Mod 6 Lab - Ordered List ADT with Binary Search The Ordered List ADT is similar to a list, but adds the requirement that items remain sorted: add (item) - adds item to the list. • remove(item) - removes the first occurrence of item from the list. Raise a RuntimeError if the item is not present. • getitem(index) - returns the item with the given index in the sorted list. This is also known as selection. contains (item) - returns True iff there is an item of the list equal to item. iter - returns an iterator over the ordered list that yields the items in sorted order. len - returns the length of the ordered list. . We have provided a working Ordered List in lab6.py . The starter code includes 3 ways of implementing contains : _bs (???) - up to you to implement. Should be O(logn). _contains_list(item) - uses python's built-in list search. O(n). contains_bs_slow(item) - uses a binary search built on slicing. O(n). def _contains_(self, item): return self._bs (item, 0, len(self)) # alternative search algorithms: # return self._contains_list(item) # return self._contains_bs_slow(item) # Requires _bs() for this to work # uses python's default list-search # uses a slow version of binary-search (slicing)
Mod 6 Lab - Ordered List ADT with Binary Search The Ordered List ADT is similar to a list, but adds the requirement that items remain sorted: add (item) - adds item to the list. • remove(item) - removes the first occurrence of item from the list. Raise a RuntimeError if the item is not present. • getitem(index) - returns the item with the given index in the sorted list. This is also known as selection. contains (item) - returns True iff there is an item of the list equal to item. iter - returns an iterator over the ordered list that yields the items in sorted order. len - returns the length of the ordered list. . We have provided a working Ordered List in lab6.py . The starter code includes 3 ways of implementing contains : _bs (???) - up to you to implement. Should be O(logn). _contains_list(item) - uses python's built-in list search. O(n). contains_bs_slow(item) - uses a binary search built on slicing. O(n). def _contains_(self, item): return self._bs (item, 0, len(self)) # alternative search algorithms: # return self._contains_list(item) # return self._contains_bs_slow(item) # Requires _bs() for this to work # uses python's default list-search # uses a slow version of binary-search (slicing)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Answer the given question with a proper explanation and step-by-step solution.
Need lab6.py code please!

Transcribed Image Text:Mod 6 Lab - Ordered List ADT with Binary Search
The Ordered List ADT is similar to a list, but adds the requirement that items remain sorted:
• add (item) - adds item to the list.
remove(item) - removes the first occurrence of item from the list. Raise a RuntimeError if the item is not present.
• getitem(index) - returns the item with the given index in the sorted list. This is also known as selection.
contains (item) - returns True iff there is an item of the list equal to item.
.
iter - returns an iterator over the ordered list that yields the items in sorted order.
len - returns the length of the ordered list.
We have provided a working Ordered List in lab6.py. The starter code includes 3 ways of implementing contains :
_bs (???) - up to you to implement. Should be O(logn).
•
_contains_list(item) - uses python's built-in list search. O(n).
• contains_bs_slow(item) - uses a binary search built on slicing. O(n).
def _contains__(self, item):
return self._bs (item, 0, len(self))
# Requires _bs() for this to work
# uses python's default list-search
# return self._contains_bs_slow(item) # uses a slow version of binary-search (slicing)
# alternative search algorithms:
# return self._contains_list(item)
Deliverable - __bs()
Implement a O(logn) binary search. You'll need to pass left/right indices instead of list slices with each recursive call to do this.
Note that TesetLab6.py, included with the starter code, tests the contains method. If you are struggling to debug with the
tests provided, try writing your own.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
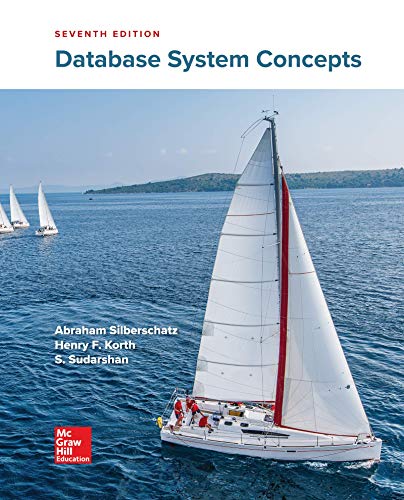
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
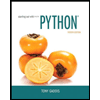
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
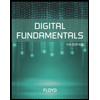
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
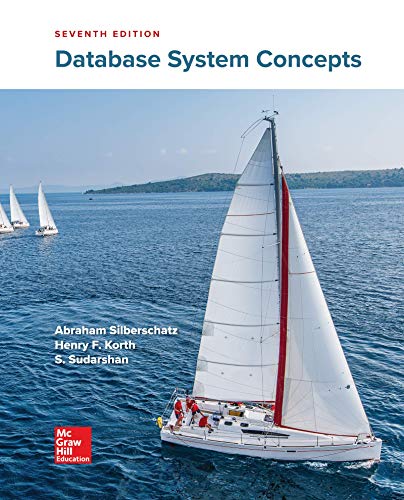
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
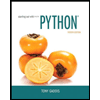
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
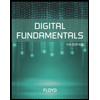
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
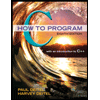
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
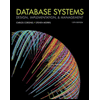
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
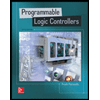
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education