Palindromes A palindrome is a string that is identical to itself when reversed. For example, "madam", "dad", and "abba" are palindromes. Note: the empty string is a palindrome, as is every string of length one. Write some code in the main () function to accomplish the following tasks: Having written code to extract data from the keyboard (stdin) in Lab 3, reuse that code to prompt the user to enter a string between 0 and 255 characters in length. You should ignore whitespace and punctuation, and all comparisons should be case-insensitive. Process the entries from the keyboard and store them in the queue ADT provided in the lab 5 code package. Use the is_palindrome () function ADT provided to implement a simple palindrome verification of the data entered. Here is the signature and documentation for the function: bool is_palindrome (char *text) Return true if text is a palindrome, false otherwise. Turn in your commented program and a screen shot (script) of the execution run of the program. Include some sample tests in your captured code run. Examples of valid palindromes: "a" "aa"
Palindromes A palindrome is a string that is identical to itself when reversed. For example, "madam", "dad", and "abba" are palindromes. Note: the empty string is a palindrome, as is every string of length one. Write some code in the main () function to accomplish the following tasks: Having written code to extract data from the keyboard (stdin) in Lab 3, reuse that code to prompt the user to enter a string between 0 and 255 characters in length. You should ignore whitespace and punctuation, and all comparisons should be case-insensitive. Process the entries from the keyboard and store them in the queue ADT provided in the lab 5 code package. Use the is_palindrome () function ADT provided to implement a simple palindrome verification of the data entered. Here is the signature and documentation for the function: bool is_palindrome (char *text) Return true if text is a palindrome, false otherwise. Turn in your commented program and a screen shot (script) of the execution run of the program. Include some sample tests in your captured code run. Examples of valid palindromes: "a" "aa"
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Ho do I code this in C

Transcribed Image Text:## Palindromes
A palindrome is a string that is identical to itself when reversed. For example, "madam", "dad", and "abba" are palindromes. Note: the empty string is a palindrome, as is every string of length one.
Write some code in the `main()` function to accomplish the following tasks:
- **Having written code to extract data from the keyboard (`stdin`) in Lab 3,** reuse that code to prompt the user to enter a string between 0 and 255 characters in length. You should ignore whitespace and punctuation, and all comparisons should be case-insensitive.
- **Process the entries from the keyboard and store them in the queue ADT provided in the Lab 5 code package.**
- **Use the `is_palindrome()` function ADT provided** to implement a simple palindrome verification of the data entered. Here is the signature and documentation for the function:
```c
bool is_palindrome(char *text)
```
Return true if text is a palindrome, false otherwise.
Turn in your commented program and a screenshot (`script`) of the execution run of the program. Include some sample tests in your captured code run. Examples of valid palindromes:
```
""
"a"
"aa"
```
```
"aaa"
"aba"
"abba"
"Taco cat"
"Madam, I'm Adam"
"A man, a plan, a canal: Panama"
"Doc, note: I dissent. A fast never prevents a fatness. I diet on cod."
```
---
This document contains explanations and sample code exercises designed for educational purposes, specifically focusing on the concept of palindromes in string processing.

Transcribed Image Text:**Educational Topic: Dynamic Arrays and Queue Operations in C Programming**
In this section, we will look at the essential files and function definitions used for handling dynamic arrays and queue operations in C.
### Files Overview
- **main.c**: Contains the main function code.
- **lab5.c**: Code specific to carrying out this lab exercise.
- **lab5.h**: Header definitions for functions specific to carrying out this lab exercise.
- **dynarray.c**: A dynamic array set of structures and functions for stacks and queues.
- **dynarray.h**: Header definitions for functions used for stacks and queues.
- **makefile**: A makefile that creates the "queue" executable easier (including clean).
### Quick Reference to Functions in `dynarray.c`
```c
typedef dynarray_t; // dynamic array struct
```
#### Basic/Common Operations
```c
void dynarray_init (dynarray_t *a); // initialize struct
void dynarray_free (dynarray_t *a); // free struct
size_t dynarray_size (dynarray_t *a); // return element count
size_t dynarray_is_empty(dynarray_t *a); // return true if empty
```
These functions provide basic operations:
- `dynarray_init`: Initializes the dynamic array structure.
- `dynarray_free`: Frees the allocated memory for the dynamic array.
- `dynarray_size`: Returns the number of elements in the dynamic array.
- `dynarray_is_empty`: Checks if the dynamic array is empty.
#### Stack Operations
```c
void dynarray_push(dynarray_t *a, data_t item); // add to back
data_t dynarray_pop (dynarray_t *a); // remove from back
data_t dynarray_top (dynarray_t *a); // return back
```
These functions handle stack operations:
- `dynarray_push`: Adds an item to the back of the dynamic array.
- `dynarray_pop`: Removes an item from the back of the dynamic array.
- `dynarray_top`: Returns the item at the back of the dynamic array.
#### Queue Operations
```c
void dynarray_enqueue(dynarray_t *a, data_t item); // add to back
data_t dynarray_dequeue(dynarray_t *a); // remove from front
data_t dynarray_front (dynarray_t *a); //
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
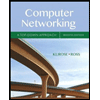
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
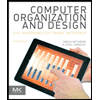
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
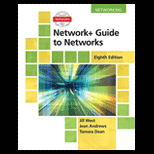
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
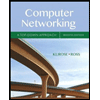
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
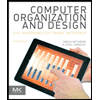
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
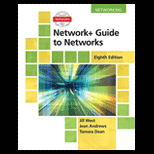
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
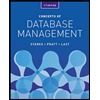
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
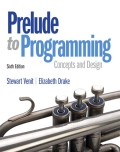
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
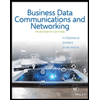
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY