Please help, write the code for the test cases: public class JunitTest_RideRequestTest { // Test parameterized constructor with invalid input @Test public void test_2_0() { try { RideRequest request = new RideRequest(new String()); fail("You should throw an exception If the input is null or empty."); } catch (IllegalArgumentException e) { // exception excepted do nothing } } @Test public void test_2_2() { RideRequest request = null; try { RideRequest request2 = new RideRequest(request); fail("You should throw an exception If the input is null."); } catch (IllegalArgumentException e) { // exception excepted do nothing } } @Test public void test_2_3() { String s = "John , Downtown , 50.0 , Y , extra info"; try { RideRequest request2 = new RideRequest(s); fail("You should throw an exception If the input is not in correct format."); } catch (IllegalArgumentException e) { // exception excepted do nothing } } @Test public void test_2_4() { String s = null; try { RideRequest request2 = new RideRequest(s); fail("You should throw an exception If the input is null."); } catch (IllegalArgumentException e) { // exception excepted do nothing } } @Test public void test_2_5() { RideRequest request = new RideRequest(" Jack , North York , 9.99 , N "); request.setCustomerName("Bob"); request.setRideDetails("Airport"); request.setRidePrice(39.39); request.setHasDiscount(true); RideRequest copyRequest = new RideRequest(request); String errorMsg = String.format( "\n Test copy constructor failed. Returned CustomerName (%s) " + "but correct CustomerName is (%s)", copyRequest.getCustomerName(), "Bob"); assertEquals(errorMsg,"Bob", request.getCustomerName()); errorMsg = String.format( "\n Test copy constructor failed. Returned RideDetails (%s) " + "but correct RideDetails is (%s)", copyRequest.getRideDetails(), "Airport"); assertEquals(errorMsg, "Airport", copyRequest.getRideDetails()); assertEquals("Error: The constructor failed to set the ride price.",39.39, copyRequest.getRidePrice(), 0.0); assertTrue("Error: The constructor failed to set the ride discount.",copyRequest.isHasDiscount()); } @Test public void test_3_0() { RideRequest request = new RideRequest("John , Downtown , 50.0 , Y "); request.setCustomerName("Alice"); assertEquals("Set Customer Name method failed to update the customer name.", "Alice", request.getCustomerName()); } @Test public void test_3_1() { RideRequest request = new RideRequest("John , Scarborough , 50.0 , Y "); request.setCustomerName("Very Long Customer Name Exceeding Limit"); assertEquals("Set Customer Name method failed. Customer name should be truncated to 10 chars", "Very Long ", request.getCustomerName()); } @Test public void test_3_2() { RideRequest request = new RideRequest("John , Scarborough , 50.0 , Y "); request.setCustomerName(null); assertEquals("Set Customer Name method failed.", "", request.getCustomerName()); }
Please help, write the code for the test cases: public class JunitTest_RideRequestTest { // Test parameterized constructor with invalid input @Test public void test_2_0() { try { RideRequest request = new RideRequest(new String()); fail("You should throw an exception If the input is null or empty."); } catch (IllegalArgumentException e) { // exception excepted do nothing } } @Test public void test_2_2() { RideRequest request = null; try { RideRequest request2 = new RideRequest(request); fail("You should throw an exception If the input is null."); } catch (IllegalArgumentException e) { // exception excepted do nothing } } @Test public void test_2_3() { String s = "John , Downtown , 50.0 , Y , extra info"; try { RideRequest request2 = new RideRequest(s); fail("You should throw an exception If the input is not in correct format."); } catch (IllegalArgumentException e) { // exception excepted do nothing } } @Test public void test_2_4() { String s = null; try { RideRequest request2 = new RideRequest(s); fail("You should throw an exception If the input is null."); } catch (IllegalArgumentException e) { // exception excepted do nothing } } @Test public void test_2_5() { RideRequest request = new RideRequest(" Jack , North York , 9.99 , N "); request.setCustomerName("Bob"); request.setRideDetails("Airport"); request.setRidePrice(39.39); request.setHasDiscount(true); RideRequest copyRequest = new RideRequest(request); String errorMsg = String.format( "\n Test copy constructor failed. Returned CustomerName (%s) " + "but correct CustomerName is (%s)", copyRequest.getCustomerName(), "Bob"); assertEquals(errorMsg,"Bob", request.getCustomerName()); errorMsg = String.format( "\n Test copy constructor failed. Returned RideDetails (%s) " + "but correct RideDetails is (%s)", copyRequest.getRideDetails(), "Airport"); assertEquals(errorMsg, "Airport", copyRequest.getRideDetails()); assertEquals("Error: The constructor failed to set the ride price.",39.39, copyRequest.getRidePrice(), 0.0); assertTrue("Error: The constructor failed to set the ride discount.",copyRequest.isHasDiscount()); } @Test public void test_3_0() { RideRequest request = new RideRequest("John , Downtown , 50.0 , Y "); request.setCustomerName("Alice"); assertEquals("Set Customer Name method failed to update the customer name.", "Alice", request.getCustomerName()); } @Test public void test_3_1() { RideRequest request = new RideRequest("John , Scarborough , 50.0 , Y "); request.setCustomerName("Very Long Customer Name Exceeding Limit"); assertEquals("Set Customer Name method failed. Customer name should be truncated to 10 chars", "Very Long ", request.getCustomerName()); } @Test public void test_3_2() { RideRequest request = new RideRequest("John , Scarborough , 50.0 , Y "); request.setCustomerName(null); assertEquals("Set Customer Name method failed.", "", request.getCustomerName()); }
Chapter12: Exception Handling
Section: Chapter Questions
Problem 6RQ
Related questions
Question
Please help, write the code for the test cases:
public class JunitTest_RideRequestTest {
// Test parameterized constructor with invalid input
@Test
public void test_2_0() {
try {
RideRequest request = new RideRequest(new String());
fail("You should throw an exception If the input is null or empty.");
} catch (IllegalArgumentException e) {
// exception excepted do nothing
}
}
@Test
public void test_2_2() {
RideRequest request = null;
try {
RideRequest request2 = new RideRequest(request);
fail("You should throw an exception If the input is null.");
} catch (IllegalArgumentException e) {
// exception excepted do nothing
}
}
@Test
public void test_2_3() {
String s = "John , Downtown , 50.0 , Y , extra info";
try {
RideRequest request2 = new RideRequest(s);
fail("You should throw an exception If the input is not in correct format.");
} catch (IllegalArgumentException e) {
// exception excepted do nothing
}
}
@Test
public void test_2_4() {
String s = null;
try {
RideRequest request2 = new RideRequest(s);
fail("You should throw an exception If the input is null.");
} catch (IllegalArgumentException e) {
// exception excepted do nothing
}
}
@Test
public void test_2_5() {
RideRequest request = new RideRequest(" Jack , North York , 9.99 , N ");
request.setCustomerName("Bob");
request.setRideDetails("Airport");
request.setRidePrice(39.39);
request.setHasDiscount(true);
RideRequest copyRequest = new RideRequest(request);
String errorMsg = String.format(
"\n Test copy constructor failed. Returned CustomerName (%s) " + "but correct CustomerName is (%s)",
copyRequest.getCustomerName(), "Bob");
assertEquals(errorMsg,"Bob", request.getCustomerName());
errorMsg = String.format(
"\n Test copy constructor failed. Returned RideDetails (%s) " + "but correct RideDetails is (%s)",
copyRequest.getRideDetails(), "Airport");
assertEquals(errorMsg, "Airport", copyRequest.getRideDetails());
assertEquals("Error: The constructor failed to set the ride price.",39.39, copyRequest.getRidePrice(), 0.0);
assertTrue("Error: The constructor failed to set the ride discount.",copyRequest.isHasDiscount());
}
@Test
public void test_3_0() {
RideRequest request = new RideRequest("John , Downtown , 50.0 , Y ");
request.setCustomerName("Alice");
assertEquals("Set Customer Name method failed to update the customer name.", "Alice", request.getCustomerName());
}
@Test
public void test_3_1() {
RideRequest request = new RideRequest("John , Scarborough , 50.0 , Y ");
request.setCustomerName("Very Long Customer Name Exceeding Limit");
assertEquals("Set Customer Name method failed. Customer name should be truncated to 10 chars", "Very Long ", request.getCustomerName());
}
@Test
public void test_3_2() {
RideRequest request = new RideRequest("John , Scarborough , 50.0 , Y ");
request.setCustomerName(null);
assertEquals("Set Customer Name method failed.", "", request.getCustomerName());
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
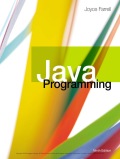
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
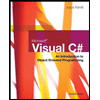
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
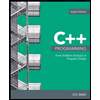
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
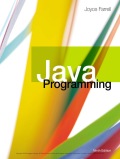
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
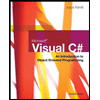
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
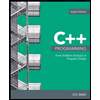
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
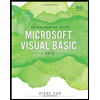
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
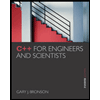
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr