Modify the Loan class definition in the program ex91.cpp to include a new class called Bank in its definition as described below. // This will go to Loan.h file class Loan { public: Loan( ); Loan(Bank bank, ID id, float amount, float rate, int term); void set( ); float payment( ); void display( ); private: Bank bank; ID id; // assume an unique integer in three integer parts float amount; // $ amount of the loan float rate; // annual interest rate int term; // number of months, length of the loan }; The Bank class is defined as: // This will go to Bank.h file class Bank // Bank class definition { public: Bank( ); Bank(int bank_ID, CONTACT phone, CONTACT fax); private: int bank_ID; // 4 digit integer Contact phone; // object three integer pieces, # # #, # # #, # # # # Contact fax; // object three integer pieces, # # #, # # #, # # # # }; The class Contact has three integer parts, for Example: part1: 828, Part2: 666, Part3: 7777. You will define the Loan ADT class in separate files. Note that by adding this class to the ex91.cpp program, you will have 4 additional files, Bank.h, Bank.cpp, Contact.cpp, and Contact.h files
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Modify the Loan class definition in the program ex91.cpp to include a new class called Bank in its definition as described below.
// This will go to Loan.h file
class Loan
{
public:
Loan( );
Loan(Bank bank, ID id, float amount, float rate, int term);
void set( );
float payment( );
void display( );
private:
Bank bank;
ID id; // assume an unique integer in three integer parts
float amount; // $ amount of the loan
float rate; // annual interest rate
int term; // number of months, length of the loan
};
The Bank class is defined as:
// This will go to Bank.h file
class Bank // Bank class definition
{
public:
Bank( );
Bank(int bank_ID, CONTACT phone, CONTACT fax);
private:
int bank_ID; // 4 digit integer
Contact phone; // object three integer pieces, # # #, # # #, # # # #
Contact fax; // object three integer pieces, # # #, # # #, # # # #
};
The class Contact has three integer parts, for Example: part1: 828, Part2: 666, Part3: 7777.
You will define the Loan ADT class in separate files. Note that by adding this class to the ex91.cpp program, you will have 4 additional files, Bank.h, Bank.cpp, Contact.cpp, and Contact.h files.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

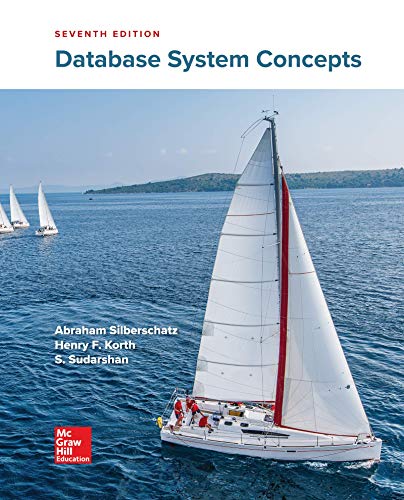
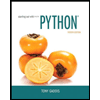
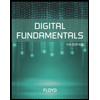
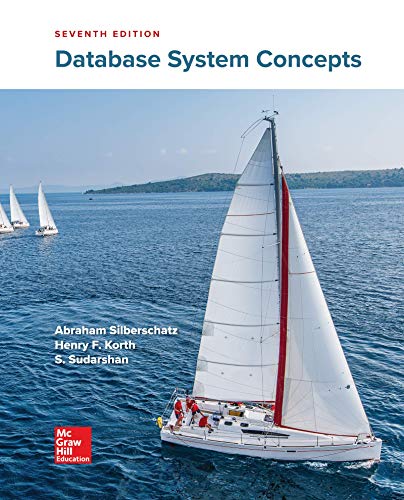
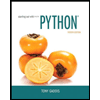
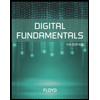
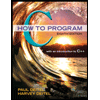
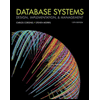
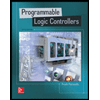