n the language of an alien race, all words take the form of Blurbs. A Blurb is a Whoozit followed by between zero or more Whatzits. A Whoozit is the character 'x' followed by between one or more 'y's. A Whatzit is a 'q' followed by either a 'z' or a 'd', followed by a Whoozit. -The program should ask the user to “Enter an alien word:”, read the input as a string, and check whether or not it is a valid word (Blurb). If it is valid, it prints "The word is fine.", otherwise it prints "The word is a mess!". -The program should loop and ask the user for new input until they enter a valid blurb. For Scheme, it is suggested that you review the “input_and_output.rkt” sample program from the first module on functional programming. This program shows how to perform a “loop” that reads in data and processes it. You may find the following forms useful: a. (read) to read a string b. (string->list) to convert the string into a list of characters, c. (display “Hello”) to write Hello to the screen. d. (begin) to help with displaying an output while not breaking out of the running procedure.
USE SCHEME IN DRRACKET.
In the language of an alien race, all words take the form of Blurbs. A Blurb is a Whoozit
followed by between zero or more Whatzits. A Whoozit is the character 'x' followed by
between one or more 'y's. A Whatzit is a 'q' followed by either a 'z' or a 'd', followed by a
Whoozit.
-The program should ask the user to “Enter an alien word:”, read the input
as a string, and check whether or not it is a valid word (Blurb). If it is valid, it prints
"The word is fine.", otherwise it prints "The word is a mess!".
-The program should loop and ask the user for new input until they enter a
valid blurb.
For Scheme, it is suggested that you review the “input_and_output.rkt” sample
program from the first module on functional
to perform a “loop” that reads in data and processes it. You may find the following
forms useful:
a. (read) to read a string
b. (string->list) to convert the string into a list of characters,
c. (display “Hello”) to write Hello to the screen.
d. (begin) to help with displaying an output while not breaking out of the running
procedure.


Let us see the answer:-
Introduction:-
(define (valid-blurb? word)
(cond ((< (string-length word) 2) #f)
((not (eq? (string-ref word 0) #\x)) #f)
(else (let loop ((i 1))
(cond ((>= i (string-length word)) #t)
((eq? (string-ref word i) #\y)
(loop (+ i 1)))
((and (eq? (string-ref word i) #\q)
(eq? (string-ref word (+ i 1)) #\z))
(loop (+ i 3)))
((and (eq? (string-ref word i) #\q)
(eq? (string-ref word (+ i 1)) #\d))
(loop (+ i 3)))
(else #f))))))
(define (blurb-check-loop)
(display "Enter an alien word: ")
(let ((word (string-trim (read))))
(if (valid-blurb? word)
(begin (display "The word is fine.\n")
#t)
(begin (display "The word is a mess!\n")
(blurb-check-loop)))))
(blurb-check-loop)
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

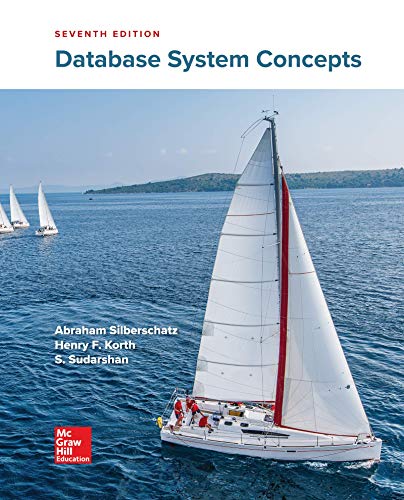
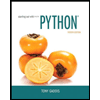
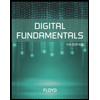
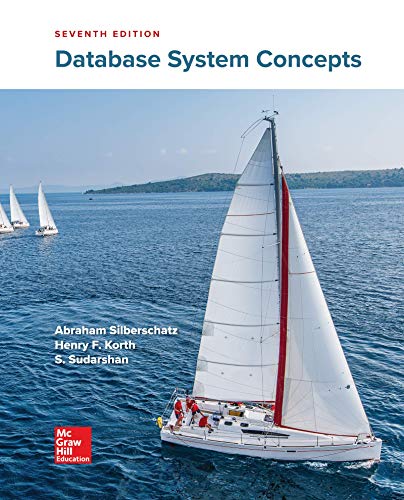
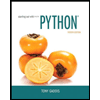
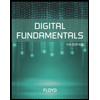
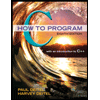
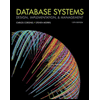
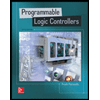