Please finish this problem in OCaml. Please implement two functions: string_explode : string -> char list string_implode : char list -> string string_explode turns a string into a list of characters and string_implode turns a list of characters back into a string. To implement these two functions, use a selection of the following higher-order functions: List.map, List.fold_right, List.fold_left and tabulate. tabulate is implemented for you in the prelude. You may also find the following functions from the OCaml string and char libraries useful: String.get : string -> int -> char returns the character at index n in string s. String.length : string -> int returns the length (number of characters) of the given string. Char.escaped : char -> string returns the string representation of the given character In order to get full marks for each question, you must use higher-order functions. Solutions using manual recursion will be capped at half marks. Please follow the follwing format: let string_explode (s : string) : char list = raise NotImplemented let string_implode (l : char list) : string = raise NotImplemented tabulate is defined as follow: let rec tabulate f n = let rec tab n acc = if n < 0 then acc else tab (n - 1) ((f n) :: acc) in tab (n - 1) []
Please finish this problem in OCaml.
Please implement two functions:
string_explode : string -> char list
string_implode : char list -> string
string_explode turns a string into a list of characters and string_implode turns a list of characters back into a string.
To implement these two functions, use a selection of the following higher-order functions: List.map, List.fold_right, List.fold_left and tabulate. tabulate is implemented for you in the prelude.
You may also find the following functions from the OCaml string and char libraries useful:
String.get : string -> int -> char returns the character at index n in string s.
String.length : string -> int returns the length (number of characters) of the given string.
Char.escaped : char -> string returns the string representation of the given character
In order to get full marks for each question, you must use higher-order functions. Solutions using manual recursion will be capped at half marks.
Please follow the follwing format:
let string_explode (s : string) : char list =
raise NotImplemented
let string_implode (l : char list) : string =
raise NotImplemented
tabulate is defined as follow:
let rec tabulate f n =
let rec tab n acc =
if n < 0 then acc
else tab (n - 1) ((f n) :: acc)
in
tab (n - 1) []

Step by step
Solved in 3 steps

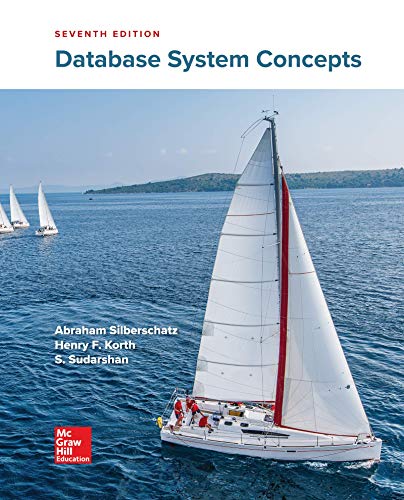
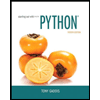
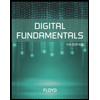
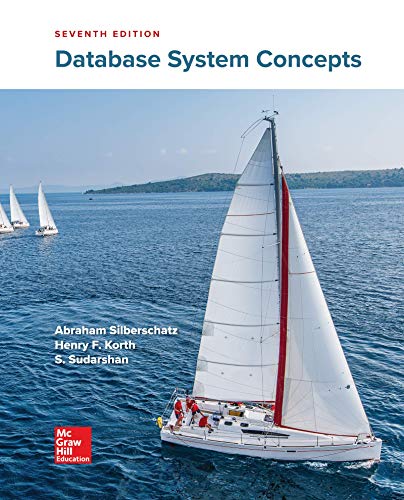
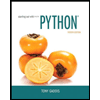
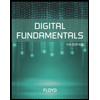
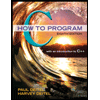
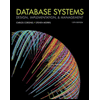
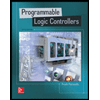