Part 3: Factorial function In computer science, and other fields, we frequently need to know how many possible combinations are possible given a specific number of numbers or characters. For example, consider three color cards: red (R), green (G), and blue (B). How many different orders are possible of these three cards? RGB, RBG, GRB, GBR, BRG, BGR = six (6) possible combinations The mathematical term for this calculation is factorial (see Factorial Function !Links to an external site. web page) and is expressed using the exclamation point (!). Surprisingly, the JavaScript Math object does not have a function for factorial, so we will write our own.
Part 3: Factorial function
In computer science, and other fields, we frequently need to know how many possible combinations are possible given a specific number of numbers or characters. For example, consider three color cards: red (R), green (G), and blue (B). How many different orders are possible of these three cards?
RGB, RBG, GRB, GBR, BRG, BGR = six (6) possible combinations
The mathematical term for this calculation is factorial (see Factorial Function !Links to an external site. web page) and is expressed using the exclamation point (!). Surprisingly, the JavaScript Math object does not have a function for factorial, so we will write our own.
So how do we calculate factorial? The steps to accomplish a task are known as an
3! = 1 * 2 * 3 = 6
Factorials only work on values >= 0, with a special rule for 0! = 1.
Below are the factorial rules you will need to implement.
Input | Result |
Input > 0 | Calculate and return factorial |
Input = 0 | Return 1 by rule |
Input < 0 | Return -1 to indicate error |
Non-numeric input | Return -1 to indicate error |
To create a factorial function, we will give you the algorithm as comment steps, and you will provide the code.
function factorial (num) { // Step 1: Set -1 as a default result for values < 0 (invalid input) // Step 2: Test if input is > 0, calculate factorial and set result to calculated value // Step 3: Test if input = 0, set result to 1 // Return result }
>> Use the above code outline steps and rules to write the factorial function. Your function must use a for loop and if conditional syntax.
Tip: Yes, you can find solutions to this function online, but please try to solve this problem on your own. Your programming "muscles" will only improve through programming "exercise," and using online solutions is not only against the integrity policy of this course and the University, but will only make solving later problems much more difficult where no online solution exists.

Step by step
Solved in 4 steps with 2 images

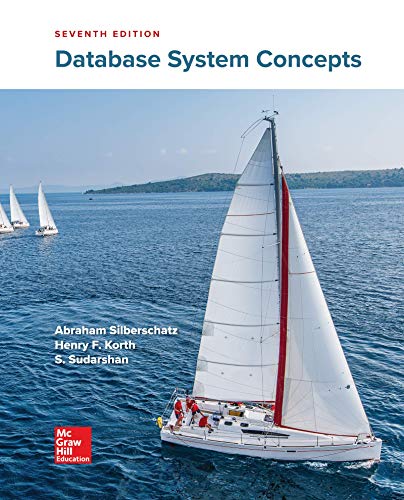
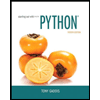
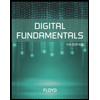
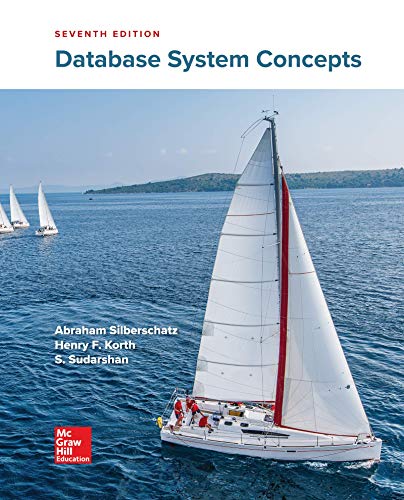
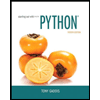
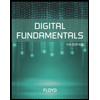
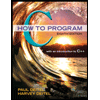
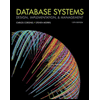
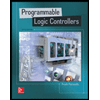