modify minishell.c to run who, ls, and date with option. who will show the count of users, ls will list files in long listing format and date will show UTC time. Modify this file so the shell can run commands with options. */ #include #include #include #include #include #include int main(int argc, char *argv[]) { char *cmd[] = {"who", "ls", "date"}; int i; while(1){ printf("0=who 1=ls 2=date : "); scanf( "%d", &i ); if(i<0 || i>2) continue; pid_t result= fork(); if(result==0) { /* child */ execlp( cmd[i], cmd[i], (char *)0 ); printf("execlp failed\n"); exit(1); } else if (result >0){ /* parent */ int status; wait(&status); } else{ //handle error } } return 0; }
modify minishell.c to run who, ls, and date with option. who will show the count of users, ls will list files in long listing format and date will show UTC time.
Modify this file so the shell can run commands with options.
*/
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <stdlib.h>
#include <fcntl.h>
int main(int argc, char *argv[])
{
char *cmd[] = {"who", "ls", "date"};
int i;
while(1){
printf("0=who 1=ls 2=date : ");
scanf( "%d", &i );
if(i<0 || i>2)
continue;
pid_t result= fork();
if(result==0) { /* child */
execlp( cmd[i], cmd[i], (char *)0 );
printf("execlp failed\n");
exit(1);
}
else if (result >0){ /* parent */
int status;
wait(&status);
}
else{
//handle error
}
}
return 0;
}

Step by step
Solved in 2 steps

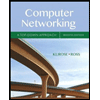
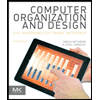
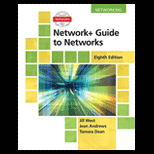
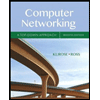
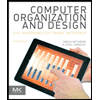
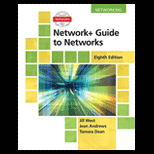
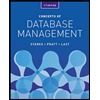
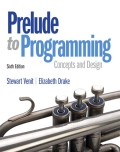
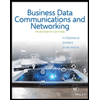