Please help with the writing array program. Having hard time figuring out the code. Attatching the instruction, code outline and display result in the image.
Please help with the writing array program. Having hard time figuring out the code. Attatching the instruction, code outline and display result in the image.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Please help with the writing array program. Having hard time figuring out the code. Attatching the instruction, code outline and display result in the image.
![### Java Programming: Lab 7 Code and Output
#### Code Explanation and Assignment
In this lab assignment, you are required to create a Java program that consist of a main class named `Lab7`, and includes methods for handling user input and calculating statistical data.
```java
package homework;
public class Lab7 {
public static void main(String[] args) {
// Creating a new array using user input with the inputScores method
double[] scores = inputScores(?); // Enter any input array size for testing
// Displaying results
System.out.println("The number of scores above average is: " +
highScoreCount(scores));
}
public static double[] inputScores(int size) {
// Please use only one scanner object
Scanner input = new Scanner(System.in);
System.out.print("Please enter " + size + " scores in the array you want to make: \n");
// Please write your code here
}
public static int highScoreCount(double[] scores) {
// Please write your code here
}
}
```
The `inputScores` method should create an array based on user input, while the `highScoreCount` method is designed to calculate how many scores are above average in the input array.
#### Output Example
Your final code should produce an output similar to the following example in terms of structure and results:
```
Please enter 5 scores in the array you want to make:
Please enter a score
78
Please enter a score
54
Please enter a score
34
Please enter a score
87
Please enter a score
34
The number of scores above average is: 2
```
---
#### Steps to Follow
1. **Define Array Length in `main` Method**:
Specify the size of the array that the user will input scores into by replacing the question mark (`?`) in the `main` method call to `inputScores`.
2. **Implement `inputScores` Method**:
- Utilize a `Scanner` object to capture user input.
- Prompt the user to enter the scores based on the specified array size.
- Store these values in a double array.
3. **Implement `highScoreCount` Method**:
- Calculate the average score of the input array.
- Count how many scores are above the average.
- Return this count.
By following these instructions and properly implementing the methods](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34215fe4-737d-4111-858a-1ca99d565feb%2F3e49d1eb-fe11-463a-a644-a7256e0ebfbe%2F9i8bfgh_processed.png&w=3840&q=75)
Transcribed Image Text:### Java Programming: Lab 7 Code and Output
#### Code Explanation and Assignment
In this lab assignment, you are required to create a Java program that consist of a main class named `Lab7`, and includes methods for handling user input and calculating statistical data.
```java
package homework;
public class Lab7 {
public static void main(String[] args) {
// Creating a new array using user input with the inputScores method
double[] scores = inputScores(?); // Enter any input array size for testing
// Displaying results
System.out.println("The number of scores above average is: " +
highScoreCount(scores));
}
public static double[] inputScores(int size) {
// Please use only one scanner object
Scanner input = new Scanner(System.in);
System.out.print("Please enter " + size + " scores in the array you want to make: \n");
// Please write your code here
}
public static int highScoreCount(double[] scores) {
// Please write your code here
}
}
```
The `inputScores` method should create an array based on user input, while the `highScoreCount` method is designed to calculate how many scores are above average in the input array.
#### Output Example
Your final code should produce an output similar to the following example in terms of structure and results:
```
Please enter 5 scores in the array you want to make:
Please enter a score
78
Please enter a score
54
Please enter a score
34
Please enter a score
87
Please enter a score
34
The number of scores above average is: 2
```
---
#### Steps to Follow
1. **Define Array Length in `main` Method**:
Specify the size of the array that the user will input scores into by replacing the question mark (`?`) in the `main` method call to `inputScores`.
2. **Implement `inputScores` Method**:
- Utilize a `Scanner` object to capture user input.
- Prompt the user to enter the scores based on the specified array size.
- Store these values in a double array.
3. **Implement `highScoreCount` Method**:
- Calculate the average score of the input array.
- Count how many scores are above the average.
- Return this count.
By following these instructions and properly implementing the methods
![## Working with Arrays
### Objective
Write a program that reads a number of scores into an array and determines how many scores are above or equal to the average. Assume that the maximum number of scores is 100 and that each score is a double value.
### Instructions
1. **Input Method:**
Write a method that returns a double array using user's inputs from the console window. The method should have the following header. The formal parameter `size` is the size of the return array.
```java
public static double[] inputScores(int size)
```
Your input should be through the console.
2. **Processing Method:**
Write a method that returns the number of scores above or equal to the average.
```java
public static int highScoreCount(double[] scores)
```
3. **Main Method:**
Your program should contain the **"main"** method that invokes the `inputScores` and `highScoreCount` methods. The number of scores above or equal to the average should be displayed in the console window as the output of this program.
### Example
Here is a brief example explaining the workflow of the program:
1. **Reading Scores:**
- Call `inputScores(int size)` to read scores from the console. For example, the user inputs scores like `78.5, 82.0, 95.5, 88.0`.
2. **Calculating Above-Average Scores:**
- Call `highScoreCount(double[] scores)` with the array of scores obtained from `inputScores`.
3. **Displaying Output:**
- Print how many scores are above or equal to the average calculated.
By following the above steps, a well-structured program can be developed to manage and analyze scores using arrays efficiently.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34215fe4-737d-4111-858a-1ca99d565feb%2F3e49d1eb-fe11-463a-a644-a7256e0ebfbe%2Fcyxensd_processed.png&w=3840&q=75)
Transcribed Image Text:## Working with Arrays
### Objective
Write a program that reads a number of scores into an array and determines how many scores are above or equal to the average. Assume that the maximum number of scores is 100 and that each score is a double value.
### Instructions
1. **Input Method:**
Write a method that returns a double array using user's inputs from the console window. The method should have the following header. The formal parameter `size` is the size of the return array.
```java
public static double[] inputScores(int size)
```
Your input should be through the console.
2. **Processing Method:**
Write a method that returns the number of scores above or equal to the average.
```java
public static int highScoreCount(double[] scores)
```
3. **Main Method:**
Your program should contain the **"main"** method that invokes the `inputScores` and `highScoreCount` methods. The number of scores above or equal to the average should be displayed in the console window as the output of this program.
### Example
Here is a brief example explaining the workflow of the program:
1. **Reading Scores:**
- Call `inputScores(int size)` to read scores from the console. For example, the user inputs scores like `78.5, 82.0, 95.5, 88.0`.
2. **Calculating Above-Average Scores:**
- Call `highScoreCount(double[] scores)` with the array of scores obtained from `inputScores`.
3. **Displaying Output:**
- Print how many scores are above or equal to the average calculated.
By following the above steps, a well-structured program can be developed to manage and analyze scores using arrays efficiently.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
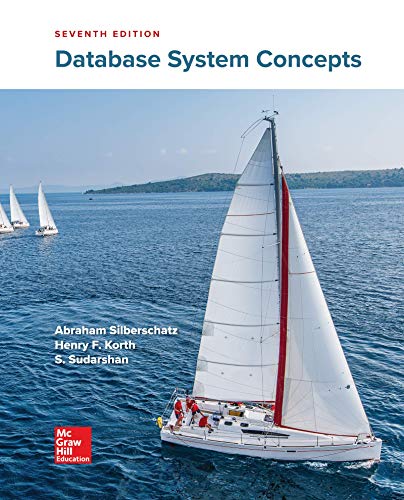
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
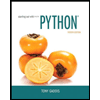
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
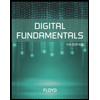
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
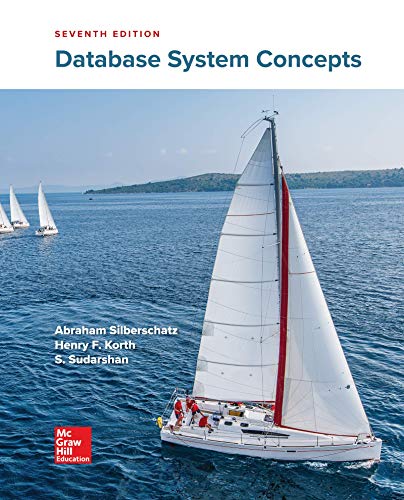
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
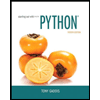
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
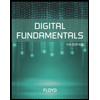
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
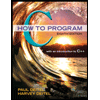
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
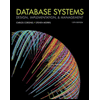
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
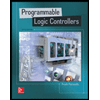
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education