Note that testing this function is a little tricky because it relies on randomness. You will not see the same output every time! We can control for this a bit by running the function many times, to ensure that on average, the probabilities roughly match the outcome. For example, if we run weighted_choice({'green': 1, 'eggs': 3, 'ham': 2}) 600 times, then we would expect to get 'green' about 100 times, 'eggs' about 300 times, and 'ham' about 200 times. >>> results = 1, 'eggs': 3, 'ham':2}) for i in range (600)] >>> results.count('green') 99 >>> results.count('eggs') 296 [weighted_choice({ 'green': >>> results.count('ham') 205 If your results are consistently off by more than 20 or so, you should probably check your algorithm.
Note that testing this function is a little tricky because it relies on randomness. You will not see the same output every time! We can control for this a bit by running the function many times, to ensure that on average, the probabilities roughly match the outcome. For example, if we run weighted_choice({'green': 1, 'eggs': 3, 'ham': 2}) 600 times, then we would expect to get 'green' about 100 times, 'eggs' about 300 times, and 'ham' about 200 times. >>> results = 1, 'eggs': 3, 'ham':2}) for i in range (600)] >>> results.count('green') 99 >>> results.count('eggs') 296 [weighted_choice({ 'green': >>> results.count('ham') 205 If your results are consistently off by more than 20 or so, you should probably check your algorithm.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In python
![Note that testing this function is a little tricky because it
relies on randomness. You will not see the same output
every time! We can control for this a bit by running the
function many times, to ensure that on average, the
probabilities roughly match the outcome. For example, if
we run
weighted_choice({'green': 1, 'eggs': 3,
'ham': 2})
600 times, then we would expect to get 'green' about 100
times, 'eggs' about 300 times, and 'ham' about 200 times.
>>> results =
1, 'eggs': 3, 'ham':2}) for i in
range (600)]
>>> results.count('green')
99
>>> results.count('eggs')
296
>>> results.count('ham')
205
[weighted_choice({ 'green':
If your results are consistently off by more than 20 or so,
you should probably check your algorithm.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8b128b53-d6cf-49c2-8658-2db63d0fdace%2Ff85a59d7-2e7b-4290-b725-3eecae221eeb%2F2nm526t_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Note that testing this function is a little tricky because it
relies on randomness. You will not see the same output
every time! We can control for this a bit by running the
function many times, to ensure that on average, the
probabilities roughly match the outcome. For example, if
we run
weighted_choice({'green': 1, 'eggs': 3,
'ham': 2})
600 times, then we would expect to get 'green' about 100
times, 'eggs' about 300 times, and 'ham' about 200 times.
>>> results =
1, 'eggs': 3, 'ham':2}) for i in
range (600)]
>>> results.count('green')
99
>>> results.count('eggs')
296
>>> results.count('ham')
205
[weighted_choice({ 'green':
If your results are consistently off by more than 20 or so,
you should probably check your algorithm.

Transcribed Image Text:As part of problem C, we're going to randomly select
words to create sentences. However, we don't want it to
be uniformly random. We will want to influence the
random selection by having some words be more likely to
appear than others. This is called weighted random
selection. In this problem, we will build towards that goal
by creating a function that does a weighted random
selection from the keys of a dictionary, using the values
as the weights.
Create a function weighted_choice that takes as a
parameter a dictionary of words and their corresponding
counts. The function should randomly choose one of the
words, using the counts as weights in the selection. For
example, if I pass the dictionary
{'green': 1, 'eggs': 1}
To this function, it should return “green” half the time and
"eggs" half the time. If I pass the dictionary
{'green': 1, 'eggs': 3, 'ham': 2}
It should return "green" one-sixth of the time, "eggs" half
(3/6) of the time, and "ham" one-third (2/6) of the time.
There are a number of ways to accomplish this task.
Many solutions require you to iterate over the given
dictionary to add up the counts to get the total of the
counts before using a function from the random module
to get a random number in the range from 1 to the total.
Then, you can use that number to figure out which word
it corresponds to and return the appropriate word.
There's also a simple method that utilizes making a list
with x copies of each key (where x is the value for said
key), and then using random.choice.
Examples (random, so won't necessarily match, but
make sure that both come up about 50% of the time):
>>> weighted_choice({'green': 1, 'eggs':
1})
'green'
>>> weighted_choice({'green': 1,
1})
eggs':
'eggs'
>>> weighted_choice({'green': 1, 'eggs':
1})
'eggs'
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
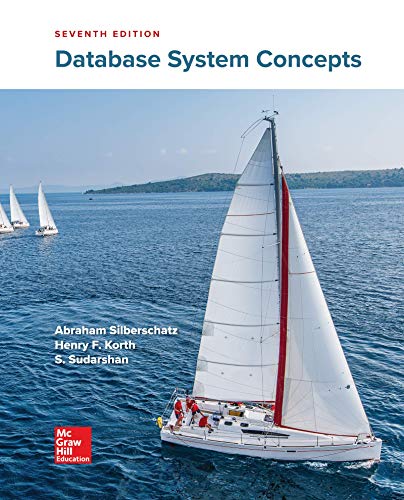
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
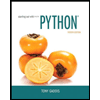
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
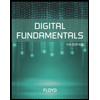
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
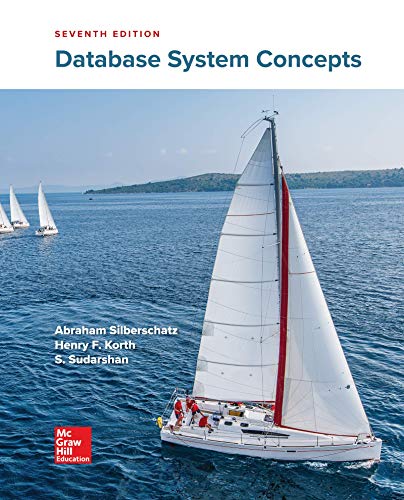
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
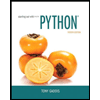
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
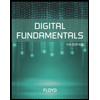
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
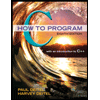
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
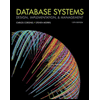
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
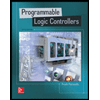
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education