Modify the scripts of Projects 1 and 2 to encrypt and decrypt entire files of text. An example of the program interface is shown below: Enter the input file name: encrypted.txt Enter the output file name: a Enter the distance value: 3 Project 1: text = (input("Please enter your text: ")) shift = int(input("Please enter the shift: ")) def circularShift(text, key): text = text.upper() cipher = "" for letter in text: shifted = ord(letter) + key if shifted < 65: shifted += 0 if shifted > 90: shifted -= 0 cipher += chr(shifted) return cipher print (circularShift(text, shift)) Project 2 text = (input("Please enter your text: ")) shift = int(input("Please enter the shift: ")) convert = -(shift) def cypher(text, key): text = text.capitalize() cipher = "" for letter in text: shifted = ord(letter) + key cipher += chr(shifted) return cipher print (cypher(text, convert))
Modify the scripts of Projects 1 and 2 to encrypt and decrypt entire files of text. An example of the program interface is shown below: Enter the input file name: encrypted.txt Enter the output file name: a Enter the distance value: 3 Project 1: text = (input("Please enter your text: ")) shift = int(input("Please enter the shift: ")) def circularShift(text, key): text = text.upper() cipher = "" for letter in text: shifted = ord(letter) + key if shifted < 65: shifted += 0 if shifted > 90: shifted -= 0 cipher += chr(shifted) return cipher print (circularShift(text, shift)) Project 2 text = (input("Please enter your text: ")) shift = int(input("Please enter the shift: ")) convert = -(shift) def cypher(text, key): text = text.capitalize() cipher = "" for letter in text: shifted = ord(letter) + key cipher += chr(shifted) return cipher print (cypher(text, convert))
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Modify the scripts of Projects 1 and 2 to encrypt and decrypt entire files of text.
An example of the program interface is shown below:
Enter the input file name: encrypted.txt
Enter the output file name: a
Enter the distance value: 3
Project 1:
text = (input("Please enter your text: "))
shift = int(input("Please enter the shift: "))
def circularShift(text, key):
text = text.upper()
cipher = ""
for letter in text:
shifted = ord(letter) + key
if shifted < 65:
shifted += 0
if shifted > 90:
shifted -= 0
cipher += chr(shifted)
return cipher
print (circularShift(text, shift))
Project 2
text = (input("Please enter your text: "))
shift = int(input("Please enter the shift: "))
convert = -(shift)
def cypher(text, key):
text = text.capitalize()
cipher = ""
for letter in text:
shifted = ord(letter) + key
cipher += chr(shifted)
return cipher
print (cypher(text, convert))
Expert Solution

Step 1
Algorithm:
- Start
- Read input and output file names
- Read shift
- Open input file and read the text and store it in text variable
- Implement a method circularShift() which takes text and key as arguments
- Inside the method iterate through the text
- Set shifted = ord(letter) + key
- If letter is upper case, add chr((shifted-65)%26+65) to cipher
- Else if letter is lower case, add chr((shifted-97)%26+97) to cipher
- Else add letter to text
- After completion of iteration, return cipher
- Inside the main method, call circularShift() method and write the returned value to output file
- Stop
Trending now
This is a popular solution!
Step by step
Solved in 8 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
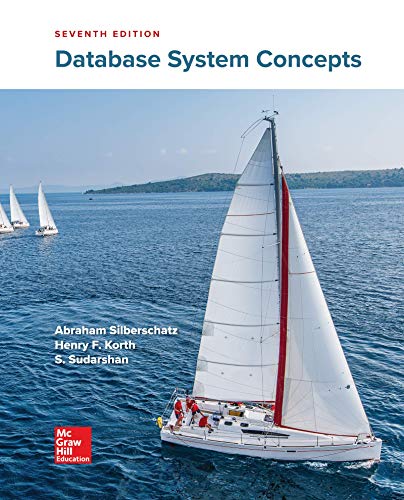
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
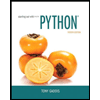
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
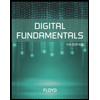
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
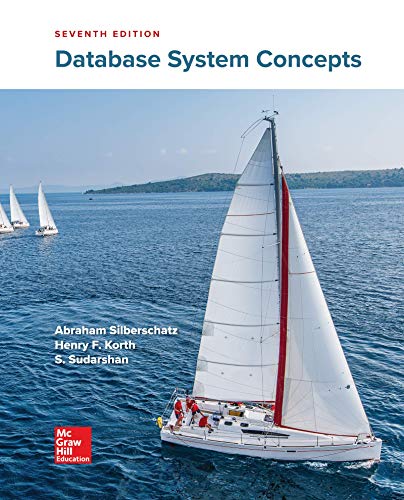
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
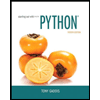
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
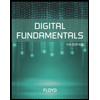
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
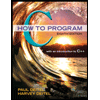
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
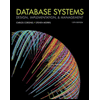
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
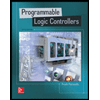
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education