Need to make a flowchart diagram
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Need to make a flowchart diagram
![The image contains Python code that deals with comparing two lists of letters and categorizing their combinations. Here's how the code is structured:
```python
list1 = ['A', 'B', 'C', 'E', 'J']
list2 = ['A', 'A', 'C', 'D', 'B', 'E', 'Z', 'B', 'A', 'F', 'B', 'H', 'A']
letter_combinations = []
repeated_combinations = []
def combination():
for i in list1:
for j in list2:
if (i, j) in letter_combinations:
repeated_combinations.append((i, j))
else:
letter_combinations.append((i, j))
return letter_combinations
def repeats():
for i in list1:
for j in list2:
if ((i, j) in letter_combinations and (i, j) not in repeated_combinations):
repeated_combinations.append((i, j))
elif (i, j) not in letter_combinations:
letter_combinations.append((i, j))
return repeated_combinations
print("letter_combinations:")
print(combination())
print("repeated_combinations:")
print(repeats())
```
### Explanation:
- **Variables:**
- `list1` and `list2` are predefined lists containing letters.
- `letter_combinations` is a list meant to store unique combinations of letters from `list1` and `list2`.
- `repeated_combinations` is a list to store combinations that appear more than once.
- **Functions:**
- `combination()`:
- Iterates over every pairing of elements from `list1` and `list2`.
- If the pair `(i, j)` is already in `letter_combinations`, it's added to `repeated_combinations`. Otherwise, it's added to `letter_combinations`.
- Returns `letter_combinations`.
- `repeats()`:
- Similar loop over elements from `list1` and `list2`.
- Checks if the pair `(i, j)` exists in `letter_combinations` but not in `repeated_combinations`, then adds it to `repeated_combinations`.
- If not in `letter_combinations`, adds it.
- Returns `repeated_combinations`.
- **Output:**
-](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F48fa6f50-a510-4234-9950-4191a13ca9b5%2F2c4ca3d3-2ed9-4b06-a3b7-ee9b367c5304%2Fhb3s5s8_processed.jpeg&w=3840&q=75)
Transcribed Image Text:The image contains Python code that deals with comparing two lists of letters and categorizing their combinations. Here's how the code is structured:
```python
list1 = ['A', 'B', 'C', 'E', 'J']
list2 = ['A', 'A', 'C', 'D', 'B', 'E', 'Z', 'B', 'A', 'F', 'B', 'H', 'A']
letter_combinations = []
repeated_combinations = []
def combination():
for i in list1:
for j in list2:
if (i, j) in letter_combinations:
repeated_combinations.append((i, j))
else:
letter_combinations.append((i, j))
return letter_combinations
def repeats():
for i in list1:
for j in list2:
if ((i, j) in letter_combinations and (i, j) not in repeated_combinations):
repeated_combinations.append((i, j))
elif (i, j) not in letter_combinations:
letter_combinations.append((i, j))
return repeated_combinations
print("letter_combinations:")
print(combination())
print("repeated_combinations:")
print(repeats())
```
### Explanation:
- **Variables:**
- `list1` and `list2` are predefined lists containing letters.
- `letter_combinations` is a list meant to store unique combinations of letters from `list1` and `list2`.
- `repeated_combinations` is a list to store combinations that appear more than once.
- **Functions:**
- `combination()`:
- Iterates over every pairing of elements from `list1` and `list2`.
- If the pair `(i, j)` is already in `letter_combinations`, it's added to `repeated_combinations`. Otherwise, it's added to `letter_combinations`.
- Returns `letter_combinations`.
- `repeats()`:
- Similar loop over elements from `list1` and `list2`.
- Checks if the pair `(i, j)` exists in `letter_combinations` but not in `repeated_combinations`, then adds it to `repeated_combinations`.
- If not in `letter_combinations`, adds it.
- Returns `repeated_combinations`.
- **Output:**
-
![Read two lists, list1 and list2, containing letters.
Declare lists: `letter_combinations` and `repeated_combinations` to hold data elements.
Loop `i = 0` to the length of `list1`
Loop `j = 0` to the length of `list2`
If `(list1[i], list2[j])` exists in `letter_combinations` then,
Append `(list1[i], list2[j])` to `repeated_combinations`
Otherwise,
Append `(list1[i], list2[j])` to `letter_combinations`
End if
End j
End i
Print the lists `letter_combinations` and `repeated_combinations` using a proper method.
End](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F48fa6f50-a510-4234-9950-4191a13ca9b5%2F2c4ca3d3-2ed9-4b06-a3b7-ee9b367c5304%2F4srkzrm_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Read two lists, list1 and list2, containing letters.
Declare lists: `letter_combinations` and `repeated_combinations` to hold data elements.
Loop `i = 0` to the length of `list1`
Loop `j = 0` to the length of `list2`
If `(list1[i], list2[j])` exists in `letter_combinations` then,
Append `(list1[i], list2[j])` to `repeated_combinations`
Otherwise,
Append `(list1[i], list2[j])` to `letter_combinations`
End if
End j
End i
Print the lists `letter_combinations` and `repeated_combinations` using a proper method.
End
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
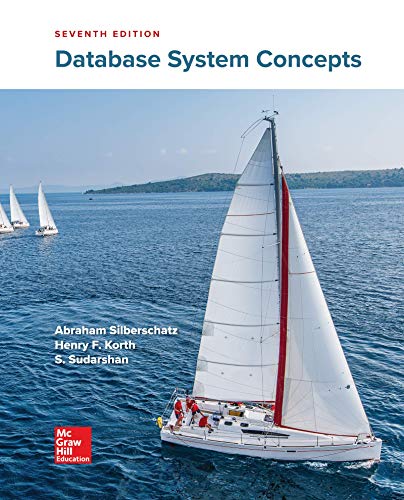
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
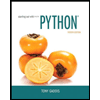
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
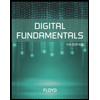
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
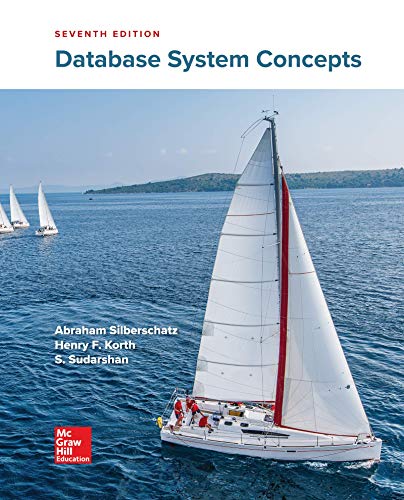
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
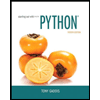
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
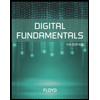
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
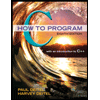
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
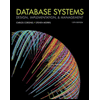
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
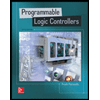
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education