Modify the Figures code below: In constructors, use for parameters the same names as the fields, and use this to access the fields. Make all fields private. Create getter methods to return the values of the fields. Update the main method as needed. For point and rectangle classes create a class variable to keep how many objects were instantiated. Create a gettter to return the number. In the main method show the value after each new element is created. Modify the Bicycle code below: In constructors, use for parameters the same names as the fields, and use this to access the fields. Make all fields private. Create getter methods to return the values of the fields. Update the main method as needed. For the generic and mountain bicycle classes create a class variable to keep how many objects were instantiated. Create a gettter to return the number. In the main method show the value after each new element is created. Bicycle code: interface Bicycle{ //interface for bicycle void changeGear(int val); //abstract methods void changeSpeed(int inc); void applyBrakes(int dec); void ringBell(int count); } class NewCycle implements Bicycle{ //class for a new cycle int speed=0; //stores the speed value int gear=0; //stores the gear value @Override public void changeGear(int val) { //method to change the gear gear=val; } @Override public void changeSpeed(int inc) { //method to change the speed speed=speed+inc; } @Override public void applyBrakes(int dec) { //method to apply brakes speed=speed-dec; } @Override public void ringBell(int count) { //method to ring the bell for(int i=0;i
IN JAVA
Using this link to help:
Classes and Objects https://docs.oracle.com/javase/tutorial/java/javaOO/index.html
- More on Classes https://docs.oracle.com/javase/tutorial/java/javaOO/more.html
- Returning a Value from a Method https://docs.oracle.com/javase/tutorial/java/javaOO/returnvalue.html
- Using the this Keyword https://docs.oracle.com/javase/tutorial/java/javaOO/thiskey.html
- Controling Access to Members of a Class https://docs.oracle.com/javase/tutorial/java/javaOO/accesscontrol.html
- Understanding Class Members https://docs.oracle.com/javase/tutorial/java/javaOO/classvars.html
- Initializing Fields https://docs.oracle.com/javase/tutorial/java/javaOO/initial.html
- Summary https://docs.oracle.com/javase/tutorial/java/javaOO/summaryclasses.html
Instructions:
- Modify the Figures code below:
- In constructors, use for parameters the same names as the fields, and use this to access the fields.
- Make all fields private. Create getter methods to return the values of the fields. Update the main method as needed.
- For point and rectangle classes create a class variable to keep how many objects were instantiated. Create a gettter to return the number. In the main method show the value after each new element is created.
- Modify the Bicycle code below:
- In constructors, use for parameters the same names as the fields, and use this to access the fields.
- Make all fields private. Create getter methods to return the values of the fields. Update the main method as needed.
- For the generic and mountain bicycle classes create a class variable to keep how many objects were instantiated. Create a gettter to return the number. In the main method show the value after each new element is created.
- Bicycle code:
interface Bicycle{ //interface for bicycle
void changeGear(int val); //abstract methods
void changeSpeed(int inc);
void applyBrakes(int dec);
void ringBell(int count);
}
class NewCycle implements Bicycle{ //class for a new cycle
int speed=0; //stores the speed value
int gear=0; //stores the gear value
@Override
public void changeGear(int val) { //method to change the gear
gear=val;
}
@Override
public void changeSpeed(int inc) { //method to change the speed
speed=speed+inc;
}
@Override
public void applyBrakes(int dec) { //method to apply brakes
speed=speed-dec;
}
@Override
public void ringBell(int count) { //method to ring the bell
for(int i=0;i<count;i++){
System.out.print("Clang!!"+" ");
}
System.out.println("");
}
public void printState(){ //method to print the states
System.out.println("Ring bell, Speed, Gear, Brake, Ring Bell, Ring Bell");
}
}
public class BicycleDemo { //application class
public static void main(String args[]){ //main method
NewCycle obj=new NewCycle(); //object creation
obj.ringBell(1); //method call to ring the bell
obj.changeSpeed(3); //method call for changing the speed
System.out.println("Speed of bicycle: "+obj.speed);
obj.changeGear(2); //method call for changing the gear
System.out.println("Gear of bicycle: "+obj.gear);
obj.applyBrakes(1); //method call for applying brakes
System.out.println("Speed of bicycle: "+obj.speed);
obj.ringBell(2); //method call to ring the bell
obj.printState(); //method call for printing the states
}
- Figure code:
class Point{ //class for Point
int x; //stores the value of x-coordinate
int y; //stores the value of y-coordinate
String pname; //stores the name of the point
public Point(){ //default constructor
this.x=1;
this.y=1;
this.pname="Point";
}
public Point(int a,int b,String n){ //parameterized constructor
this.x=a;
this.y=b;
this.pname=n;
}
public void printPoint(){ //prints the point data
System.out.println(this.pname+"("+this.x+","+this.y+")");
}
}
class Rectangle extends Point{ //class for Rectangle
int height; //stores the height of the rectangle
int width; //stores the width of the rectangle
String rname; //stores the name of the rectangle
public Rectangle(Point p,int h,int w,String n){ //parameterized constructor
super();
this.height=h;
this.width=w;
this.rname=n;
}
public void printRect(){ //prints the rectangle data
System.out.println(this.rname+"("+this.x+","+this.y+","+this.width+","+this.height+")");
}
}
public class PointNRectangle { //application class
public static void main (String args[]){ //main method
Point pobj=new Point(5,5,"Point"); //creates an object of point class
pobj.printPoint(); //method call
Rectangle robj=new Rectangle(pobj,5,10,"Rectangle"); //creates an object of rectangle class
robj.printRect(); //method call
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

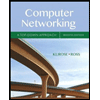
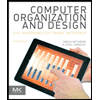
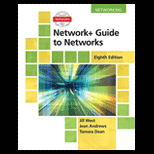
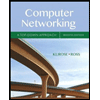
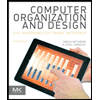
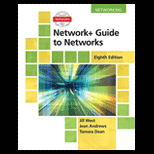
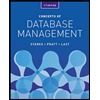
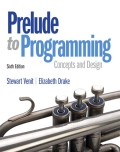
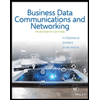