overload the rest of the operators, add to the code arithmetic operators (multiplication, division but avoid division by zero, subtraction) as friend members. Comparison operators as friend members increment and decrement --p,p--,++p,p++ as member functions main.cpp #include #include "point.hpp" using namespace std; int main() { point p1(10, 12); point p2(2, 3); point p3; p3 = p1 + p2; p3.print(); pair P(2, 3); point p4 = p1 + P; p4.print(); point p5 = P + p1; p5.print(); return 0; } point.hpp #include #include "point.hpp" using namespace std; int main() { point p1(10, 12); point p2(2, 3); point p3; p3 = p1 + p2; p3.print(); pair P(2, 3); point p4 = p1 + P; p4.print(); point p5 = P + p1; p5.print(); return 0; }
overload the rest of the operators, add to the code
arithmetic operators (multiplication, division but avoid division by zero, subtraction) as friend members.
Comparison operators as friend members
increment and decrement --p,p--,++p,p++ as member functions
main.cpp
#include <iostream>
#include "point.hpp"
using namespace std;
int main() {
point p1(10, 12);
point p2(2, 3);
point p3;
p3 = p1 + p2;
p3.print();
pair <double, double> P(2, 3);
point p4 = p1 + P;
p4.print();
point p5 = P + p1;
p5.print();
return 0;
}
point.hpp
#include <iostream>
#include "point.hpp"
using namespace std;
int main() {
point p1(10, 12);
point p2(2, 3);
point p3;
p3 = p1 + p2;
p3.print();
pair <double, double> P(2, 3);
point p4 = p1 + P;
p4.print();
point p5 = P + p1;
p5.print();
return 0;
}

#include <iostream>
#include "point.hpp"
using namespace std;
int main()
{
point p1(10,12);
point p2(2,3);
cout<<"Points are:"<<endl;
p1.print();
p2.print();
point p3 ;
p3 = p1 + p2;
cout<<"sum of two points:"<<endl;
p3.print();
//p1+(2,3)
pair <double,double> P(2,3);
point p4 = p1 + P;
p4.print();
//(2,3) + p1
point p5 = P + p1;
p5.print ();
//
cout<<"product of two points:"<<endl;
point p6= p1 * p2;
p6.print();
cout<<"division of two points:"<<endl;
point p7= p1 / p2;
p7.print();
cout<<"Difference of two points:"<<endl;
point p8= p1 - p2;
p8.print();
return 0;
}
#include <iostream>
using namespace std;
#ifndef POINT_H
#define POINT_H
class point
{
public:
point(double X = 0, double Y = 0): x(X), y(Y) {}
void setX(double x)
{
this ->x=x;
}
double getX() const
{
return this->x ;
}
void setY(double y)
{
this->y=y ;
}
double getY() const
{
return this->y ;
}
void print() const ;
private:
double x, y;// Private data members
friend point operator+(point lhp, point rhp);
friend point operator*(point lhp, point rhp);
friend point operator/(point lhp, point rhp);
friend point operator-(point lhp, point rhp);
friend point operator+(point lhp, pair <double, double> rhp);
friend point operator+(pair <double, double> lhp, point rhp) ;
};
void point::print() const{
cout<<"x,y : ("<<this ->x<<","<<this->y<<")"<<endl;
}
point operator+(point lhp, point rhp){
return point(lhp.x +rhp.x, +lhp.y+rhp.y);
}
point operator+(point lhp, pair<double,double> rhp){
return point(lhp.x +rhp.first, lhp.y +rhp.second);
}
point operator+(pair<double,double> lhp, point rhp){
return point(lhp.first +rhp.x, lhp.second +rhp.y);
}
point operator*(point lhp, point rhp){
return point(lhp.x *rhp.x, lhp.y*rhp.y);
}
point operator/(point lhp, point rhp){
return point(lhp.x /rhp.x, lhp.y/rhp.y);
}
point operator-(point lhp, point rhp){
return point(lhp.x -rhp.x, lhp.y-rhp.y);
}
#endif
Step by step
Solved in 2 steps with 1 images

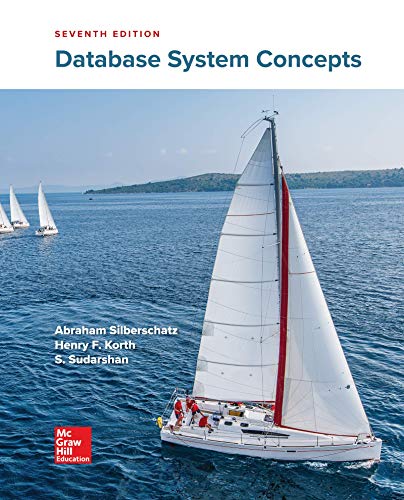
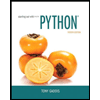
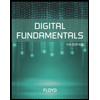
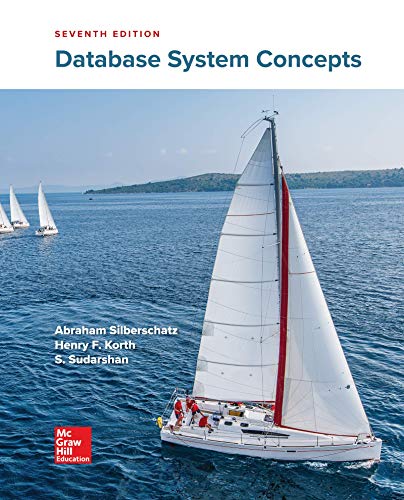
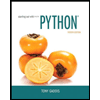
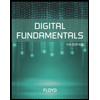
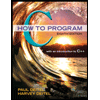
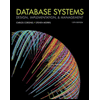
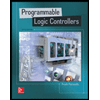