nction of a the C code of this, some instructions are in the images. Input 1. The number of commands 2. The initial count of elements in the array 3. A series of commands with their corresponding inputs needed Description If the command number is: - 1, the succeeding inputs are the elements of the array. - 2, there are no succeeding inputs - 3, the succeeding input is the element to be searched - 4, the succeeding input is the element to be searched - 5, there are no succeeding inputs - 6, there are no succeeding inputs - 7, the succeeding inputs are the element to be inserted and the index where the element will be inserted - 8, the succeeding input is the element to be inserted - 9, the succeeding input is the index to be removed - 10, the succeeding input is the element to be removed - 11, there are no succeeding inputs Output Enter·number·of·commands:·5
I need a function of a the C code of this, some instructions are in the images.
Input
1. The number of commands
2. The initial count of elements in the array
3. A series of commands with their corresponding inputs needed
Description
If the command number is: - 1, the succeeding inputs are the elements of the array. - 2, there are no succeeding inputs - 3, the succeeding input is the element to be searched - 4, the succeeding input is the element to be searched - 5, there are no succeeding inputs - 6, there are no succeeding inputs - 7, the succeeding inputs are the element to be inserted and the index where the element will be inserted - 8, the succeeding input is the element to be inserted - 9, the succeeding input is the index to be removed - 10, the succeeding input is the element to be removed - 11, there are no succeeding inputs
Output
#include<stdio.h>
#include "arrayOptn.h"
#define MAX_SIZE 100
int main(void) {
int array[MAX_SIZE];
int count = 0;
int numberOfCommands;
printf("Enter number of commands: ");
scanf("%d", &numberOfCommands);
printf("Enter count of elements: ");
scanf("%d", &count);
printf("\n");
int command;
int elem, k;
for(int c = 1; c <= numberOfCommands; c++) {
printf("COMMAND #: ");
scanf("%d", &command);
switch(command) {
case 1:
createArray(array, count, MAX_SIZE);
printf("\n");
break;
case 2:
printArray(array, count);
printf("\n\n");
break;
case 3:
printf("Enter element: ");
scanf("%d", &elem);
printf("Return Value: %d", locateIndex(array, count, elem));
printf("\n\n");
break;
case 4:
printf("Enter element: ");
scanf("%d", &elem);
printf("Return Value: %d", locateElement(array, count, elem));
printf("\n\n");
break;
case 5:
printElementsInAscending(array, count);
printf("\n\n");
break;
case 6:
printElementsInDescending(array, count);
printf("\n\n");
break;
case 7:
printf("Enter element: ");
scanf("%d", &elem);
printf("Enter position: ");
scanf("%d", &k);
printf("Return Value: %d", insertAtPos(array, &count, elem, k));
printf("\n\n");
break;
case 8:
printf("Enter element: ");
scanf("%d", &elem);
printf("Return Value: %d", insertFront(array, &count, elem));
printf("\n\n");
break;
case 9:
printf("Enter position: ");
scanf("%d", &k);
printf("Return Value: %d", removeAtPos(array, &count, k));
printf("\n\n");
break;
case 10:
printf("Enter element: ");
scanf("%d", &elem);
printf("Return Value: %d", removeElement(array, &count, elem));
printf("\n\n");
break;
case 11:
printf("Return Value: %d", removeFront(array, &count));
printf("\n\n");
}
}
return 0;
}
![Checks if the
List of functions to be
implemented:
2 - void printArray(int
arr[], int count)
Parameters:
int arr[] - a reference
to the array whose
elements are to be
4 - int
locateElement(int
arr[], int count, int
elem)
Parameters:
3 - int
5 - void
printElementslnAsce
nding(int arr[], int
count)
Parameters:
6 - void
printElementslnDesc
ending(int arr[], int
count)
Paramters:
7- int insertAtPos(int arr), int
*count, int elem, int k)
Parameters:
int arr) - a reference to the array
locatelndex(int arr[),
int count, int elem)
Parameters:
8- int insertFront(int
arr), int *count, int
elem)
1 - void createArray(int
arr[], int count, int
maxCapacity)
Parameters:
int arr[] - a reference to
an array
Parameters:
int arr[] - a reference
to the array where
we will search the
where we insert the element
int arrl - a reference to
int arr[] - a reference int arr[] - a reference
int *count - a pointer to the current
number of elements in the array
the array where we
Int arr] - a reference
to the array to be
processed
int count - the
int
to the array where
we will search the
to the array to be
processed
int count - the
number of elements
printed
insert the element
int elem - the element to be
int count - the
number of elements
element
int *count - a pointer to
inserted
int k- the index position where the
int count - the
element
the current number of
elements in the array
In the array
Description:
Prints all the
elements of the
int count - the number
number of elements
in the array
int elem - the
int count - the
number of elements
in the array
Description:
Prints all the
elements of the
element will be inserted
int elem - the element
to be inserted
of elements to be
inputted by the user and
to be stored in the array
int maxCapacity - the
max capacity of the
array
number of elements in the array
Description:
Prints all the
elements of the
in the array
int elem - the
element to be
Description:
Inserts the element at the kth index Description:
element to be
of the array and moves all the
elements to the right starting at the index 0 of the array and
Inserts the element at
array starting from
Index 0 up to index
count - 1
The format of the
searched
Description:
Finds the index of
searched
passed array in
kth position. For example, if k is 3,
ascending order
The format of the
passed array in
descending order
The format of the
moves all of the
elements to the right.
Before inserting the
element at index 4 will be moved to element, check first if it
Description:
the current element at index 3 will
Description:
the element in the
be moved to index 4, the current
Asks the user for the
elements of the array.
The prompt message is:
"Enter element at index
message is:
"Elements: el1 el2
el3 elx" where e1,
array
Return Value:
element is in the
message is:
"Elements: el1 el2
message is:
"Elements: el1 el2
array
Return Value:
index 5, the current element at
index 5 will be moved to index 6,
and so on. Then, the element to be already, print the
does not yet exist in the
array. If it does exist
f the element is
el3 elx" where e1,
el2. el3, elx are the
el3 elx" where e1,
found, this returns
the index of the
element. Otherwise,
el2, el3, elx are the
If the element is
el2, el3, elx are the
inserted will be placed at index k.
Before inserting the element,
check first if it does not yet exist in
the array. If it does exist already,
print the message "Data element
you wish to add already exists in
the array. Try another!"
After inserting the element,
increase the count of the array
through the pointer passed
Hint: You may use the
locateElement() function to check if function to check if the
N: "where N is the
current elements of
found, this returns 1 current elements of
message "Data
element you wish to
add already exists in
the array. Try another!"
After inserting the
element, increase the
count of the array
through the pointer
current elements of
the array.
current index position
the array.
to represent true.
Otherwise, this
the array.
being scanned.
Each inputted integer
must be unique. If an
element already exists
in the array, you prompt
the user the message
"Inputted element
already exists, try
another number"
this returns -1.
returns 0 to
represent false.
passed
Hint: You may use the
locateElement()
If the count is less than
the element already exists
element already exists
Return Value:
int - 1 if the insertion
the maxCapacity, set
the values of all the
remaining indices of the
array to 0.
Return Value:
int - 1 if the insertion was
successful and 0 if not.
was successful and 0
if not.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9490f4b0-0337-4e00-bf69-d25dd1e500d1%2F0c243eb5-b4ee-4750-a06a-db9eec6f308c%2Fgwtl3ut_processed.jpeg&w=3840&q=75)
![9 - int removeAtPos(int arr),
int *count, int k)
Parameters:
int arr - a reference to the
array where we remove an
element
int *count -a pointer to the
10 - int removeElement(int arr[], int
*count, int elem)
Parameters:
int arr] - a reference to the array
where we remove an element
11 - int removeFront(int
arr], int *count)
Parameters:
int arr[] - a reference to
the array where we
remove an element
int *count - a pointer to the current
number of elements in the array
int *count - a pointer to
the current number of
elements in the array
Description:
Removes the element
at index 0 by moving all
the elements to the left
starting at index 1.
After removing the
element, decrement
the count of the array
through the pointer
passed
If the passed element
does not exist, print the
message"There is no
such element in the
current number of elements in
int elem - the element to be
the array
int k- the index position where
removed
Description:
Finds the element in the array and
we remove an element
Description:
Removes the element at the
kth index of the array by
moving all the elements to the
left starting at the k + 1
position. For example, if k is 3,
removes it by moving all the
elements to the left starting at the k
+ 1 position where k is the index of
the po. For example, if k is 3, the
current element at index 4 will be
the current element at index 4
will be moved to index 3, the
current element at index 5 will
be moved to index 4, the
current element at index 6 will
be moved to index 5, and so
moved to index 3, the current
element at index 5 will be moved to
index 4, the current element at
index 6 will be moved to index 5,
and so on.
After removing the element,
decrement the count of the array
through the pointer passed
If the passed element does not
exist, print the message"There is
no such element in the array. Try
another!"
on.
After removing the element,
decrement the count of the
array through the pointer
passed
If the passed index k is
greater than or equal to the
array. Try another!"
Return Value:
int - 1 if the removal
was successful and 0 if
array's current count of
elements, print the message
"There is no element in that
not.
Return Value:
int - 1 if the removal was
index. Try another!"
Return Value:
int - 1 if the removal was
successful and 0 if not.
successful and 0 if not.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9490f4b0-0337-4e00-bf69-d25dd1e500d1%2F0c243eb5-b4ee-4750-a06a-db9eec6f308c%2Flgpy9im_processed.jpeg&w=3840&q=75)

Step by step
Solved in 4 steps with 3 images

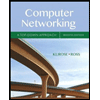
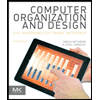
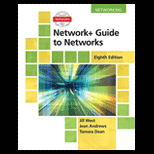
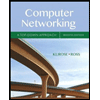
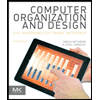
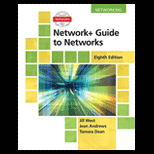
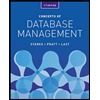
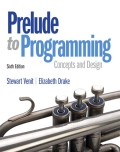
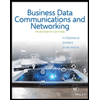