Please help me with this! Develop a high-quality, menu-driven object-oriented C++ program that creates a small database, using a binary search tree structure to store and process the data. The database will contain the top 100 highest grossing films of 2017. The C++ object-oriented program must use the BinarySearchTree class . When designing and implementing the program, apply good software engineering principles. Start the analysis and design process by drawing a complete UML class diagram for the program that includes all the classes that are contained in the program, including the classes provided by the textbook and the classes that you create. The UML class diagram will assist you in understanding the components of the project. Your program must include: a Film class that stores all the data for a Film and provides appropriate methods to support good software engineering principles, a FilmDatabase class that stores the binary search tree and provides appropriate methods in support of database queries and reporting using good software engineering principles, an application that interacts with the end-user. The design is up to you and may include any number of classes in support of the given application. A Menu class is strongly recommended. Note that the binary search tree must store Film objects, and the >, <, and == operators must be defined for that class because the BinarySearchTree class uses those overloaded operators. Data Details: The database contains data pertaining to the 100 highest grossing films of 2017. A comma delimited file named Films2017.csv contains the initial data. Each record is stored on one line of the file in the following format: Data Data type Rank int Film Title (key) string Studio string Total Gross double Total Theaters int Opening Gross double Opening Theaters int Opening Date string Each of the data fields is separated in the file using the comma (,) character as a delimiter. There is no comma (,) character after the last field on the line. The data is considered clean; There are no errors in the input file. When storing the data in the binary search tree, use the data types shown above. The Film Title will serve as the key field. Therefore, an inorder traversal of the BST will produce the Films in order of title. Menu Details: Your application must be menu driven. The menu system consists of a main menu and sub-menus. All menu choices are selected by entering the letter of the desired choice. After a selection is processed, the current me
Please help me with this!
Develop a high-quality, menu-driven object-oriented C++ program that creates a small
The C++ object-oriented program must use the BinarySearchTree class . When designing and implementing the program, apply good software engineering principles. Start the analysis and design process by drawing a complete UML class diagram for the program that includes all the classes that are contained in the program, including the classes provided by the textbook and the classes that you create. The UML class diagram will assist you in understanding the components of the project.
Your program must include:
- a Film class that stores all the data for a Film and provides appropriate methods to support good software engineering principles,
- a FilmDatabase class that stores the binary search tree and provides appropriate methods in support of database queries and reporting using good software engineering principles,
- an application that interacts with the end-user. The design is up to you and may include any number of classes in support of the given application. A Menu class is strongly recommended.
Note that the binary search tree must store Film objects, and the >, <, and == operators must be defined for that class because the BinarySearchTree class uses those overloaded operators.
Data Details:
The database contains data pertaining to the 100 highest grossing films of 2017. A comma delimited file named Films2017.csv contains the initial data. Each record is stored on one line of the file in the following format:
Data | Data type |
Rank | int |
Film Title (key) | string |
Studio | string |
Total Gross | double |
Total Theaters | int |
Opening Gross | double |
Opening Theaters | int |
Opening Date | string |
Each of the data fields is separated in the file using the comma (,) character as a delimiter. There is no comma (,) character after the last field on the line. The data is considered clean; There are no errors in the input file.
When storing the data in the binary search tree, use the data types shown above. The Film Title will serve as the key field. Therefore, an inorder traversal of the BST will produce the Films in order of title.
Menu Details:
Your application must be menu driven. The menu system consists of a main menu and sub-menus. All menu choices are selected by entering the letter of the desired choice. After a selection is processed, the current menu should be re-displayed. Do NOT use recursion to do this; use a loop. The current menu continues until the X option (return to main menu or exit) is selected.
When the application begins, the following main menu should be displayed:
MAIN MENU
A - About the Application
R - Reports
S - Search the Database
X - Exit the Program
Enter Selection ->
A - About the Application
If the end-user chooses About the Application, the program provides a detailed description for the user, explaining what the application is doing and how it works. Note that this method does NOT substitute for javadoc-style comments. The audience for this method consists of non-technical users that have no information at all about the assignment.
R - Reports
If the end-user chooses Reports from the MAIN MENU, the program displays the following sub-menu:
REPORTS MENU
T - Order by Film Title report
R - Order by Rank report
X - Return to main menu
Enter Selection ->



Trending now
This is a popular solution!
Step by step
Solved in 8 steps with 6 images

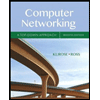
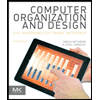
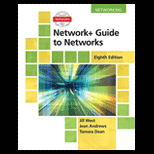
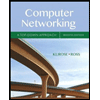
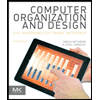
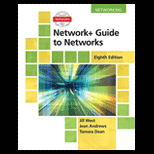
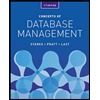
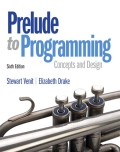
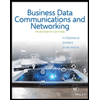