Need help correcting the 24-hour clock as its showing as regular time! #include #include #pragma warning(disable : 4996) using namespace std; time_t now = time(0); tm* local = localtime(&now); int hour = local->tm_hour; int minutes = local->tm_min; int sec = local->tm_sec; // Function to display the 12-hour clock void Clock12() { // Converts 24-hour clock to 12-hour if (hour > 12) { hour -= 12; } cout << "*****************************" << " " << "*****************************\n"; cout << "* 12-Hour Clock *" << " " << "* 24-Hour Clock *\n"; cout << "* " << (hour < 10 ? "0" : "") << hour << ":" << (minutes < 10 ? "0" : "") << minutes << ":" << (sec < 10 ? "0" : "") << sec << (local->tm_hour < 12 ? " AM" : " PM") << " * "; } // Function to display the 24-hour clock void Clock24() { cout << "* " << (hour < 10 ? "0" : "") << hour << ":" << (minutes < 10 ? "0" : "") << minutes << ":" << (sec < 10 ? "0" : "") << sec << " * \n"; cout << "*****************************" << " " << "*****************************\n"; } // Function to add one hour to the clock void AddOneHour() { hour++; if (hour > 23) { hour = 0; } } // Function to add one minute to the clock void AddOneMinute() { minutes++; if (minutes > 59) { minutes = 0; hour++; } if (hour > 23) { hour = 0; } } // Function to add one second to the clock void AddOneSecond() { sec++; if (sec > 59) { sec = 0; minutes++; } if (minutes > 59) { minutes = 0; hour++; } if (hour > 23) { hour = 0; } } int main() { int choice; while (true) { Clock12(); Clock24(); cout << "\n1-Add One Hour" << endl; cout << "2-Add One minute" << endl; cout << "3-Add One Second" << endl; cout << "4-Exit Program" << endl; cin >> choice; switch (choice) { case 1: AddOneHour(); break; case 2: AddOneMinute(); break; case 3: AddOneSecond(); break; case 4: return 0; } } }
Need help correcting the 24-hour clock as its showing as regular time!
#include <iostream>
#include <ctime>
#pragma warning(disable : 4996)
using namespace std;
time_t now = time(0);
tm* local = localtime(&now);
int hour = local->tm_hour;
int minutes = local->tm_min;
int sec = local->tm_sec;
// Function to display the 12-hour clock
void Clock12() {
// Converts 24-hour clock to 12-hour
if (hour > 12) {
hour -= 12;
}
cout << "*****************************" << " " << "*****************************\n";
cout << "* 12-Hour Clock *" << " " << "* 24-Hour Clock *\n";
cout << "* " << (hour < 10 ? "0" : "") << hour << ":" << (minutes < 10 ? "0" : "") << minutes << ":" << (sec < 10 ? "0" : "") << sec << (local->tm_hour < 12 ? " AM" : " PM") << " * ";
}
// Function to display the 24-hour clock
void Clock24() {
cout << "* " << (hour < 10 ? "0" : "") << hour << ":" << (minutes < 10 ? "0" : "") << minutes << ":" << (sec < 10 ? "0" : "") << sec << " * \n";
cout << "*****************************" << " " << "*****************************\n";
}
// Function to add one hour to the clock
void AddOneHour() {
hour++;
if (hour > 23) {
hour = 0;
}
}
// Function to add one minute to the clock
void AddOneMinute() {
minutes++;
if (minutes > 59) {
minutes = 0;
hour++;
}
if (hour > 23) {
hour = 0;
}
}
// Function to add one second to the clock
void AddOneSecond() {
sec++;
if (sec > 59) {
sec = 0;
minutes++;
}
if (minutes > 59) {
minutes = 0;
hour++;
}
if (hour > 23) {
hour = 0;
}
}
int main() {
int choice;
while (true) {
Clock12();
Clock24();
cout << "\n1-Add One Hour" << endl;
cout << "2-Add One minute" << endl;
cout << "3-Add One Second" << endl;
cout << "4-Exit Program" << endl;
cin >> choice;
switch (choice) {
case 1:
AddOneHour();
break;
case 2:
AddOneMinute();
break;
case 3:
AddOneSecond();
break;
case 4:
return 0;
}
}
}

Step by step
Solved in 4 steps with 3 images

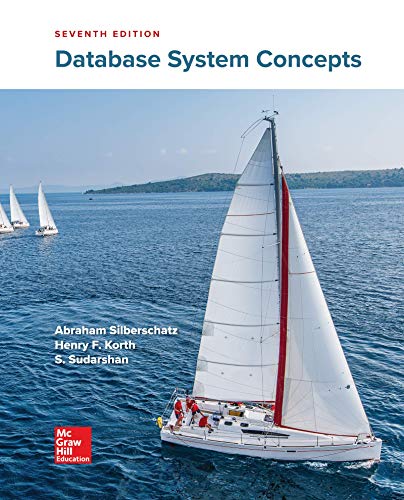
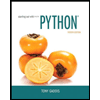
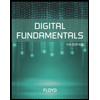
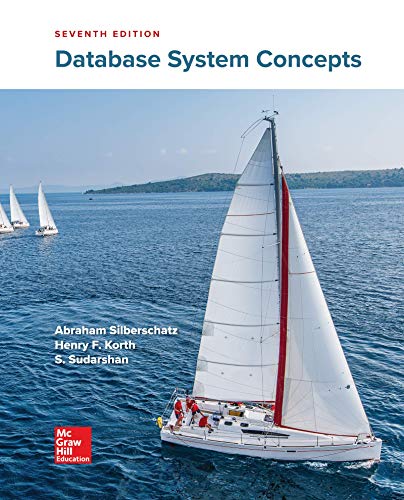
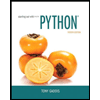
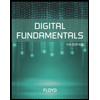
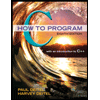
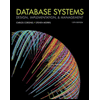
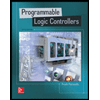