// New Function prototypes void showValues(const int *, int); // display 10 numbers in a line from the array void fillArray(int *, int, int, int); // fill out random numbers between low and high into the array bool searchValue(const int *, int, int); // search value from the array void sortArray( int *, int); //sort the array in an ascending order void swap(int *, int *); //swap the values Modify the code below to create 50 random numbers instead of 10 and use the above new function protoypes. In the output, remove the display of the minimum, maximum and average from the array. Modify the showValues() function to display 10 numbers in a line from the array. [Part 2] Add the two functions to sort an array in an ascending order: void sortArray(int*, int); //ascending order void swap(int*, int*); In the sortArray() function called the swap() function to swap the values from two elements in the array. Code: #include #include #include #include using namespace std; // Function prototype void showValues(const int[], int); void fillArray(int[], int, int, int); int maximum(const int[], int); int minimum(const int[], int); double average(const int[], int); int main() { const int ARRAY_SIZE = 10; int numbers[ARRAY_SIZE]; srand(time(0)); int low = 0, high = 1; cout << "**This program will generate 10 random integers between [low] and [high]**" << endl; // prompt to get the user input for the low and high do { cout << "Please enter an integer value for the value for lower bound: "; cin >> low; cout << "Please enter an integer value for the value for higher bound: "; cin >> high; if (low > high) cout << "The lower bound should be smaller than the higher bound! \n"; } while (low > high); // .... // fill out the random numbers in the array fillArray(numbers, ARRAY_SIZE, low, high); cout << "\nThe 10 random integers between " << low << " and " << high << " are:" << endl; cout << fixed << setprecision(2); showValues(numbers, ARRAY_SIZE); cout << "The greatest value from the random numbers is, " << maximum(numbers, ARRAY_SIZE) << endl; cout << "The samllest value from the random numbers is, " << minimum(numbers, ARRAY_SIZE) << endl; cout << "The average from the random numbers is, " << average(numbers, ARRAY_SIZE) << endl; return 0; } void showValues(const int nums[], int size) { for (int index = 0; index < size; index++) cout << nums[index] << " "; cout << endl; // Use a loop to display all elements in the array } // This function will fill out the nums array with random values between low and high void fillArray(int nums[], int size, int low, int high) { for (int i = 0; i < size; i++) nums[i] = rand() % (high - low) + low; // Use a loop to fill out each element of the array between low to high } int maximum(const int nums[], int size) { int max = nums[0]; for (int i = 1; i < size; i++) if (nums[i] > max) max = nums[i]; // Use a loop to find the max value of the array return max; } int minimum(const int nums[], int size) { int min = nums[0]; for (int i = 1; i < size; i++) if (nums[i] < min) min = nums[i]; // Use a loop to find the min value of the array return min; } double average(const int nums[], int size) { int sum = 0; for (int i = 0; i < size; i++) sum = sum + nums[i]; // Use a loop to get the sum of the array return (double)sum / size; } C++
// New Function prototypes
void showValues(const int *, int); // display 10 numbers in a line from the array
void fillArray(int *, int, int, int); // fill out random numbers between low and high into the array
bool searchValue(const int *, int, int); // search value from the array
void sortArray( int *, int); //sort the array in an ascending order
void swap(int *, int *); //swap the values
Modify the code below to create 50 random numbers instead of 10 and use the above new function protoypes.
In the output, remove the display of the minimum, maximum and average from the array. Modify the showValues() function to display 10 numbers in a line from the array.
[Part 2]
Add the two functions to sort an array in an ascending order:
void sortArray(int*, int); //ascending order
void swap(int*, int*);
In the sortArray() function called the swap() function to swap the values from two elements in the array.
Code:
#include <iostream>
#include <iomanip>
#include <cstdlib>
#include <ctime>
using namespace std;
// Function prototype
void showValues(const int[], int);
void fillArray(int[], int, int, int);
int maximum(const int[], int);
int minimum(const int[], int);
double average(const int[], int);
int main()
{
const int ARRAY_SIZE = 10;
int numbers[ARRAY_SIZE];
srand(time(0));
int low = 0, high = 1;
cout << "**This program will generate 10 random integers between [low] and [high]**" << endl;
// prompt to get the user input for the low and high
do
{
cout << "Please enter an integer value for the value for lower bound: ";
cin >> low;
cout << "Please enter an integer value for the value for higher bound: ";
cin >> high;
if (low > high) cout << "The lower bound should be smaller than the higher bound! \n";
}
while (low > high);
// ....
// fill out the random numbers in the array
fillArray(numbers, ARRAY_SIZE, low, high);
cout << "\nThe 10 random integers between " << low << " and " << high << " are:" << endl;
cout << fixed << setprecision(2);
showValues(numbers, ARRAY_SIZE);
cout << "The greatest value from the random numbers is, " << maximum(numbers, ARRAY_SIZE) << endl;
cout << "The samllest value from the random numbers is, " << minimum(numbers, ARRAY_SIZE) << endl;
cout << "The average from the random numbers is, " << average(numbers, ARRAY_SIZE) << endl;
return 0;
}
void showValues(const int nums[], int size)
{
for (int index = 0; index < size; index++)
cout << nums[index] << " ";
cout << endl;
// Use a loop to display all elements in the array
}
// This function will fill out the nums array with random values between low and high
void fillArray(int nums[], int size, int low, int high)
{
for (int i = 0; i < size; i++)
nums[i] = rand() % (high - low) + low;
// Use a loop to fill out each element of the array between low to high
}
int maximum(const int nums[], int size)
{
int max = nums[0];
for (int i = 1; i < size; i++)
if (nums[i] > max)
max = nums[i];
// Use a loop to find the max value of the array
return max;
}
int minimum(const int nums[], int size)
{
int min = nums[0];
for (int i = 1; i < size; i++)
if (nums[i] < min)
min = nums[i];
// Use a loop to find the min value of the array
return min;
}
double average(const int nums[], int size)
{
int sum = 0;
for (int i = 0; i < size; i++)
sum = sum + nums[i];
// Use a loop to get the sum of the array
return (double)sum / size;
}
C++
![**This program will generate 50 random integers between [low] and [high]**
Please enter an integer value for the lower bound: 100
Please enter an integer value for the higher bound: 200
Please enter an value betwen 100 and 200 to search: 150
The 50 random integers betwen 100 and 200 are:
149 173 159 114 143 171 188 164 134 104
133 120 114 101 149 198 194 118 177
131
183
137 127 199
164 149 173 196 124 141
117 132 176 128 127 130 101 127 135 173
150 128 148 139 102 158 105 187 122 180
150 is found from the random numbers!
Display the 50 random integers after sorting:
101 101 102 104 105
114 114 117 118 120
122 124 127 127 127
128 128 130 131
132
133 134 135 137 139
141 143 148 149 149
149
150
177
164
187
158
159
164
171
173 173 173
176
180
183
188
194
196
198
199](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8e7c4d7d-ede9-4b81-8e82-325ff8e9546c%2F7082bc6a-3bf8-4205-871f-2b0d65fe044a%2Fk8u47tg_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

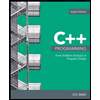
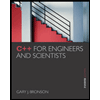
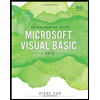
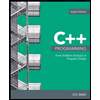
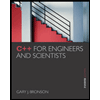
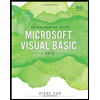
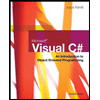