param maze param currentPos param path The {@code Maze; object to solve param history The {@code Coordinate} obje The {@code ArrayList} of (@ from the start of the maze coordinates from start up to all the coordinates from sta The f@code ArrayList} of f@
param maze param currentPos param path The {@code Maze; object to solve param history The {@code Coordinate} obje The {@code ArrayList} of (@ from the start of the maze coordinates from start up to all the coordinates from sta The f@code ArrayList} of f@
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
The purpose of this assignment is to practice using Recursion with backtracking to solve a maze.
I need help to implement the private static void solve(Maze maze, Coordinate currentPos, ArrayList<Coordinate> path, ArrayList<Coordinate> history) method in the MazeSolver class.

Transcribed Image Text:```java
/**
* The recursive helper method that YOU will implement. This method will do all the work for
* solving the maze.
*
* @param maze The {@code Maze} object to solve.
* @param currentPos The {@code Coordinate} object that keeps track of your current position in the maze.
* @param path The {@code ArrayList} of {@code Coordinate} objects that are currently on the path
* from the start of the maze to the end. This ArrayList should only contain the
* coordinates from start up to your current position, and eventually should contain
* all the coordinates from start to end.
* @param history The {@code ArrayList} of {@code Coordinate} objects that you have already visited.
* This helps you to keep track of where you have been so you do not check the same
* position more than once.
*/
private static void solve(Maze maze, Coordinate currentPos, ArrayList<Coordinate> path, ArrayList<Coordinate> history) {
//THIS IS THE METHOD THAT YOU MUST IMPLEMENT.
//YOU MAY NOT CHANGE THE METHOD HEADER
}
/**
* Private method to print the final results once a solution has been found.
*
* @param maze A {@code Maze} object that we wish to display.
* @param path An ArrayList of {@code Coordinate} objects that are on the path from the start
* of the maze to the end.
*/
private static void printResult(Maze maze, ArrayList<Coordinate> path) {
for (int i = 0; i < maze.getNumRows(); i++) {
for (int j = 0; j < maze.getNumCols(); j++) {
Coordinate current = new Coordinate(i, j);
if (current.equals(maze.getStart())) {
System.out.print("S");
```
### Explanation:
This Java code represents part of a program to solve a maze using a recursive method. The code defines two methods:
1. **`solve` Method**:
- Designed as a recursive helper that is meant to be implemented to solve a given maze.
- **Parameters**:
- `maze`: The maze object to solve.
- `currentPos`: Keeps track of the current position within the maze.
- `path`: An `ArrayList` that tracks the path from the start of the maze to the end
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Recommended textbooks for you
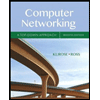
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
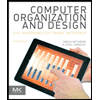
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
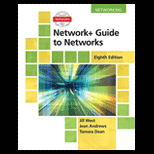
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
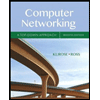
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
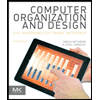
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
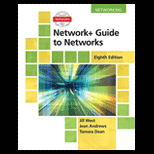
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
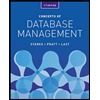
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
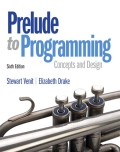
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
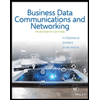
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY