(our program must have function ma in, function presentValue, three read functions, and a display function. Including main this is six unctions. The presentValue function must have the following signature: double presentValue (double futureValue, double interestRate, int numberYears) The presentvalue needs to calculate the present value and return that back to the calling function. The formula for this is above. Note hat the annual interest would be .08 for 8%. Failure to follow the C++ requirements could reduce the points received from passing the tests. Seneral overview n part 2 you will be creating multiple functions to calculate the present value. (ou may be asking what a 'present value" is. Suppose you want to deposit a certain amount of money into a savings account and then save it alone to draw interest for some amount of time, say 12 years. At the end of the 12 years you want to have $15,000 in the account. The present value is the amount of money you would have to deposit today to have $15,000 in 12 years. The formula used needs the future value (F) and annual interest rate (r) and the number of years (n) the money will sit in the account, anchanged. You will be calculating the present value (P). > = F/ (1 +r)^n) n the above expression the value (1 + r) needs to be raised to the nth power. Assume that is the power function and x^2 is x to the 2nd power (x squared) rou are not allowed to use any global variables. Use of global variables will result in a grade of zero for part 2. Three read functions (ou must have functions to read in the future value, the annual interest rate, and the number of years. That would be three different unctions. Give these functions meaningful names. Note that the order of the values will be future value, annual interest rate, and number of ears. n all cases you need to return any valid value back to the calling function. For all three functions you will write out to cout as prompt for an input value. You will read in that value from cin. If the value is invalid zero or negative) you need to display an error message and reread the value (with another prompt). You need to do this in a loop and continue looping until a valid value has been entered. Only the number of years can be an snt value. The rest should be of type double. tere are the prompts for the three values you need to read in: Enter future value Enter annual interest rate Enter number of years Note that the interest rate will be a number such as 10 or 12.5. These are to be read in as percentages (10% and 12.5%). You will need to ivide these values by 100 to convert them into the values needed in the function (,1 and.125 for the above values). This conversion needs o be done before you call the presentValue function (see below). If you do the conversion in the presentValue function your orogram will failthe unit tests, so do the conversion before you call the calculate function. Here are the error messages you need to display if the values are negative:
(our program must have function ma in, function presentValue, three read functions, and a display function. Including main this is six unctions. The presentValue function must have the following signature: double presentValue (double futureValue, double interestRate, int numberYears) The presentvalue needs to calculate the present value and return that back to the calling function. The formula for this is above. Note hat the annual interest would be .08 for 8%. Failure to follow the C++ requirements could reduce the points received from passing the tests. Seneral overview n part 2 you will be creating multiple functions to calculate the present value. (ou may be asking what a 'present value" is. Suppose you want to deposit a certain amount of money into a savings account and then save it alone to draw interest for some amount of time, say 12 years. At the end of the 12 years you want to have $15,000 in the account. The present value is the amount of money you would have to deposit today to have $15,000 in 12 years. The formula used needs the future value (F) and annual interest rate (r) and the number of years (n) the money will sit in the account, anchanged. You will be calculating the present value (P). > = F/ (1 +r)^n) n the above expression the value (1 + r) needs to be raised to the nth power. Assume that is the power function and x^2 is x to the 2nd power (x squared) rou are not allowed to use any global variables. Use of global variables will result in a grade of zero for part 2. Three read functions (ou must have functions to read in the future value, the annual interest rate, and the number of years. That would be three different unctions. Give these functions meaningful names. Note that the order of the values will be future value, annual interest rate, and number of ears. n all cases you need to return any valid value back to the calling function. For all three functions you will write out to cout as prompt for an input value. You will read in that value from cin. If the value is invalid zero or negative) you need to display an error message and reread the value (with another prompt). You need to do this in a loop and continue looping until a valid value has been entered. Only the number of years can be an snt value. The rest should be of type double. tere are the prompts for the three values you need to read in: Enter future value Enter annual interest rate Enter number of years Note that the interest rate will be a number such as 10 or 12.5. These are to be read in as percentages (10% and 12.5%). You will need to ivide these values by 100 to convert them into the values needed in the function (,1 and.125 for the above values). This conversion needs o be done before you call the presentValue function (see below). If you do the conversion in the presentValue function your orogram will failthe unit tests, so do the conversion before you call the calculate function. Here are the error messages you need to display if the values are negative:
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C++
![### Programming Assignment: Present Value Calculation
In this assignment, you will create a program to calculate the present value based on future value, annual interest rate, and number of years. Your program must include a `main` function, `presentValue`, and supporting functions such as input and output display. Ensure no global variables are used.
#### Function Signature
```cpp
double presentValue(double futureValue, double interestRate, int numberYears)
```
Use the formula:
\[ P = \frac{F}{(1 + r)^n} \]
- \( P \) = Present Value
- \( F \) = Future Value
- \( r \) = Annual Interest Rate (as a decimal)
- \( n \) = Number of Years
### Input Requirements
- Future Value must be greater than zero.
- Interest Rate must be positive.
- Number of Years must be positive.
### Conversion
Interest rates should be input as percentages (e.g., 10 for 10%); convert to decimals inside your program (e.g., 0.10).
### Error Messages
In case of invalid inputs, display:
- "The future value must be greater than zero"
- "The interest rate must be greater than zero"
- "The number of years must be greater than zero"
### Sample Output
For a future value of $100,000, an interest rate of 2.5%, and a term of 15 years:
```
Present value: 64064.56
Future value: 100000.00
Annual interest rate: 2.5
Years: 15
```
### Program Structure
- Define input and validation functions to get valid future value, interest rate, and number of years.
- Implement the `presentValue` function to compute the present value based on the inputs.
- Write a display function to show the calculated and input values.
- `main` should coordinate the functions, display results, and handle multiple entries.
#### Example Inputs and Outputs
- **Input:**
```
100000.0
2.5
15
```
- **Output:**
```
Present value: 64064.56
Future value: 100000.00
Annual interest rate: 2.5
Years: 15
```
- **Input:**
```
-100
0.0](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F76b36f83-c1e4-4a45-8d99-813f462bfa30%2F803bdfe1-a9d0-49ae-9753-b22720890390%2Fye0xknq_processed.png&w=3840&q=75)
Transcribed Image Text:### Programming Assignment: Present Value Calculation
In this assignment, you will create a program to calculate the present value based on future value, annual interest rate, and number of years. Your program must include a `main` function, `presentValue`, and supporting functions such as input and output display. Ensure no global variables are used.
#### Function Signature
```cpp
double presentValue(double futureValue, double interestRate, int numberYears)
```
Use the formula:
\[ P = \frac{F}{(1 + r)^n} \]
- \( P \) = Present Value
- \( F \) = Future Value
- \( r \) = Annual Interest Rate (as a decimal)
- \( n \) = Number of Years
### Input Requirements
- Future Value must be greater than zero.
- Interest Rate must be positive.
- Number of Years must be positive.
### Conversion
Interest rates should be input as percentages (e.g., 10 for 10%); convert to decimals inside your program (e.g., 0.10).
### Error Messages
In case of invalid inputs, display:
- "The future value must be greater than zero"
- "The interest rate must be greater than zero"
- "The number of years must be greater than zero"
### Sample Output
For a future value of $100,000, an interest rate of 2.5%, and a term of 15 years:
```
Present value: 64064.56
Future value: 100000.00
Annual interest rate: 2.5
Years: 15
```
### Program Structure
- Define input and validation functions to get valid future value, interest rate, and number of years.
- Implement the `presentValue` function to compute the present value based on the inputs.
- Write a display function to show the calculated and input values.
- `main` should coordinate the functions, display results, and handle multiple entries.
#### Example Inputs and Outputs
- **Input:**
```
100000.0
2.5
15
```
- **Output:**
```
Present value: 64064.56
Future value: 100000.00
Annual interest rate: 2.5
Years: 15
```
- **Input:**
```
-100
0.0
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Recommended textbooks for you
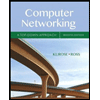
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
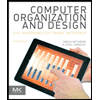
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
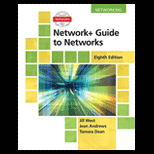
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
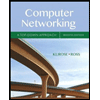
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
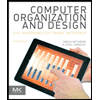
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
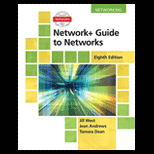
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
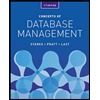
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
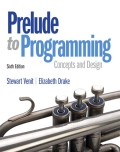
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
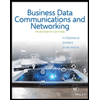
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY