My issue: I wrote my questions in bold in the comments of the code Java code: import java.util.Scanner; public class DescendingOrder { // TODO: Write a void method selectionSortDescendTrace() that takes // an integer array and the number of elements in the array as arguments, // and sorts the array into descending order. public static void selectionSortDescendTrace(int [] numbers, int numElements) { //my code starts here int i; int j; int elementIndex; int temp; //loop in ascending order using textbook example for (i=0; i < numElements-1; ++i) { index=i; for (j=i+1; j < numElements; ++j) { if (numbers[j] < numbers[elementIndex]) { elementIndex=j; } } //swap using textbook example temp=numbers[i]; numbers[i]=numbers[elementIndex]; numbers[elementIndex]=temp; //I don't know how to put it in descending order. Is it using a loop? //where do I put in descending order? Is it in the method definitions or main method ??? } } //my code ends here public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int input, i = 0; int numElements = 0; int [] numbers = new int[10]; // TODO: Read in a list of up to 10 positive integers; stop when // -1 is read. Then call selectionSortDescendTrace() method. //my code starts here for (i=0; i < numbers.length; ++i) { input=scnr.nextInt(); numbers[i]=input; if (numbers[i]==-1) { break; numElements++; } //selectionSortDescendTrace(); //what goes inside the parenthesis??? //my code ends here } }
My issue:
I wrote my questions in bold in the comments of the code
Java code:
import java.util.Scanner;
public class DescendingOrder {
// TODO: Write a void method selectionSortDescendTrace() that takes
// an integer array and the number of elements in the array as arguments,
// and sorts the array into descending order.
public static void selectionSortDescendTrace(int [] numbers, int numElements) {
//my code starts here
int i;
int j;
int elementIndex;
int temp;
//loop in ascending order using textbook example
for (i=0; i < numElements-1; ++i) {
index=i;
for (j=i+1; j < numElements; ++j) {
if (numbers[j] < numbers[elementIndex]) {
elementIndex=j;
}
}
//swap using textbook example
temp=numbers[i];
numbers[i]=numbers[elementIndex];
numbers[elementIndex]=temp;
//I don't know how to put it in descending order. Is it using a loop?
//where do I put in descending order? Is it in the method definitions or main method ???
}
}
//my code ends here
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int input, i = 0;
int numElements = 0;
int [] numbers = new int[10];
// TODO: Read in a list of up to 10 positive integers; stop when
// -1 is read. Then call selectionSortDescendTrace() method.
//my code starts here
for (i=0; i < numbers.length; ++i) {
input=scnr.nextInt();
numbers[i]=input;
if (numbers[i]==-1) {
break;
numElements++;
}
//selectionSortDescendTrace(); //what goes inside the parenthesis???
//my code ends here
}
}

![Selection sort has the advantage of being easy to code, involving one loop nested within another loop, as shown below.
Figure 8.6.1: Selection sort algorithm.
public class SelectionSort {
public static void selectionSort (int [] numbers) {
int i;
int j;
int indexSmallest;
int temp;
// Temporary variable for swap
for (i = e; i« numbers.length
1; ++i) {
// Find index of smallest remaining element
indexSmallest = i;
for (j = i + 1; j< numbers.length; ++j) {
if (numbers[j] < numbers [indexSmallest]) {
indexSmallest - j;
}
// Swap numbers[i] and numbers[indexSmallest]
temp = numbers[i];
numbers[i] = numbers[indexSmallest];
numbers[indexSmallest] = temp;
UNSORTED: 10 2 78 4 45 32 7 11
SORTED: 2 4 7 10 11 32 45 78
public static void main(String [] args)
int numbers [] = {10, 2, 78, 4, 45, 32, 7, 11};
int i;
System.out.print ("UNSORTED: ");
for (i = e; i < numbers.length; ++i)
System.out.print (numbers[i] +
System.out
In()3B
/* initial call to selection sort with index */
selectionSort(numbers);
System.out.print("SORTED: ");
for (i = e; i < numbers.length; ++i) {
System.out.print (numbers[i] + " ");
System.out.println();
Feedback?
Selection sort may require a large number of comparisons. The selection sort algorithm runtime is O(N2). If a list has N elements, the outer
loop executes N -1 times. For each of those N -1 outer loop executions, the inner loop executes an average of * times. So the total](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc14768d3-3359-407d-85b4-624ecac227c9%2F92779156-44f9-4ce0-990d-4585e12ab813%2Fwm1css_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

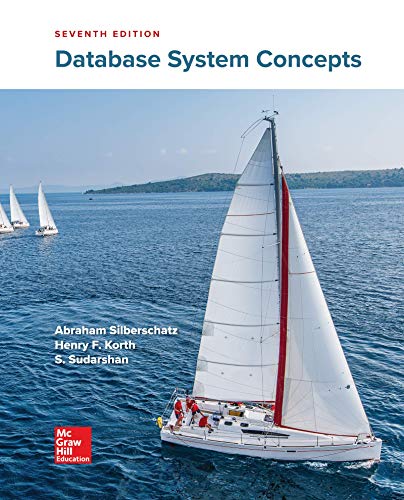
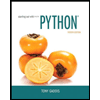
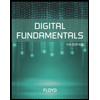
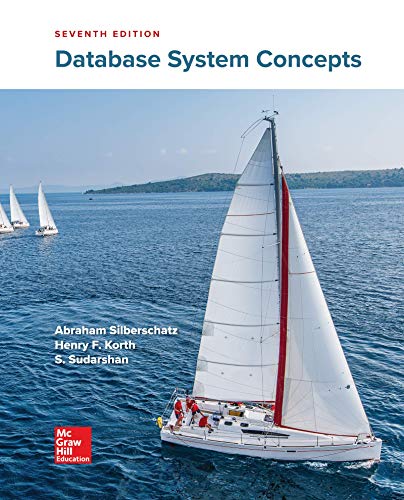
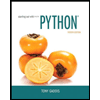
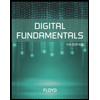
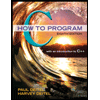
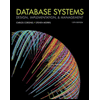
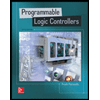