Objectives > Using file input output > Processing records Practice with exceptions Command Prompt-pyth... -MENU---- Print Address Book (1) Search Contact (2) Add new Contact (3) Delete Contact (4) Quit (5) Enter your choice K Problem Create a program (addressbook.py) that will manage an individual's address book. The program should allow a user to add new contacts, print the entire address book, search for a contact, delete a contact, and exit the program. These features should work as follows: Part 1: Create a function named Menu to display the menu to the user that contains all the above options using numbers from 1 to 5 (see output above). In function Menu, validate the user input. If validation fails, display a message to the user and display the menu again. If validation is successful, return the user selected choice value from the menu. Create the following functions and simply display the function name within each function (just for testing the menu in this part): > PrintAddress Book > Search Contact > fAddContact >Delete Contact Create function main which is used to orchestrate the entire program. In main, call function Menu and store the returned value in variable choice. Test the variable choice for its value and then call the corresponding function (which will print the function name, for now). For the exit option, ask the user whether they want to continue (yin). If they would like to continue, then display the menu and get input from the user. If not, exit the program Validate the user input, and if the input is not y or n then ask the user again whether they want to continue. Test the functionality in this part before continuing Part 2: Remove the print statements from the individual functions. Add the following functionality to each function: Add new contact: For this option, get the name, street address, and city state zipcode (one line), from the user. Write add them to a file contacts.txt. Write out the file such that all the information about an individual is on separate lines. For example, the name "Mickey" and address "DisneyLand" should be on separate lines rather than on the same line.
Objectives > Using file input output > Processing records Practice with exceptions Command Prompt-pyth... -MENU---- Print Address Book (1) Search Contact (2) Add new Contact (3) Delete Contact (4) Quit (5) Enter your choice K Problem Create a program (addressbook.py) that will manage an individual's address book. The program should allow a user to add new contacts, print the entire address book, search for a contact, delete a contact, and exit the program. These features should work as follows: Part 1: Create a function named Menu to display the menu to the user that contains all the above options using numbers from 1 to 5 (see output above). In function Menu, validate the user input. If validation fails, display a message to the user and display the menu again. If validation is successful, return the user selected choice value from the menu. Create the following functions and simply display the function name within each function (just for testing the menu in this part): > PrintAddress Book > Search Contact > fAddContact >Delete Contact Create function main which is used to orchestrate the entire program. In main, call function Menu and store the returned value in variable choice. Test the variable choice for its value and then call the corresponding function (which will print the function name, for now). For the exit option, ask the user whether they want to continue (yin). If they would like to continue, then display the menu and get input from the user. If not, exit the program Validate the user input, and if the input is not y or n then ask the user again whether they want to continue. Test the functionality in this part before continuing Part 2: Remove the print statements from the individual functions. Add the following functionality to each function: Add new contact: For this option, get the name, street address, and city state zipcode (one line), from the user. Write add them to a file contacts.txt. Write out the file such that all the information about an individual is on separate lines. For example, the name "Mickey" and address "DisneyLand" should be on separate lines rather than on the same line.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
PLeae write in python
The populated contacts.txt file with at least 5 contacts
1 screenshot of executed code in command line/terminal window for the print address book option (either paste
into Word document or as an image)
1 screenshot of executed code in command line/terminal window for the search contact option (either paste into
Word document or as an image)

Transcribed Image Text:Objectives
> Using file input/output
> Processing records
> Practice with exceptions
Lab 4
Command Prompt-pyth...
-MENU--
Print Address Book (1)
Search Contact (2)
Add new Contact (3)
Delete Contact (4)
Quit (5)
Enter your choice
> PrintAddress Book
➤ fSearch Contact
>fAddContact
<
Problem
Create a program (addressbook.py) that will manage an individual's address book. The program should allow a user to add
new contacts, print the entire address book, search for a contact, delete a contact, and exit the program. These features
should work as follows:
Part 1:
Create a function named Menu to display the menu to the user that contains all the above options using numbers from 1
to 5 (see output above). In function fMenu, validate the user input. If validation fails, display a message to the user and
display the menu again. If validation is successful, return the user selected choice value from the menu.
Create the following functions and simply display the function name within each function (just for testing the menu in this
part):
>Delete Contact
Create function main which is used to orchestrate the entire program. In main, call function fMenu and store the returned
value in variable choice. Test the variable choice for its value and then call the corresponding function (which will print
the function name, for now).
For the exit option, ask the user whether they want to continue (y/n). If they would like to continue, then display the menu
and get input from the user. If not, exit the program. Validate the user input, and if the input is not y or n then ask the user
again whether they want to continue.
Test the functionality in this part before continuing.
Part 2:
Remove the print statements from the individual functions. Add the following functionality to each function:
Add new contact: For this option, get the name, street address, and city state zipcode (one line), from the user. Write add
them to a file contacts.txt. Write out the file such that all the information about an individual is on separate lines. For
example, the name "Mickey" and address "DisneyLand" should be on separate lines rather than on the same line.
![Print the Address Book: For this option, print all the contents of the address book.
Each record should be separated by a line of hyphens (see output)
Search for a contact: For this option, ask the user for the name of the contact who
they are looking for. Based on the name, look for that individual in the file one line
Mary
at a time and, if they are found, display all the information for that individual. If the Almaden ave
San Jose, CA 90740
individual is not found, then display a message to the user saying "Contact [name]
not found" where [name] is the name that the user searched for.
Delete a contact: For this option, ask the user for the name of the contact who they
want to delete from the address book. There are two different methods:
23 Command Prompt-pyth...
enter your choice 1
Michael
1234 Test Ave
San Francisco, CA 94105
Suzan
45 Hope St
seryville, CA 94512
Do you want to continue) (y/n).
> Write the all contacts except the one to be deleted to a new file, then delete the contacts.txt file, and rename the
new file back to contacts.txt.
> Store the all contacts except the one to be deleted in a variable and then overwrite the file contacts.txt with the
content of the variable
Test this part using all menu options. Populate the contacts file with at least 5 contacts.
Part 3:
You may have already noticed that the file contacts is opened in multiple functions. Create a new function named
fFileOpen having the filename and the mode as parameters. Use the open method using the two parameters to open the
file and assign it into a variable. Return the variable back to the calling program. Use exception handling to test for the
condition when the file is not found.
Replace the open method in all the functions with the function call fFileOpen.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb97d67e1-dda9-48ae-b6f6-b8910f9a7c16%2F6478aa18-b210-4fd9-90a7-83f3769c70ad%2F6efj2zg_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Print the Address Book: For this option, print all the contents of the address book.
Each record should be separated by a line of hyphens (see output)
Search for a contact: For this option, ask the user for the name of the contact who
they are looking for. Based on the name, look for that individual in the file one line
Mary
at a time and, if they are found, display all the information for that individual. If the Almaden ave
San Jose, CA 90740
individual is not found, then display a message to the user saying "Contact [name]
not found" where [name] is the name that the user searched for.
Delete a contact: For this option, ask the user for the name of the contact who they
want to delete from the address book. There are two different methods:
23 Command Prompt-pyth...
enter your choice 1
Michael
1234 Test Ave
San Francisco, CA 94105
Suzan
45 Hope St
seryville, CA 94512
Do you want to continue) (y/n).
> Write the all contacts except the one to be deleted to a new file, then delete the contacts.txt file, and rename the
new file back to contacts.txt.
> Store the all contacts except the one to be deleted in a variable and then overwrite the file contacts.txt with the
content of the variable
Test this part using all menu options. Populate the contacts file with at least 5 contacts.
Part 3:
You may have already noticed that the file contacts is opened in multiple functions. Create a new function named
fFileOpen having the filename and the mode as parameters. Use the open method using the two parameters to open the
file and assign it into a variable. Return the variable back to the calling program. Use exception handling to test for the
condition when the file is not found.
Replace the open method in all the functions with the function call fFileOpen.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
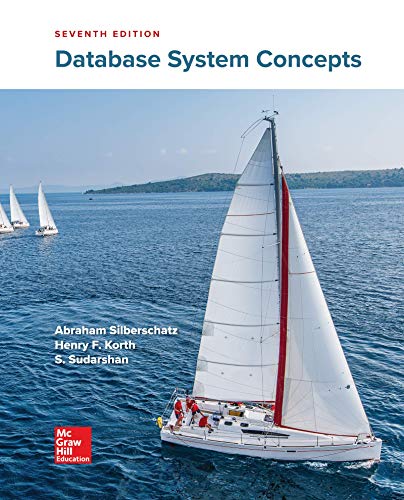
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
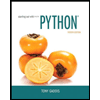
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
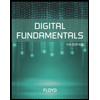
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
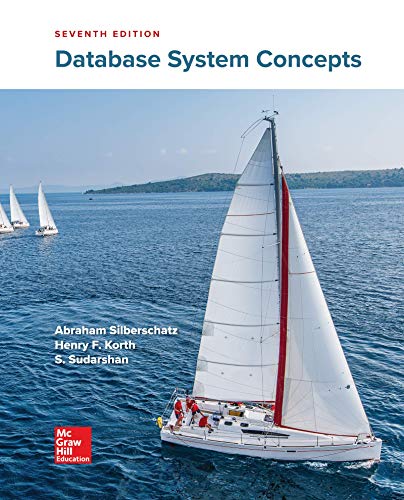
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
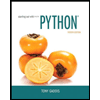
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
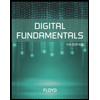
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
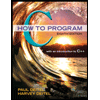
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
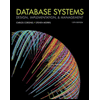
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
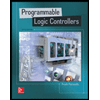
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education