Please help! There is a bug in my program that causes it to jump to the notfound function before checking the entire substring The program should determine whether the second string is a substring of the first. If it is, then the program should print out the first index in which the second string appears in the first. As an example, if the first string is “Hello World” and the second string is “lo”, then the program should print out 3, i.e. the starting index of “lo” in “Hello World.” If the second string is not contained in the first string, then the program should print out -1. Here is the code: .datastr1: .space 51 #space for str1 (max50 characters including null terminator)str2: .space 51 #space for str2 (max50 characters incl null terminator)msg1: .asciiz "Please enter the first string: " #prompt user to enter str1msg2: .asciiz "Please enter the second string: " #prompt user to enter str2newline: .asciiz "\n" .text.globl mainmain: # Print sttr1 li $v0, 4 la $a0, msg1 syscall # Read string 1 li $v0, 8 la $a0, str1 li $a1, 51 syscall # Print newline li $v0, 4 la $a0, newline syscall # Print message 2 li $v0, 4 la $a0, msg2 syscall # Read string 2 li $v0, 8 la $a0, str2 li $a1, 51 syscall #Initialize the counters & pointers la $t0, str1 # Register for str1 la $t1, str2 # Register for str2 li $t3, 0 # Index counter for str1 move $t2, $t0 # Pointer to keep track of starting position in the first string substringfinder: lb $t4, 0($t0) # Load character from the first string beqz $t4, notfound # If it reaches end of str1 with no match, not found lb $t5, 0($t1) # Load character from the second string beqz $t5, notfound #If it reaches end of str2 with no match, not found # Check if current characters match beq $t4, $t5, checksubstring # Check next character in str1 addiu $t0, $t0, 1 addiu $t3, $t3, 1 #Increase index counter by 1 move $t2, $t0 # Update starting position j substringfinder # Check next character checksubstring: addiu $t0, $t0, 1 #Move to next character in the first string addiu $t1, $t1, 1 #Move to next character in the second string lb $t4, 0($t0) #Load next character from the str1 lb $t5, 0($t1) #Load next character from the str2 beqz $t5, found # If end of str2, substring found bne $t4, $t5, reset # If characters do not match, reset pointers j checksubstring #keep checking reset: move $t0, $t2 # Reset str1 pointer to initial position la $t1, str2 # Reset str2 pointer addiu $t2, $t2, 1 # Move start position in str1 by 1 j substringfinder # Keep searching found: li $v0, 1 # display integer move $a0, $t3 # Load index of substring into $a0 syscall j exit # Exit the program notfound: li $v0, 1 # Display integer li $a0, -1 # Load -1 to indicate not found syscall exit: li $v0, 10 # Exit syscall
Please help! There is a bug in my
The program should determine whether the second string is a substring of the first.
If it is, then the program should print out the first index in which the second string appears in the first.
As an example, if the first string is “Hello World” and the second string is “lo”, then the program should print out 3, i.e. the starting index of “lo” in “Hello World.”
If the second string is not contained in the first string, then the program should print out -1.
Here is the code:
.data
str1: .space 51 #space for str1 (max50 characters including null terminator)
str2: .space 51 #space for str2 (max50 characters incl null terminator)
msg1: .asciiz "Please enter the first string: " #prompt user to enter str1
msg2: .asciiz "Please enter the second string: " #prompt user to enter str2
newline: .asciiz "\n"
.text
.globl main
main:
# Print sttr1
li $v0, 4
la $a0, msg1
syscall
# Read string 1
li $v0, 8
la $a0, str1
li $a1, 51
syscall
# Print newline
li $v0, 4
la $a0, newline
syscall
# Print message 2
li $v0, 4
la $a0, msg2
syscall
# Read string 2
li $v0, 8
la $a0, str2
li $a1, 51
syscall
#Initialize the counters & pointers
la $t0, str1 # Register for str1
la $t1, str2 # Register for str2
li $t3, 0 # Index counter for str1
move $t2, $t0 # Pointer to keep track of starting position in the first string
substringfinder:
lb $t4, 0($t0) # Load character from the first string
beqz $t4, notfound # If it reaches end of str1 with no match, not found
lb $t5, 0($t1) # Load character from the second string
beqz $t5, notfound #If it reaches end of str2 with no match, not found
# Check if current characters match
beq $t4, $t5, checksubstring
# Check next character in str1
addiu $t0, $t0, 1
addiu $t3, $t3, 1 #Increase index counter by 1
move $t2, $t0 # Update starting position
j substringfinder # Check next character
checksubstring:
addiu $t0, $t0, 1 #Move to next character in the first string
addiu $t1, $t1, 1 #Move to next character in the second string
lb $t4, 0($t0) #Load next character from the str1
lb $t5, 0($t1) #Load next character from the str2
beqz $t5, found # If end of str2, substring found
bne $t4, $t5, reset # If characters do not match, reset pointers
j checksubstring #keep checking
reset:
move $t0, $t2 # Reset str1 pointer to initial position
la $t1, str2 # Reset str2 pointer
addiu $t2, $t2, 1 # Move start position in str1 by 1
j substringfinder # Keep searching
found:
li $v0, 1 # display integer
move $a0, $t3 # Load index of substring into $a0
syscall
j exit # Exit the program
notfound:
li $v0, 1 # Display integer
li $a0, -1 # Load -1 to indicate not found
syscall
exit:
li $v0, 10 # Exit
syscall

Step by step
Solved in 2 steps

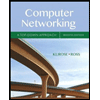
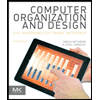
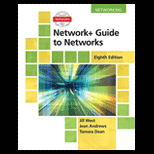
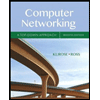
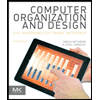
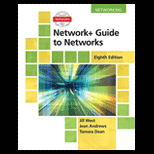
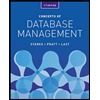
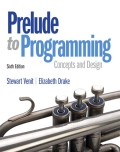
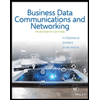