please do in java firstname_lastname_assignment_6.zip ├── library │ └── docs │ └── StudentGrades.java Folder Structure Your project will contain three sub-directories: library test In the library directory, create the StudentGrades class. In the test directory, create the test application (Part 1). StudentGrades Class Write a class encapsulating the concept of Student Grades. Use the class diagram above as well as the specific requirements below to develop your class: Class Members The attribute grades will store grades for students. StudentGrades(int) - Initializes grades to a new array with the specified number of elements. The grade values are zero. StudentGrades(int[]) - Initializes grades to the specified array. Ensure that the reference to the grades is not accessible outside of the class. copyArray(int[]) : int[] - Returns a copy of the specified array. getGrades() : int[] - Returns a copy of the grades. setGrades(int[]) : void - Sets the grades. Ensure that the reference to the grades is not accessible outside of the class. getSortedGrades() : int[] - Returns a new array containing the values of the grades attribute sorted in ascending order. getHighestGrade() : int - Returns the value of the highest grade in the grades. getAverageOfGrades() : double - Returns the mean average of the values in the grades array. Formula: (grades[0] + grades[1] + …. grades[n]) / number of elements in array The toString will return a String representation of the class in the following format: toString Format =================== Student Grade =================== 1 {first element value} 2 {second element value} ... n {nth element value} Example =================== Student Grade =================== 1 79 2 54 ... n 82
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
please do in java
firstname_lastname_assignment_6.zip
├── library
│ └── docs
│ └── StudentGrades.java
Folder Structure
Your project will contain three sub-directories:
- library
- test
In the library directory, create the StudentGrades class. In the test directory, create the test application (Part 1).
StudentGrades Class
Write a class encapsulating the concept of Student Grades. Use the class diagram above as well as the specific requirements below to develop your class:
Class Members
- The attribute grades will store grades for students.
- StudentGrades(int) - Initializes grades to a new array with the specified number of elements. The grade values are zero.
- StudentGrades(int[]) - Initializes grades to the specified array. Ensure that the reference to the grades is not accessible outside of the class.
- copyArray(int[]) : int[] - Returns a copy of the specified array.
- getGrades() : int[] - Returns a copy of the grades.
- setGrades(int[]) : void - Sets the grades. Ensure that the reference to the grades is not accessible outside of the class.
- getSortedGrades() : int[] - Returns a new array containing the values of the grades attribute sorted in ascending order.
- getHighestGrade() : int - Returns the value of the highest grade in the grades.
- getAverageOfGrades() : double - Returns the mean average of the values in the grades array.
- Formula: (grades[0] + grades[1] + …. grades[n]) / number of elements in array
- The toString will return a String representation of the class in the following format:
toString Format
Example
![Class Diagram
Student Grades
-grades: int[]
+StudentGrades (numberOfStudents : int)
+StudentGrades (grades: int[])
-copyArray(toCopy: int[]): int[]
+getGrades() int[]
+ setGrades (grades int[]): void
+getSorted Grades(): int[]
+getHighestGrade(): int
+getAverageOfGrades(): double
+toString(): String](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa4bd34a9-cad0-4bb6-9739-21da21649a7b%2F62216c9f-2d47-4c7c-8f2c-3ce854d094c7%2Fediy2iw_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

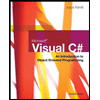
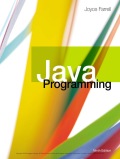
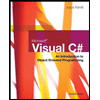
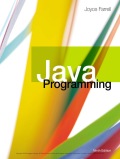