mplement the 4 methods marked ''// Your Code' in the OurListSet class. public boolean addAll(OurSet set) public boolean containsAll(OurSet set) public boolean removeAll(OurSet set) public boolean retainAll(OurSet set) (note: this was written in BlueJ) Here is the full code for OutListSet.java: import java.util.Iterator; /** * Write a description of class OurListSet here. * * @author (your name) * @version (a version number or a date) */ public class OurListSet implements OurSet { private OurList list; /** * Constructor for objects of class OurListSet */ public OurListSet() { this(new OurArrayList()); } // Designated Constructor public OurListSet(OurList newList) { list = newList; } private class OurListIterator implements Iterator { private OurList listToIterateOver; private int position; public OurListIterator(OurList list) { listToIterateOver = list; position = 0; } public boolean hasNext() { return position < listToIterateOver.size(); } public E next() { E elementToReturn = listToIterateOver.get(position); position++; return elementToReturn; } } public boolean add(E obj) { if(contains(obj)) { return false; } else { return list.add(obj); } } public boolean contains(E obj) { return list.contains(obj); } // Your Code public boolean addAll(OurSet set) { return false; } // Your Code public boolean containsAll(OurSet set) { return false; } // Your Code public boolean removeAll(OurSet set) { return false; } // Your Code public boolean retainAll(OurSet set) { return false; } public boolean isEmpty() { return list.isEmpty(); } public Iterator iterator() { return new OurListIterator(list); } public boolean remove(Object obj) { return list.remove(obj); } public int size() { return list.size(); }
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Implement the 4 methods marked ''// Your Code' in the OurListSet class.
public boolean addAll(OurSet<E> set)
public boolean containsAll(OurSet<E> set)
public boolean removeAll(OurSet<E> set)
public boolean retainAll(OurSet<E> set)
(note: this was written in BlueJ)
Here is the full code for OutListSet.java:
import java.util.Iterator;
/**
* Write a description of class OurListSet here.
*
* @author (your name)
* @version (a version number or a date)
*/
public class OurListSet<E> implements OurSet<E>
{
private OurList<E> list;
/**
* Constructor for objects of class OurListSet
*/
public OurListSet()
{
this(new OurArrayList());
}
// Designated Constructor
public OurListSet(OurList<E> newList)
{
list = newList;
}
private class OurListIterator<E> implements Iterator<E>
{
private OurList<E> listToIterateOver;
private int position;
public OurListIterator(OurList<E> list)
{
listToIterateOver = list;
position = 0;
}
public boolean hasNext()
{
return position < listToIterateOver.size();
}
public E next()
{
E elementToReturn = listToIterateOver.get(position);
position++;
return elementToReturn;
}
}
public boolean add(E obj)
{
if(contains(obj))
{
return false;
}
else
{
return list.add(obj);
}
}
public boolean contains(E obj)
{
return list.contains(obj);
}
// Your Code
public boolean addAll(OurSet<E> set)
{
return false;
}
// Your Code
public boolean containsAll(OurSet<E> set)
{
return false;
}
// Your Code
public boolean removeAll(OurSet<E> set)
{
return false;
}
// Your Code
public boolean retainAll(OurSet<E> set)
{
return false;
}
public boolean isEmpty()
{
return list.isEmpty();
}
public Iterator<E> iterator()
{
return new OurListIterator<E>(list);
}
public boolean remove(Object obj)
{
return list.remove(obj);
}
public int size()
{
return list.size();
}
}

Step by step
Solved in 2 steps

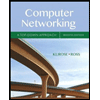
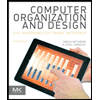
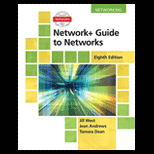
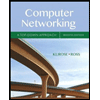
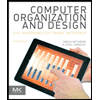
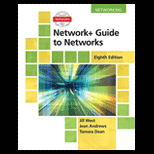
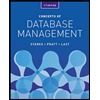
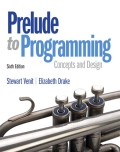
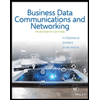