Need assistance in determining what went wrong with my code and why I cannot seem to fix all of the issues. I am using visual studio code. I need to have my python code corrected as soon as possible and learn where my errors are. #The main function def main(): endProgram = "no" endOrder = "no" totalBurger = 0.0 totalFry = 0.0 totalSoda = 0.0 total = 0.0 tax = 0.0 subtotal = 0.0 option = 0 burgerCount = 0 fryCount = 0 sodaCount = 0 # Reset Variables while ("endProgram" == "no"): # reset variables totalburger, totalFry, totalSoda, total, tax, subtotal totalBurger = 0.0 totalFry = 0.0 totalSOda = 0.0 total = 0.0 tax = 0.0 subtotal = 0.0 endOrder = "no" # Loop that takes meal order while ("endOrder" == "no"): print ("Enter 1 for Yum Yum Burger") print ("Enter 2 for Grease Yum Fries") print ("Enter 3 for Soda Yum") option = int(input("Enter options: ")) if (option == 1): totalBurger = "getBurger"(totalBurger, burgerCount) elif (option == 2): totalFry = getFry(totalFry, fryCount) elif (option == 3): totalSoda = getSoda(totalSoda, sodaCount) endOrder = input("Do you want to end your order? (Enter no to process a new order)") total = "calcTotal" ("totalBurger, totalFry, totalSoda, total, subtotal, tax") printReceipt(total) endProgram = input ("do you want to end the progress?(enter no to process a new order)") def getBurger (totalBurger, burgerCount): burgerCount = int(input("how many burgers do you want? 0 if none")) totalBurger = totalBurger + (burgerCount * 0.99) returntotalBurger def getFry(totalFry, fryCount): fryCount = int(input("How many fries do you want? (0 if none) ")) totalFry = totalFry + (fryCount * 0.79) returntotalFry def getSoda(totalSoda, sodaCount): sodaCount = int(input("How many sodas do you want? (0 if none) ")) totalSoda = totalSoda + (sodaCount * 1.09) returntotalSOda def calcTotal(totalBurger, totalFry, totalSoda, total, subtotal, tax): tax = subtotal * 0.06 total = subtotal + tax return total def printReciept (total): print ("your total is $ {0:.2f}".format(total)) # calls main main()
Addition of Two Numbers
Adding two numbers in programming is essentially the same as adding two numbers in general arithmetic. A significant difference is that in programming, you need to pay attention to the data type of the variable that will hold the sum of two numbers.
C++
C++ is a general-purpose hybrid language, which supports both OOPs and procedural language designed and developed by Bjarne Stroustrup. It began in 1979 as “C with Classes” at Bell Labs and first appeared in the year 1985 as C++. It is the superset of C programming language, because it uses most of the C code syntax. Due to its hybrid functionality, it used to develop embedded systems, operating systems, web browser, GUI and video games.
Need assistance in determining what went wrong with my code and why I cannot seem to fix all of the issues. I am using visual studio code. I need to have my python code corrected as soon as possible and learn where my errors are.

While writing codes in python, we must always take care of indentation.
Indentation is very important in python.
I have highlighted all the changes done in the code.
I have also attached images of the code.
Errors made in the code:
- improper indentation
- the code does not calculate sub total and hence, the end result is wrong
- while writing the condition in while loop, do NOT write the variable name within double quotes. Write only the string within ("") double quotes.
For example: while ("endOrder" == "no"): is WRONG
CORRECT: while (endOrder == "no"): - while calling the printReciept (total) function, the spelling was wrong.
Rest everything is correct.
Make sure you properly indent your code as per your requirement.
Step by step
Solved in 4 steps with 5 images

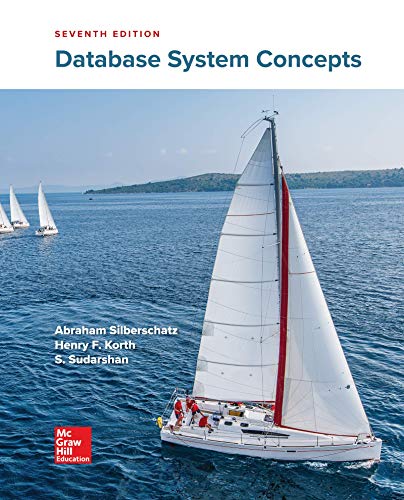
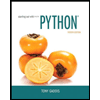
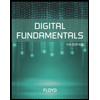
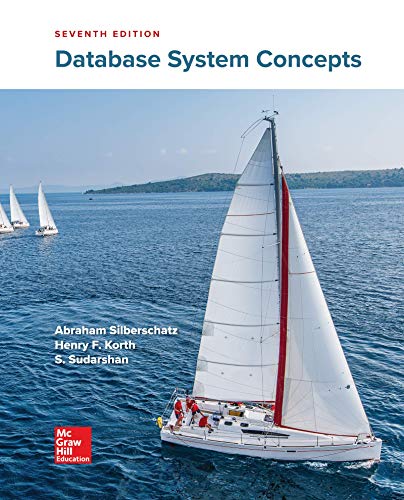
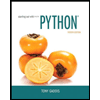
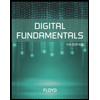
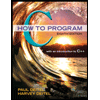
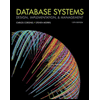
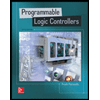