PART 1 1. Using python, create a program with a function of enc_text(text, key) to encrypt the variable text using the provided key (executing a modified version of Ceasar Cipher). Follow these rules: a. The key will contain a word made up of the letters 'a' and 'b'. The cipher will use the key to know the direction of the shift: • 'a' - the character must be shifted upwards • 'b' - the character is to be shifted downward. b. The number of characters in the key will determine the number of positions to shift. c. The given text variable will only have characters from the ASCII table (values between 32 and 126). d. If the key is invalid (contains characters other than a and b or an empty string) or the text is invalid (empty string), no encryption will happen and the function returns the original text. e. The key will loop through again to accommodate the additional text characters if the text has more characters than the key. f. Brute force solutions will not be accepted and an iterative approach should be used to solve this problem. g. The user will enter two strings one after the other: • the first will be the text to encrypt • the second will be the key used to encrypt it h. 0<=len(text)<=1000 0<=len(key)<=1000 len(key) can be greater than len(text) 2. Use the guiding codes below and complete the program. Run the sample inputs below and the codes must get the exact expected output. 3. Take a screenshot of your codes on your python complier and the expected output as well. def enc_text(text, key): encrypted_text ################################################### # place your code here # #################################################### return encrypted_text if_name text = input () key = input() print(enc_text(text, key)) main_": == SAMPLE INPUT 1 SAMPLE INPUT 2 SAMPLE INPUT 3 Hello World abracadabra expelliarmus! abb aa bbb EXPECTED OUTPUT EXPECTED OUTPUT EXPECTED OUTPUT cdtcecfcdtc Kbiol|Zlooa bumbiif^ojrp} PART 2 1. Using python, create a program with a function of dec_text(text, key) to decrypt text applying version of Ceasar Cipher described in PART 1. 2. Use the guiding codes below and complete the program. Run the sample inputs below and the codes must get the exact expected output. 3. Take a screenshot of your codes on your python complier and the expected output as well. def dec_text(encrypted_text, key): decrypted_text #################################################### # place your code here # #################################################### return decrypted_text if text = input () key = input() print(dec_text(text, key)) name main_": 3D SAMPLE INPUT 1 SAMPLE INPUT 2 SAMPLE INPUT 3 Kbiol|Zlooa cdtcecfcdtc bumbiif^ojrp} abb aa bbb EXPECTED OUTPUT EXPECTED OUTPUT EXPECTED OUTPUT Hello World abracadabra expelliarmus!
PART 1 1. Using python, create a program with a function of enc_text(text, key) to encrypt the variable text using the provided key (executing a modified version of Ceasar Cipher). Follow these rules: a. The key will contain a word made up of the letters 'a' and 'b'. The cipher will use the key to know the direction of the shift: • 'a' - the character must be shifted upwards • 'b' - the character is to be shifted downward. b. The number of characters in the key will determine the number of positions to shift. c. The given text variable will only have characters from the ASCII table (values between 32 and 126). d. If the key is invalid (contains characters other than a and b or an empty string) or the text is invalid (empty string), no encryption will happen and the function returns the original text. e. The key will loop through again to accommodate the additional text characters if the text has more characters than the key. f. Brute force solutions will not be accepted and an iterative approach should be used to solve this problem. g. The user will enter two strings one after the other: • the first will be the text to encrypt • the second will be the key used to encrypt it h. 0<=len(text)<=1000 0<=len(key)<=1000 len(key) can be greater than len(text) 2. Use the guiding codes below and complete the program. Run the sample inputs below and the codes must get the exact expected output. 3. Take a screenshot of your codes on your python complier and the expected output as well. def enc_text(text, key): encrypted_text ################################################### # place your code here # #################################################### return encrypted_text if_name text = input () key = input() print(enc_text(text, key)) main_": == SAMPLE INPUT 1 SAMPLE INPUT 2 SAMPLE INPUT 3 Hello World abracadabra expelliarmus! abb aa bbb EXPECTED OUTPUT EXPECTED OUTPUT EXPECTED OUTPUT cdtcecfcdtc Kbiol|Zlooa bumbiif^ojrp} PART 2 1. Using python, create a program with a function of dec_text(text, key) to decrypt text applying version of Ceasar Cipher described in PART 1. 2. Use the guiding codes below and complete the program. Run the sample inputs below and the codes must get the exact expected output. 3. Take a screenshot of your codes on your python complier and the expected output as well. def dec_text(encrypted_text, key): decrypted_text #################################################### # place your code here # #################################################### return decrypted_text if text = input () key = input() print(dec_text(text, key)) name main_": 3D SAMPLE INPUT 1 SAMPLE INPUT 2 SAMPLE INPUT 3 Kbiol|Zlooa cdtcecfcdtc bumbiif^ojrp} abb aa bbb EXPECTED OUTPUT EXPECTED OUTPUT EXPECTED OUTPUT Hello World abracadabra expelliarmus!
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:PART 1
1. Using python, create a program with a function of enc_text(text, key) to encrypt the variable text using the
provided key (executing a modified version of Ceasar Cipher). Follow these rules:
а.
The key will contain a word made up of the letters 'a' and 'b'. The cipher will use the key to know the
direction of the shift:
• 'a' - the character must be shifted upwards
• 'b' - the character is to be shifted downward.
b. The number of characters in the key will determine the number of positions to shift.
c. The given text variable will only have characters from the ASCII table (values between 32 and 126).
d. If the key is invalid (contains characters other than a and b or an empty string) or the text is invalid
(empty string), no encryption will happen and the function returns the original text.
e. The key will loop through again to accommodate the additional text characters if the text has more
characters than the key.
Brute force solutions will not be accepted and an iterative approach should be used to solve this
problem.
f.
g.
The user will enter two strings one after the other:
• the first will be the text to encrypt
• the second will be the key used to encrypt it
h. 0<=len(text)<=1000 0<=len(key)<=1000 len(key) can be greater than len(text)
2. Use the guiding codes below and complete the program. Run the sample inputs below and the codes must get
the exact expected output.
3. Take a screenshot of your codes on your python complier and the expected output as well.
def enc_text(text, key):
encrypted_text
2########################
############
# place your code here #
########################
return encrypted_text
if
main_":
_name
text = input()
key = input ()
print(enc_text(text, key))
SAMPLE INPUT 1
SAMPLE INPUT 2
SAMPLE INPUT 3
Hello World
abracadabra
expelliarmus!
abb
aa
bbb
EXPECTED OUTPUT
EXPECTED OUTPUT
EXPECTED OUTPUT
cdtcecfcdtc
Kbiol|Zlooa
bumbiif^ojrp}
PART 2
1. Using python, create a program with a function of dec_text(text, key) to decrypt text applying version of Ceasar
Cipher described in PART 1.
2. Use the guiding codes below and complete the program. Run the sample inputs below and the codes must get
the exact expected output.
3. Take a screenshot of your codes on your python complier and the expected output as well.
def dec_text(encrypted_text, key):
decrypted_text
##############
#####
######
# place your code here #
####################################################
return decrypted_text
if
main_":
name
text = input ()
key = input()
print(dec_text(text, key))
==
SAMPLE INPUT 1
SAMPLE INPUT 2
SAMPLE INPUT 3
Kbiol|Zlooa
cdtcecfcdtc
bumbiif^ojrp}
abb
aa
bbb
EXPECTED OUTPUT
EXPECTED OUTPUT
EXPECTED OUTPUT
Hello World
abracadabra
expelliarmus!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 5 images

Recommended textbooks for you
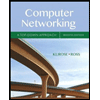
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
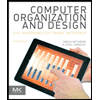
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
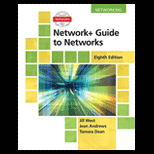
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
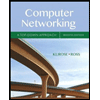
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
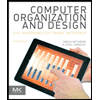
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
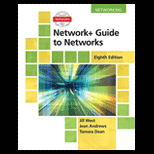
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
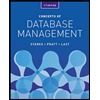
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
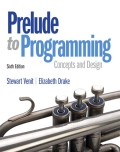
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
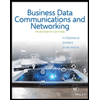
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY