please answer this question using python 3. Submit function: reads the five inputs, calls the functions listed below as needed, and displays tax. Inputs # of jobs: income : # of children: Is Married?: Is Student? : Outputs: tax functions Required 1. count_regular_exemptions() Calculates number of regular exemptions. Everyone starts with one exemption If no jobs, you get one more exemption If you have one job: You get one more exemption if you are married, or if you are a single student. If you have more than one job: You get one more exemption if your income is less than 1500. 2. count_child_exemptions() Calculates number of child credit exemptions. If your income is less than $70,000 : You get 4 times the number of children as the number of exemptions. Example: income 60,000 with 3 children: 4 * 3 = 12 exemptions If your income is at or above $70,000 : You get one additional exemption for every 2 kids. That is: 0 exemptions for 1 child, 1 for 2 children, 1 for 3, 2 for 4 and 5 children, 3 for 6 and 7 children, etc. 3. compute_taxable_income() Calculates the taxable income. Each child exemption is worth $1000. Each regular exemption is worth $2000. exemption amount is the sum of child exemption amount and regular exemption amount, each computed using the counts and unit exemptions above. Taxable income = Income - Exemption amount 4. compute_tax_rate Determine tax rate based on taxable income. Find the tax rate based on the table below. Rate Single Married 10% Up to,including, 38,000 Up to and including $78,000 15% Above 38,000 Above 78,000 tax is determined using taxable income and tax rate. 5. compute_deduction Calculate deduction amount to be subtracted from tax. Deduction amount starts at 0 dollars. if student and income < $80,000: $2000 if student and income < $57,000: $3000 The income referred to above is the ‘income’ input by the user. Not taxable income. tax is updated by subtracting any deduction amount from tax. If tax < 0: set tax due to $0.00. Output format: Your estimated taxes due are: $XXXX Summary: Show average tax for students, and the number of taxpayers. Use a function to compute the average. Reset: Getting ready for a new series of inputs. Additional features: revisit and optimize the if statements to be as efficient as possible Include counts of: married students, single students, and non-student taxpayers in summary #main quit = False while not quit: print('1.Submit 2.Summary 3.Reset 4.Exit') choice = int(input('Enter choice: ')) if choice == 1: submit() elif choice == 2: summary() elif choice == 3: reset() elif choice == 4: quit = True else: print('Invalid Choice!')
please answer this question using python 3.
Submit function:
reads the five inputs, calls the functions listed below as needed, and displays tax.
Inputs
# of jobs:
income :
# of children:
Is Married?:
Is Student? :
Outputs: tax
functions Required
1. count_regular_exemptions()
Calculates number of regular exemptions.
- Everyone starts with one exemption
- If no jobs, you get one more exemption
- If you have one job:
You get one more exemption if you are married, or if you are a single student.
- If you have more than one job:
You get one more exemption if your income is less than 1500.
2. count_child_exemptions()
Calculates number of child credit exemptions.
- If your income is less than $70,000 : You get 4 times the number of children as the number of exemptions. Example: income 60,000 with 3 children: 4 * 3 = 12 exemptions
- If your income is at or above $70,000 : You get one additional exemption for every 2 kids. That is: 0 exemptions for 1 child, 1 for 2 children, 1 for 3, 2 for 4 and 5 children, 3 for 6 and 7 children, etc.
3. compute_taxable_income()
Calculates the taxable income.
Each child exemption is worth $1000.
Each regular exemption is worth $2000.
exemption amount is the sum of child exemption amount and regular exemption amount, each computed using the counts and unit exemptions above.
Taxable income = Income - Exemption amount
4. compute_tax_rate
Determine tax rate based on taxable income.
Find the tax rate based on the table below.
Rate |
Single |
Married |
10% |
Up to,including, 38,000 |
Up to and including $78,000 |
15% |
Above 38,000 |
Above 78,000 |
tax is determined using taxable income and tax rate.
5. compute_deduction
Calculate deduction amount to be subtracted from tax.
Deduction amount starts at 0 dollars.
- if student and income < $80,000: $2000
- if student and income < $57,000: $3000
The income referred to above is the ‘income’ input by the user. Not taxable income.
tax is updated by subtracting any deduction amount from tax.
If tax < 0: set tax due to $0.00.
Output format: Your estimated taxes due are: $XXXX
Summary:
Show average tax for students, and the number of taxpayers. Use a function to compute the average.
Reset: Getting ready for a new series of inputs.
Additional features:
- revisit and optimize the if statements to be as efficient as possible
- Include counts of: married students, single students, and non-student taxpayers in summary
#main
quit = False
while not quit:
print('1.Submit 2.Summary 3.Reset 4.Exit')
choice = int(input('Enter choice: '))
if choice == 1:
submit()
elif choice == 2:
summary()
elif choice == 3:
reset()
elif choice == 4:
quit = True
else:
print('Invalid Choice!')

- Initialize the following variables:
- num_married_students = 0
- num_single_students = 0
- num_non_student_taxpayers = 0
- total_tax_students = 0
- total_tax_taxpayers = 0
- Define a function count_regular_exemptions that takes num_jobs, income, is_married, and is_student as input. a. Set exemptions to 1. b. If num_jobs is 0, increment exemptions by 1. c. If num_jobs is 1:
- If is_married or is_student is true, increment exemptions by 1.
- If income is less than 1500, increment exemptions by 1. d. If num_jobs is greater than 1 and income is less than 1500, increment exemptions by 1. e. Return the calculated exemptions.
- Define a function count_child_exemptions that takes income and num_children as input. a. If income is less than 70000, return 4 times the number of children. b. Otherwise, return the integer division of num_children by 2.
- Define a function compute_taxable_income that takes income and exemptions as input. a. Calculate the child_exemption_amount as 1000 times the child exemptions. b. Calculate the regular_exemption_amount as 2000 times the regular exemptions. c. Calculate the total_exemption_amount as the sum of child_exemption_amount and regular_exemption_amount. d. Return the difference between income and total_exemption_amount as the taxable income.
- Define a function compute_tax_rate that takes taxable_income and is_married as input. a. If is_married is true and taxable_income is less than or equal to 78000, return 0.10. b. If is_married is true and taxable_income is greater than 78000, return 0.15. c. If is_married is false and taxable_income is less than or equal to 38000, return 0.10. d. If is_married is false and taxable_income is greater than 38000, return 0.15.
- Define a function compute_deduction that takes is_student and income as input. a. Set deduction_amount to 0. b. If is_student is true and income is less than 80000, set deduction_amount to 2000. c. If is_student is true, income is less than 57000, and deduction_amount is already set to 2000, update deduction_amount to 3000. d. Return the deduction_amount.
- Define a function submit that takes no input. a. Within submit: i. Prompt the user for input: num_jobs, income, num_children, is_married, and is_student. ii. Calculate exemptions using count_regular_exemptions and count_child_exemptions functions. iii. Calculate taxable_income using compute_taxable_income function. iv. Calculate tax_rate using compute_tax_rate function. v. Calculate tax as taxable_income multiplied by tax_rate. vi. Calculate deduction using compute_deduction function. vii. Subtract deduction from tax and ensure it is non-negative. viii. Display the estimated taxes due. ix. Update counts and total tax for the summary.
- Define a function summary that takes no input. a. Within summary: i. Calculate the average tax for students and display it. ii. Display the number of taxpayers - married students, single students, and non-student taxpayers, and the total count.
- Define a function reset that takes no input. a. Within reset: i. Reset all count variables to 0.
Implement the main program using a while loop: a. Display a menu with options for submission, summary, reset, and exit. b. Prompt the user for a choice. c. Based on the user's choice, call the appropriate function: submit, summary, reset, or exit the program. d. Handle invalid choices by displaying an error message.
End the algorithm.
Step by step
Solved in 3 steps with 1 images

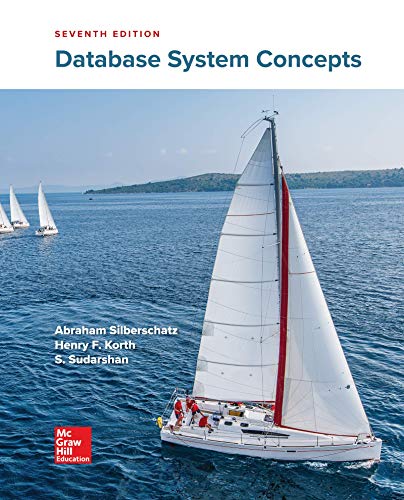
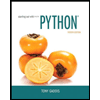
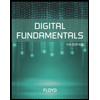
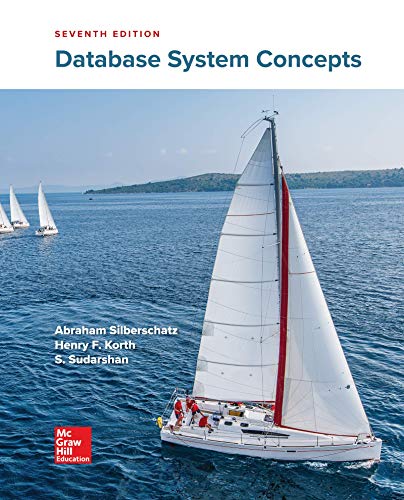
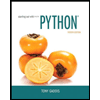
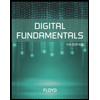
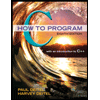
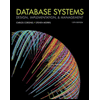
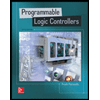