| Exercise16_21 1미지 30 | Exercise16_21 29 | Exercise16_21 28 (d) х
Write a
in seconds in the text field and press the Enter key to count down the seconds,
as shown in Figure d . The remaining seconds are redisplayed
every second.
When the seconds are expired, the program starts to play music
continuously.


Program:
/*Import necessary packages into program*/
import java.io.File;
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.text.Font;
import javafx.stage.Stage;
import javafx.util.Duration;
//Class definition
public class Exercise16_21 extends Application
{
/*Object definition for “textfield”*/
TextField txtsec = new TextField();
/*Object definition for Timeline*/
Timeline aniTimeline = new Timeline(
new KeyFrame(Duration.millis(1000), e ->
{
int seconds = Integer.parseInt(txtsec.getText());
txtsec.setText(seconds - 1 + "");
if (seconds - 1 <= 0)
{
stopAnimation();
}
}));
//Define the music file into variable
String bip="C:\\Users\\Public\\Music\\Sample Music\\Kalimba.mp3";
//Define the object for "Media"
Media media = new Media(new File(bip).toURI().toString());
//Define the object for "MediaPlayer"
MediaPlayer mp = new MediaPlayer(media);
/*Method definition for application*/
public void start(Stage primaryStage)
{
/*Define the count for timeline*/
mp.setCycleCount(Timeline.INDEFINITE);
/*Define the cycle count*/
aniTimeline.setCycleCount(Timeline.INDEFINITE);
/*Define alignment for text field*/
txtsec.setAlignment(Pos.CENTER);
/*Define alignment for text second*/
txtsec.setOnAction(e -> {
/*Playing the animation*/
aniTimeline.play();
});
//Set the font for text field
txtsec.setFont(Font.font("Times", 35));
/*Define the "Scene" for text field*/
Scene scene = new Scene(txtsec, 200, 100);
/*Set the title for aplication*/
primaryStage.setTitle("Exercise16_21");
/*Set the Scene*/
primaryStage.setScene(scene);
//Display the application
primaryStage.show();
}
/*Method definition for stop the animation*/
private void stopAnimation()
{
//Stop the animation
aniTimeline.stop();
//Play the animation
mp.play();
}
//Main method
public static void main(String[] args)
{
/*Launch the application*/
launch(args);
}
}
Output:
- If the timer reaches “0”, it plays the “mp3” music which is mentioned on code.
Step by step
Solved in 3 steps with 3 images

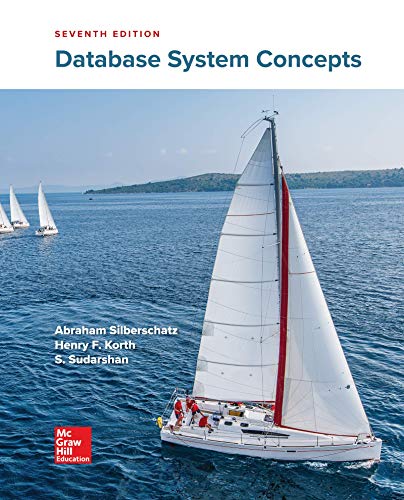
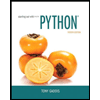
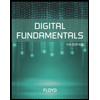
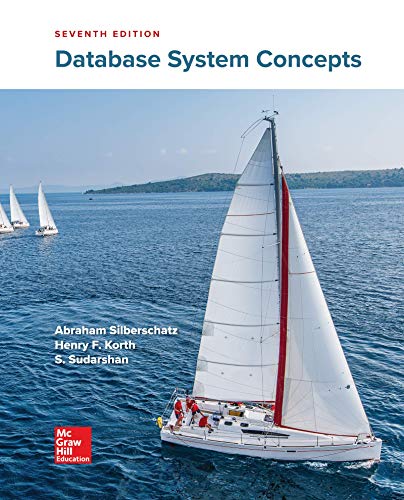
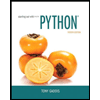
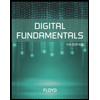
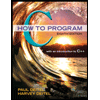
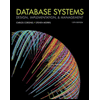
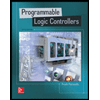