Please help me create a web application and show examples of images and links.
Please help me create a web application and show examples of images and links.
app.js
var express = require('express');
var path = require('path');
var indexRouter = require('./routes/index');
var app = express();
app.use('/', indexRouter);
const PORT = process.env.PORT || 3050
app.listen(PORT,()=> console.info(`Server has started on ${PORT}`))
module.exports = app;
index.js
var express = require('express');
var router = express.Router();
const path = require("path");
router.get('/', function(req, res, next) {
res.sendFile(path.resolve('public/index.html') );
});
router.get('/*', function(req, res, next) {
res.sendFile(path.resolve('public/' + req.url) );
});
you will create four static web pages.
The main page (index.html) will contain three links to open the three pages defined below.
The three content pages will contain a name, a description and a picture of a character of your choosing
The main page
The main page must contain three links, one to each of the content pages.
Each of the three links must use <a> elements.
The main page does not need any particular styling.
The three content pages
The content pages should include characters from a book, video, show, movie, etc.
This is up to you.
Each content page must include the following:
A name using the <h1> element.
A description using the <p> element.
The description should be short. Somewhere between 1 - 5 sentences of data.
An image using the <img> element
Each image should be the same size, no bigger than 300x300 pixels.
A link to the next content page using the <a> element (5 pts)
page 1 will link to page 2.
page 2 will link to page 3.
page 3 will link to page 1
A link to the main page
The link must use the <a> element.
The content pages do not need any particular styling.
The site MUST use the node.js server.

Step by step
Solved in 3 steps

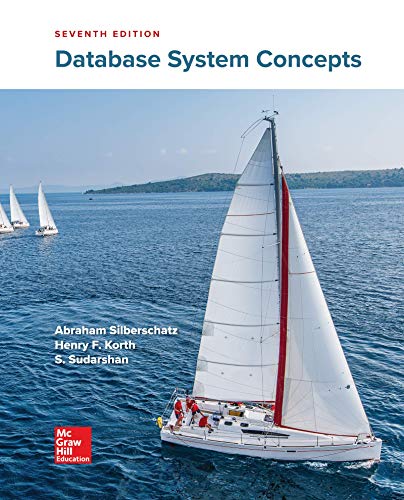
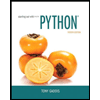
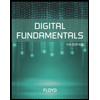
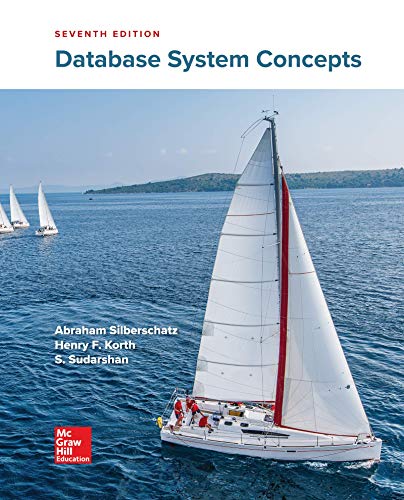
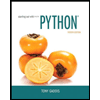
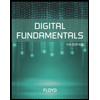
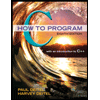
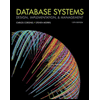
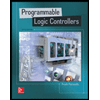