1
3 produced d by Thread 0
4 produced e by Thread 0
2 consumed c by Thread 1
5 produced f by Thread 0
6 produced a by Thread 0
3 consumed d by Thread 1
7 produced b by Thread 0
8 produced c by Thread 0
9 produced d by Thread 0
4 consumed e by Thread 1

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

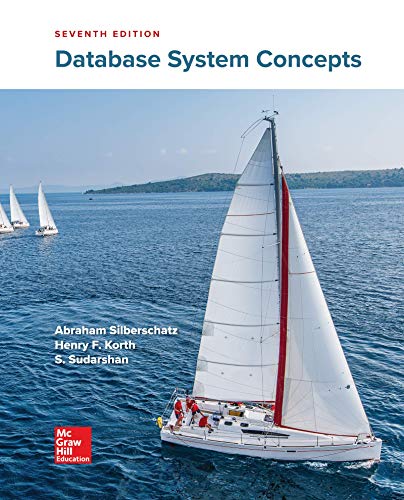
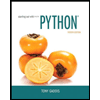
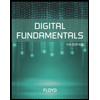
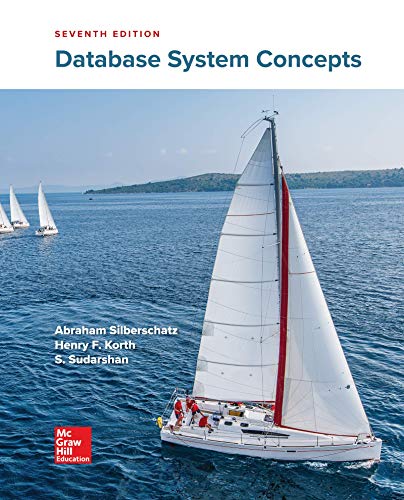
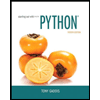
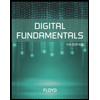
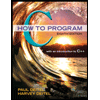
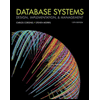
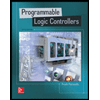