-Modify your parameter constructors to call your set methods. This will cause validation of the parameter. -Create an exception class named TractorException. -Modify your setters to throw TractorException if invalid values are passed in. You may also need to add a throws clause to your parameter constructors. This way we will not have any print methods in our Tractor object defining class -Modify your main method to try and catch exceptions. It should catch TractorExceptions and general Exceptions and display an appropriate message import java.util.*; class TestException { public static void main(String s[]) { Scanner scanner = new Scanner(System.in); boolean valid=false; // validation loop while (!valid) { try { System.out.println("Enter integer:"); int number = scanner.nextInt(); // may throw an exception System.out.println("You entered "+ number); valid=true; } catch(Exception e) { scanner.nextLine(); // clears out the junk from the scanner input System.out.println("ERROR: Must enter a number!!!"); } } } }
-Modify your parameter constructors to call your set
methods. This will cause validation of the parameter.
-Create an exception class named TractorException.
-Modify your setters to throw TractorException if
invalid values are passed in. You may also need to add a
throws clause to your parameter constructors. This way
we will not have any print methods in our Tractor object
defining class
-Modify your main method to try and catch
exceptions. It should catch TractorExceptions and
general Exceptions and display an appropriate message
import java.util.*;
class TestException {
public static void main(String s[]) {
Scanner scanner = new Scanner(System.in);
boolean valid=false;
// validation loop
while (!valid) {
try {
System.out.println("Enter integer:");
int number = scanner.nextInt(); // may throw an exception
System.out.println("You entered "+ number);
valid=true;
} catch(Exception e) {
scanner.nextLine(); // clears out the junk from the scanner input
System.out.println("ERROR: Must enter a number!!!");
}
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

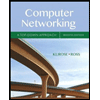
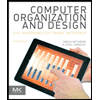
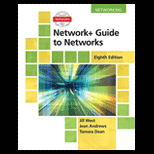
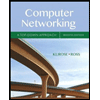
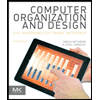
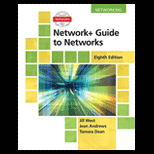
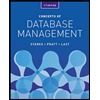
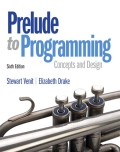
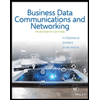