please help with python code, i will need to open a file, but inside the text file has three columns the first column is english, second column morse code, third column is phoneticand i want to find the morse code inside the file, and make it morse dictionary, for example make it like this: {"A": ".-", "B": "-...", etc} or is there anyway that i can use dictionary to covert all three column into the dictionary then i can skip part above? heres my code, and the file that i need to open.
please help with python code, i will need to open a file, but inside the text file has three columns the first column is english, second column morse code, third column is phoneticand i want to find the morse code inside the file, and make it morse dictionary, for example make it like this: {"A": ".-", "B": "-...", etc} or is there anyway that i can use dictionary to covert all three column into the dictionary then i can skip part above? heres my code, and the file that i need to open.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
please help with python code, i will need to open a file, but inside the text file has three columns the first column is english, second column morse code, third column is phoneticand
i want to find the morse code inside the file, and make it morse dictionary, for example make it like this: {"A": ".-", "B": "-...", etc}
or is there anyway that i can use dictionary to covert all three column into the dictionary then i can skip part above? heres my code, and the file that i need to open.
![```python
def fonts(font):
#morse_dic = {}
with open(font, "r") as infile:
for line in infile:
morse = dict(line.findall(r'(\w)\d+([.-]+)'))
#(key, val) = line.split()
#new[str(key)] = val
#return morse
```
The image contains a Python function definition, `fonts`, which appears to be a work in progress for processing Morse code data from a file. Here’s a breakdown of the code's components:
1. **Function Definition**:
- `def fonts(font):` defines a function named `fonts` which takes one parameter `font`.
2. **Commented Dictionary**:
- `#morse_dic = {}` is a commented-out line indicating the potential initialization of an empty dictionary named `morse_dic`.
3. **Opening a File**:
- `with open(font, "r") as infile:` opens the file specified by `font` in read mode. `infile` is used as a file object.
4. **Reading Lines**:
- `for line in infile:` iterates over each line in the file.
5. **Regex and Dictionary Creation**:
- `morse = dict(line.findall(r'(\w)\d+([.-]+)'))`: This line appears to use a regular expression to find patterns in each line where a word character is followed by digits and a series of dots and dashes, then converts the result into a dictionary.
6. **Commented Code Lines**:
- `#(key, val) = line.split()` suggests an alternative approach for splitting each line into a key-value pair.
- `#new[str(key)] = val` likely indicates updating a dictionary with the key-value pair.
7. **Commented Return Statement**:
- `#return morse` hints at returning the `morse` dictionary but is currently commented out.
Overall, the script seems to be under development for parsing Morse code entries from a file, with various lines commented out for potential troubleshooting or future use.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F10732faa-f9b7-4c12-81f1-19b5d255f419%2F88bb725a-30ed-48ad-9810-023a5f806e60%2Fkwaaym9_processed.png&w=3840&q=75)
Transcribed Image Text:```python
def fonts(font):
#morse_dic = {}
with open(font, "r") as infile:
for line in infile:
morse = dict(line.findall(r'(\w)\d+([.-]+)'))
#(key, val) = line.split()
#new[str(key)] = val
#return morse
```
The image contains a Python function definition, `fonts`, which appears to be a work in progress for processing Morse code data from a file. Here’s a breakdown of the code's components:
1. **Function Definition**:
- `def fonts(font):` defines a function named `fonts` which takes one parameter `font`.
2. **Commented Dictionary**:
- `#morse_dic = {}` is a commented-out line indicating the potential initialization of an empty dictionary named `morse_dic`.
3. **Opening a File**:
- `with open(font, "r") as infile:` opens the file specified by `font` in read mode. `infile` is used as a file object.
4. **Reading Lines**:
- `for line in infile:` iterates over each line in the file.
5. **Regex and Dictionary Creation**:
- `morse = dict(line.findall(r'(\w)\d+([.-]+)'))`: This line appears to use a regular expression to find patterns in each line where a word character is followed by digits and a series of dots and dashes, then converts the result into a dictionary.
6. **Commented Code Lines**:
- `#(key, val) = line.split()` suggests an alternative approach for splitting each line into a key-value pair.
- `#new[str(key)] = val` likely indicates updating a dictionary with the key-value pair.
7. **Commented Return Statement**:
- `#return morse` hints at returning the `morse` dictionary but is currently commented out.
Overall, the script seems to be under development for parsing Morse code entries from a file, with various lines commented out for potential troubleshooting or future use.

Transcribed Image Text:**NATO Phonetic Alphabet and Morse Code Chart**
The chart displays the NATO phonetic alphabet alongside their Morse code representations. Each letter and number is associated with a specific word from the NATO phonetic alphabet used to communicate clearly over radio and telephone, especially in noisy conditions. Below is the transcribed list from the image:
- **A**: .- ALPHA
- **B**: -... BRAVO
- **C**: -.-. CHARLIE
- **D**: -.. DELTA
- **E**: . ECHO
- **F**: ..-. FOXTROT
- **G**: --. GOLF
- **H**: .... HOTEL
- **I**: .. INDIA
- **J**: .--- JULIETT
- **K**: -.- KILO
- **L**: .-.. LIMA
- **M**: -- MIKE
- **N**: -. NOVEMBER
- **O**: --- OSCAR
- **P**: .--. PAPA
- **Q**: --.- QUEBEC
- **R**: .-. ROMEO
- **S**: ... SIERRA
- **T**: - TANGO
- **U**: ..- UNIFORM
- **V**: ...- VICTOR
- **W**: .-- WHISKEY
- **X**: -..- XRAY
- **Y**: -.-- YANKEE
- **Z**: --.. ZULU
**Numbers:**
- **0**: ----- ZEE-ROW
- **1**: .---- WUN
- **2**: ..--- TOO
- **3**: ...-- TREE
- **4**: ....- FOW-ER
- **5**: ..... FIFE
- **6**: -.... SIX
- **7**: --... SEV-VEN
- **8**: ---.. ATE
- **9**: ----. NIN-ER
- **.**: .-.-.- STOP
- **!**: -.-.-- BANG
This chart is essential for learning both Morse code and the phonetic alphabet, which are widely used in military, aviation, and radio communications for clarity and accuracy.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
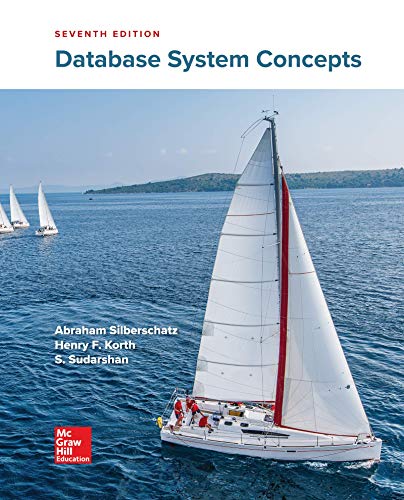
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
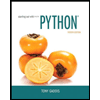
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
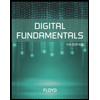
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
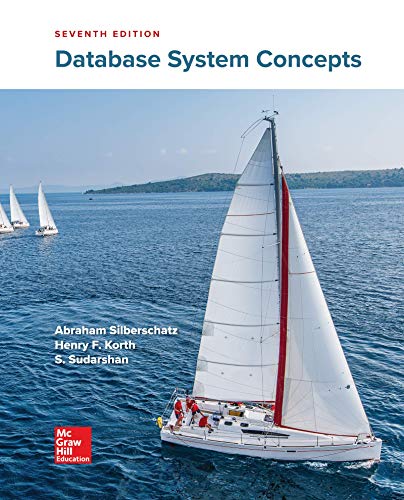
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
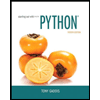
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
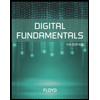
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
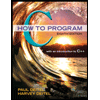
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
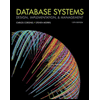
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
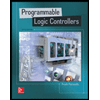
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education