Please help review my code, not sure its working as intended.... its in C! Objective Give practice with Recursion in C. Give practice with Backtracking in C. Give practice with Stacks in C. Input will begin with a line containing 2 integers, n and c (1 ≤ n ≤ 20; 0 ≤ c ≤ n choose 2), representing the number of animal exhibits and the number of constraints. The following c lines will each contain a constraint description in the form of 3 space separated integers, f, s, and d
Please help review my code, not sure its working as intended.... its in C!
Objective
Give practice with Recursion in C.
Give practice with Backtracking in C.
Give practice with Stacks in C.
Input will begin with a line containing 2 integers, n and c (1 ≤ n ≤ 20; 0 ≤ c ≤ n choose 2),
representing the number of animal exhibits and the number of constraints. The following c lines
will each contain a constraint description in the form of 3 space separated integers, f, s, and d
(1 ≤ f, s ≤ n; f ≠ s; 0 ≤ c ≤ n - 2), representing the index of the first animal, the index of the
second animal, and the exact number of cages that should be in between those 2 animals.
For 7 of the 10 cases each animal will be in at most 1 constraint.
Output should contain 1 line. In the event that a solution exists, you should print out n space
separated integers representing the animal index given in order from the first cage to the last. If
there is no solution the output should be only the phrase “No Solution.” (quotes for clarity).
Sample Input | Sample output |
4 2 1 2 1 3 4 0 |
No Solution |
Sample Input | Sample Output |
5 4 1 2 1 1 3 1 1 5 0 2 5 2 |
2 4 1 5 3 |
Code below:

Step by step
Solved in 3 steps with 1 images

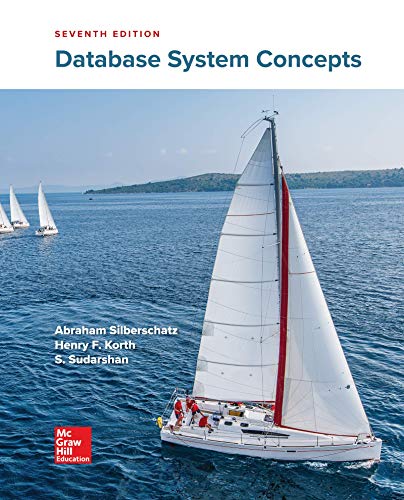
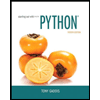
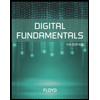
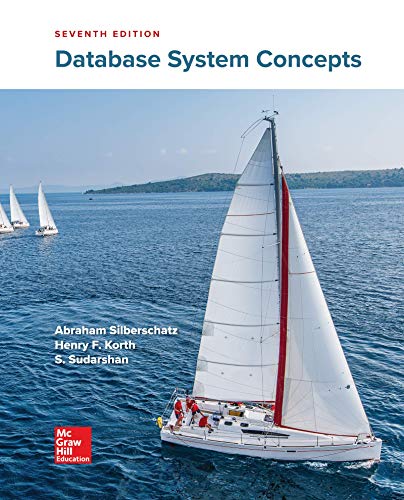
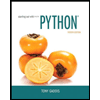
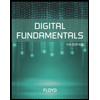
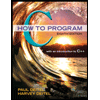
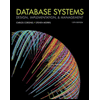
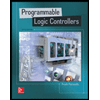