Now modify the program to display the averages of homework, quizzes and tests for each student using a nested for loop and the len() function to calculate the average of each grade your read as you loop.
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
![Program #3: Gradebook Report (60 pts)
• Create a Python program named Exam-3.py
• Create three dictionaries: 1loyd, alice, and tyler.
• Give each dictionary the keys "name", "homework", "quizzes", and
"tests".
Now copy this code:
lloyd = {
"name": "Lloyd",
"homework": [90.e,97.e,75.e,92.0],
"quizzes": [88.0,40.0,94.0],
"tests": [75.0,90.0]
alice = {
"name": "Alice",
"homework": [100.e, 92.0, 98.e, 100.0],
"quizzes": [82.0, 83.0, 91.0],
"tests": [89.e, 97.e]
tyler = {
"name": "Tyler",
"homework": [e.e, 87.e, 75.e, 22.e],
"quizzes": [0.0, 75.0, 78.0],
"tests": [100.0, 100.0]
Add this code to create a list to include the above dictionaries as items in the list
called Student:
students-[1loyd, alice, tyler]
Add this code to display each student's name and the values of their grades for
each category:
for student in students:
print(student["name"])
print (student["homework" ])
print (student "quizzes"])
print (student["tests"])](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6c09ee89-7aaf-40a8-885a-22523f04bf20%2Fe44a7ddf-1b6e-4510-b624-4ebb39473997%2Fivsbcwl_processed.jpeg&w=3840&q=75)
![Once your run the program it should display something like this:
Lloyd
[90.0, 97.0, 75.0, 92.0]
[88.0, 40.0, 94.0]
[75.0, 90.0]
Alice
[100.0, 92.0, 98.0, 100.0]
[82.0, 84.0, 92.0]
[89.0, 97.0]
Tyler
[0.0, 87.0, 75.0, 22.0]
[0.0, 75.0, 78.0]
[100.0, 100.0]
Now modify the program to display the averages of homework, quizzes and tests for
each student using a nested for loop and the len() function to calculate the average
of each grade your read as you loop.
Final outcome after modification:
Lloyd
Homework average is: 88.5
Quizzes average is: 74.0
Tests average is: 82.5
Alice
Homework average is: 97.5
Quizzes average is: 86.0
Tests average is: 93.0
Tyler
Homework average is: 46.0
Quizzes average is: 51.0
Tests average is: 100.0
Hint (for the nested loop):
hw=0
#print (student["homework"])
for h in student["homework"]:
hw+= h
print ("Homework average is:", hw/len(student["homework"]))](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6c09ee89-7aaf-40a8-885a-22523f04bf20%2Fe44a7ddf-1b6e-4510-b624-4ebb39473997%2F5m9x1xl_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 1 images

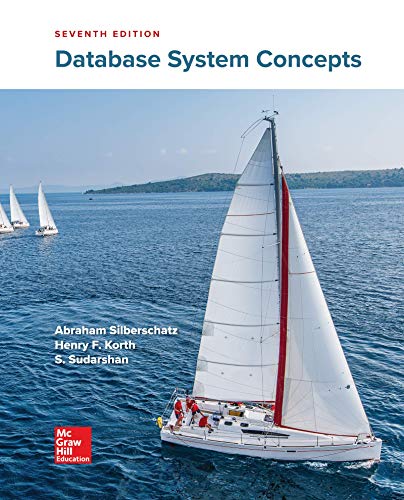
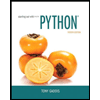
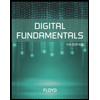
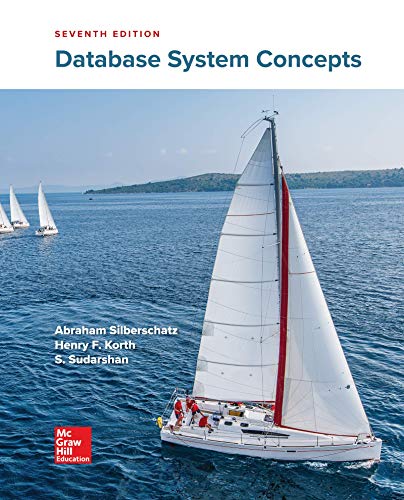
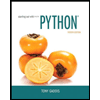
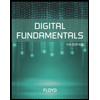
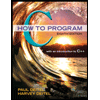
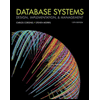
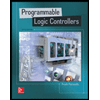