modify it based on the instructions below: -clicking a to add the amount (which shouldn't be displayed before pressing a, it should just have the info), -clicking b which will modify only the last name to uppercase (the first and last name are already uppercase before I press b which shouldn't be happening, and 'b' is used for another feature not just changing it to uppercase) maybe use an if statement and ask the user if they want to change it to uppercase for the first or last name, or -increase the hourly rate by 10 for comp sci majors. -clicking b should also add 10 to the hourly rate for computer science majors when updating data so if you could implement that please -the last thing is when clicking c, you should be able to insert a new node to the sequence with the amount calculated and display it, make it so the user can enter In the new node and have it displayed. please do it in a timely manner code below #include using namespace std; struct Employee { string firstName; string lastName; int numOfHours; float hourlyRate; char major[2]; float amount; Employee* next; }; void printRecord(Employee* e) { cout << left << setw(10) << e->lastName << setw(10) << e->firstName << setw(12) << e->numOfHours << setw(12) << e->hourlyRate << setw(10) << e->amount << setw(9) << e->major[0]<< setw(7) << e->major[1]<next != nullptr) { current = current->next; } current->next = newNode; } } void displayLinkedList(Employee* head) { Employee* current = head; if(current!=nullptr){ cout << left << setw(10) << "LastName "<< setw(10) << "FirstName " << setw(12)<<"# of Hours"<< setw(12) <<"Hourly Rate "<< setw(10) << "Amount" << setw(9) << "Comp Sci"<< setw(7) << "Comp Sec" << endl; } while (current != nullptr) { printRecord(current); current = current->next; } //have it so if the employee is comp sci major it adds 10 dollar extra per hour to their total void updateData(Employee* head) { Employee* current = head; while (current != nullptr) { transform(current->firstName.begin(), current->firstName.end(), current->firstName.begin(), ::toupper); transform(current->lastName.begin(), current->lastName.end(), current->lastName.begin(), ::toupper); // Increase hourly rate by 10 for CS majors /*Updated :: if the employee is comp sci add 1000 and if they are comp sec add 1000 and if they are both add 2000 to the total amount.*/ if (current->major[0] == 'Y') { current->amount += 1000; } if (current->major[1] == 'Y') { current->amount += 1000; } current = current->next; } } //have the user enter in a new employee node here void insertNode(Employee*& head, Employee* newNode) { if (head == nullptr || head->amount > newNode->amount) { newNode->next = head; head = newNode; } else { Employee* current = head; while (current->next != nullptr && current->next->amount < newNode->amount) { current = current->next; } newNode->next = current->next; current->next = newNode; } } int main() { Employee* head = nullptr; Employee* newNode; ifstream infile("LinkedList.txt"); if (!infile) { cerr << "Error: Input file could not be opened." << endl; return 1; } string line; while (getline(infile, line)) { istringstream iss(line); newNode = new Employee; iss >> newNode->firstName >> newNode->lastName >> newNode->numOfHours >> newNode->hourlyRate; string major1, major2; iss >> major1 >> major2; newNode->major[0] = major1[0]; newNode->major[1] = major2[0]; newNode->amount = newNode->numOfHours * newNode->hourlyRate; newNode->next = nullptr; appendNode(head, newNode); } infile.close(); updateData(head); char choice; do { cout << "\nMenu:\n"; cout << "a. Create a linked list\n"; cout << "b. Update data of the linked list\n"; cout << "c. Insert a new node to the linked list\n"; cout << "d. Delete a new node to the linked list\n"; cout << "e. Display linked list\n"; cout << "f. Exit\n"; cout << "Enter your choice: "; cin >> choice; switch (choice) { case 'a': // TODO: Read data from the "LinkedList.txt" file, create a linked list, and display the linked list break; case 'b': updateData(head); break; case 'c': { Employee* newNode = new Employee; // TODO: Read data for the new node from the user, set the newNode fields, and calculate the amount insertNode(head, newNode); break; }
modify it based on the instructions below:
-clicking a to add the amount (which shouldn't be displayed before pressing a, it should just have the info),
-clicking b which will modify only the last name to uppercase (the first and last name are already uppercase before I press b which shouldn't be happening, and 'b' is used for another feature not just changing it to uppercase) maybe use an if statement and ask the user if they want to change it to uppercase for the first or last name, or
-increase the hourly rate by 10 for comp sci majors.
-clicking b should also add 10 to the hourly rate for computer science majors when updating data so if you could implement that please
-the last thing is when clicking c, you should be able to insert a new node to the sequence with the amount calculated and display it, make it so the user can enter In the new node and have it displayed.
please do it in a timely manner
code below
#include <bits/stdc++.h>
using namespace std;
struct Employee {
string firstName;
string lastName;
int numOfHours;
float hourlyRate;
char major[2];
float amount;
Employee* next;
};
void printRecord(Employee* e) {
cout << left << setw(10) << e->lastName << setw(10) << e->firstName << setw(12)
<< e->numOfHours << setw(12) << e->hourlyRate << setw(10) << e->amount
<< setw(9) << e->major[0]<< setw(7) << e->major[1]<<endl;
}
void appendNode(Employee*& head, Employee* newNode) {
if (head == nullptr) {
head = newNode;
} else {
Employee* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
void displayLinkedList(Employee* head) {
Employee* current = head;
if(current!=nullptr){
cout << left << setw(10) << "LastName "<< setw(10) << "FirstName "
<< setw(12)<<"# of Hours"<< setw(12) <<"Hourly Rate "<< setw(10)
<< "Amount"
<< setw(9) << "Comp Sci"<< setw(7) << "Comp Sec" << endl;
}
while (current != nullptr) {
printRecord(current);
current = current->next;
}
//have it so if the employee is comp sci major it adds 10 dollar extra per hour to their total
void updateData(Employee* head) {
Employee* current = head;
while (current != nullptr) {
transform(current->firstName.begin(), current->firstName.end(), current->firstName.begin(), ::toupper);
transform(current->lastName.begin(), current->lastName.end(), current->lastName.begin(), ::toupper);
// Increase hourly rate by 10 for CS majors
/*Updated :: if the employee is comp sci add 1000 and if they are comp sec add 1000 and
if they are both add 2000 to the total amount.*/
if (current->major[0] == 'Y') {
current->amount += 1000;
}
if (current->major[1] == 'Y') {
current->amount += 1000;
}
current = current->next;
}
}
//have the user enter in a new employee node here
void insertNode(Employee*& head, Employee* newNode) {
if (head == nullptr || head->amount > newNode->amount) {
newNode->next = head;
head = newNode;
} else {
Employee* current = head;
while (current->next != nullptr && current->next->amount < newNode->amount) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
}
int main() {
Employee* head = nullptr;
Employee* newNode;
ifstream infile("LinkedList.txt");
if (!infile) {
cerr << "Error: Input file could not be opened." << endl;
return 1;
}
string line;
while (getline(infile, line)) {
istringstream iss(line);
newNode = new Employee;
iss >> newNode->firstName >> newNode->lastName >> newNode->numOfHours >> newNode->hourlyRate;
string major1, major2;
iss >> major1 >> major2;
newNode->major[0] = major1[0];
newNode->major[1] = major2[0];
newNode->amount = newNode->numOfHours * newNode->hourlyRate;
newNode->next = nullptr;
appendNode(head, newNode);
}
infile.close();
updateData(head);
char choice;
do {
cout << "\nMenu:\n";
cout << "a. Create a linked list\n";
cout << "b. Update data of the linked list\n";
cout << "c. Insert a new node to the linked list\n";
cout << "d. Delete a new node to the linked list\n";
cout << "e. Display linked list\n";
cout << "f. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 'a':
// TODO: Read data from the "LinkedList.txt" file, create a linked list, and display the linked list
break;
case 'b':
updateData(head);
break;
case 'c': {
Employee* newNode = new Employee;
// TODO: Read data for the new node from the user, set the newNode fields, and calculate the amount
insertNode(head, newNode);
break;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

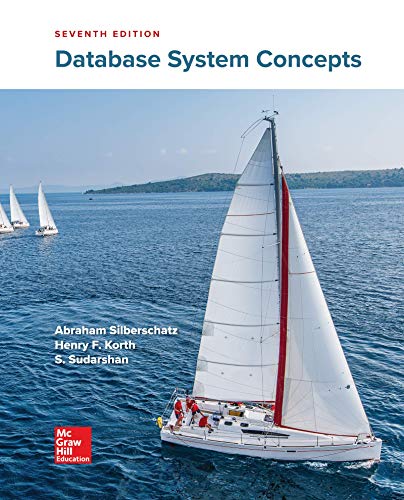
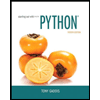
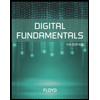
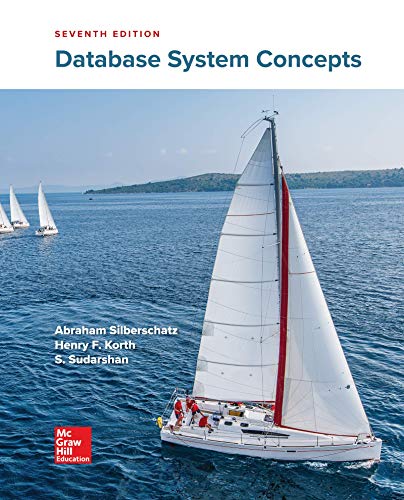
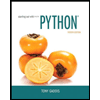
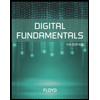
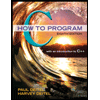
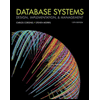
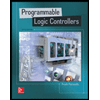