nsecutive P
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Consecutive Prime Sum:-.
![# Consecutive Prime Sum
Some prime numbers can be expressed as a sum of consecutive prime numbers.
### For example:
- \( 5 = 2 + 3 \)
- \( 17 = 2 + 3 + 5 + 7 \)
- \( 41 = 2 + 3 + 5 + 7 + 11 + 13 \)
Your task is to find out how many prime numbers which satisfy this property are present in the range \( 3 \) to \( N \) subject to a given constraint. Write code to find out the number of prime numbers that satisfy the above-mentioned property in a given range.
### Input Format:
First line contains a number \( N \)
### Output Format:
Print the total number of all such prime numbers which are less than or equal to \( N \).
### Constraints:
\[ 2 < N \leq 12,000,000,000 \]
```cpp
/*
#include<iostream>
#include<math.h>
using namespace std;
bool isPrime(int n)
{
if(n==1)
return false;
else{
for(int i=2;i<=(int)sqrt(n);i++)
{
if(n%i == 0)
return false;
}
return true;
}
}
bool binarySearch(int a[],int n,int k)
{
int first = 0;
int last = n-1;
while(first <= last)
{
int mid = (first + last)/2;
if(a[mid]>k)
last = last -1;
else if(a[mid]<k)
first = first + 1;
else if(a[mid]==k)
return true;
}
return false;
}
int main(int argc, char const *argv[])
{
int count = 0,n,sum=2,prime_count=0;
cin>>n;
int primelist[n];
for(int i=2;i<=n;i++)
{
if(isPrime(i)){
primelist[count]=i;
count++;
}
}
for(int i=1;i<count;i++){
sum = sum + primelist[i];
if(binarySearch(primelist,count,sum))
{
prime_count++;
}
}
cout<<"Total number of all such prime numbers are:"<<prime_count;
return 0;
}
```
### Explanation](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F975eedeb-cca9-452a-a623-85539861d2ea%2F1a082fb8-5118-4700-8df3-ae14eddf199e%2Ftswdzbc_processed.jpeg&w=3840&q=75)
Transcribed Image Text:# Consecutive Prime Sum
Some prime numbers can be expressed as a sum of consecutive prime numbers.
### For example:
- \( 5 = 2 + 3 \)
- \( 17 = 2 + 3 + 5 + 7 \)
- \( 41 = 2 + 3 + 5 + 7 + 11 + 13 \)
Your task is to find out how many prime numbers which satisfy this property are present in the range \( 3 \) to \( N \) subject to a given constraint. Write code to find out the number of prime numbers that satisfy the above-mentioned property in a given range.
### Input Format:
First line contains a number \( N \)
### Output Format:
Print the total number of all such prime numbers which are less than or equal to \( N \).
### Constraints:
\[ 2 < N \leq 12,000,000,000 \]
```cpp
/*
#include<iostream>
#include<math.h>
using namespace std;
bool isPrime(int n)
{
if(n==1)
return false;
else{
for(int i=2;i<=(int)sqrt(n);i++)
{
if(n%i == 0)
return false;
}
return true;
}
}
bool binarySearch(int a[],int n,int k)
{
int first = 0;
int last = n-1;
while(first <= last)
{
int mid = (first + last)/2;
if(a[mid]>k)
last = last -1;
else if(a[mid]<k)
first = first + 1;
else if(a[mid]==k)
return true;
}
return false;
}
int main(int argc, char const *argv[])
{
int count = 0,n,sum=2,prime_count=0;
cin>>n;
int primelist[n];
for(int i=2;i<=n;i++)
{
if(isPrime(i)){
primelist[count]=i;
count++;
}
}
for(int i=1;i<count;i++){
sum = sum + primelist[i];
if(binarySearch(primelist,count,sum))
{
prime_count++;
}
}
cout<<"Total number of all such prime numbers are:"<<prime_count;
return 0;
}
```
### Explanation
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
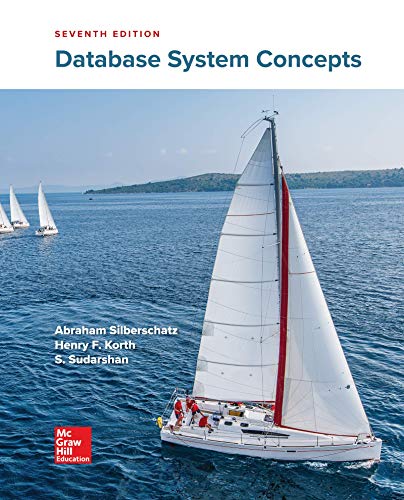
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
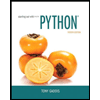
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
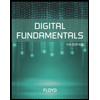
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
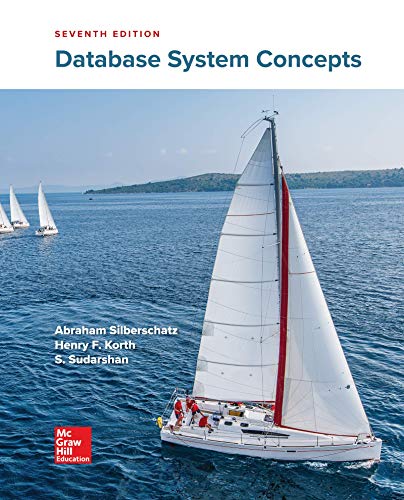
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
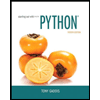
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
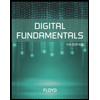
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
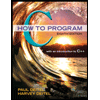
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
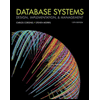
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
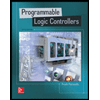
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education