Minnesota is holding elections on the day that this assignment is due! We can help them count results by writing a program. Assume that each district copiles votes into a CSV file, where each row contains one citizen's ballot data, and each column represents a different office up for election. The first row is header data indicating what office each column represents.
Minnesota is holding elections on the day that this assignment is due! We can help them count results by writing a program. Assume that each district copiles votes into a CSV file, where each row contains one citizen's ballot data, and each column represents a different office up for election. The first row is header data indicating what office each column represents.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please answer in python

Transcribed Image Text:Minnesota is holding elections on the day that this assignment is due! We can help them count results by writing a program. Assume that each district compiles votes into a CSV file, where each row contains one citizen’s ballot data, and each column represents a different office up for election. The first row is header data indicating what office each column represents.
| Mayor | County Sheriff | Governor |
|---------------|----------------|------------------|
| Jobu Tupaki | Shallan Davar | Buffy Summers |
| Evelyn Wang | Liz Lemon | Leslie Knope |
| Trevor Belmont| Liz Lemon | Gordon Freeman |
| Leslie Knope | Liz Lemon | Monkey D. Luffy |
| April Ludgate | Ron Swanson | Buffy Summers |
For example, the CSV file above would represent a district with three offices up for election, and five citizens who voted.
- The first voted for Jobu Tupaki for Mayor, Shallan Davar for Sheriff, and Buffy Summers for Governor.
- The second voted for Evelyn Wang for Mayor, Liz Lemon for Sheriff, and Leslie Knope for Governor.
And so on…
We’re going to be creating a dictionary representing the vote counts for a specific office. For example, in the spreadsheet above, if I wanted a dictionary of vote counts for the office of County Sheriff, the result would be:
`{'Shallan Davar': 1, 'Liz Lemon': 3, 'Ron Swanson': 1}`
Whereas the vote counts for Governor would be:
`{'Buffy Summers': 2, 'Leslie Knope': 1, 'Gordon Freeman': 1, 'Monkey D. Luffy': 1}`
Write a function that `count_votes(district, office)` that takes in two strings as parameters.
- `district` should be the name of a file containing all voting data for a given district, in the format specified above. You can assume that said file actually exists (no need for a try-except block).
- `office` should be the name of one of the column titles present in the CSV file. You can assume that the office passed in will match one of the columns in the CSV file.
The function should return a dictionary in which each key is a name present in the column corresponding to the given office, and the value represents how many times that name occurs within the column.
. Some fictional characters are included to simulate write-ins. The distribution of "votes" is random and should not be seen as endorsements.
### Examples
Assuming you have downloaded `hw09files.zip` and have the files in your Python working directory:
```python
>>> count_votes('district_0z.csv', 'County Sheriff')
{'Shallan Davar': 1, 'Liz Lemon': 3, 'Ron Swanson': 1}
>>> count_votes('district_4b.csv', 'Mayor')
{'Shelly Carlson': 7, 'Donna Meagle': 2, 'Kevin Nese Shores': 5}
>>> count_votes('district_60b.csv', 'County Commissioner District 4')
{'Angela Conley': 50, 'Monkey D. Luffy': 1, 'Jobu Tupaki': 2, 'Leslie Knope': 1}
```
These examples show code usage for counting votes in different districts and offices, with fictional outcomes.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F77fc5231-144e-4658-a4e4-843a776de72b%2Fef58c680-c7f8-4250-a9ec-a701e6a89851%2F4x8z8e9u_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Handling Vote Counting for Multiple Offices
When processing vote data, it is essential to ensure accuracy in counting votes for different offices. A candidate may be entered for multiple offices, so it is crucial to count occurrences in the column corresponding to the specific office you are evaluating.
### Importing Modules
You may import the `csv` module to assist with reading data, but it is not mandatory.
### Hints
- **Last Column Caution**: If splitting the file by commas, be cautious as the last column may include a newline character `\n`. This character should not be part of candidate names or office titles.
- **Comma Assumption**: You can assume names of candidates and offices do not contain commas.
### Disclaimer
The data in the CSV files is randomly generated based on names appearing on ballots from a specific district, as found on [Minnesota's official ballot website](https://myballotmn.sos.state.mn.us/). Some fictional characters are included to simulate write-ins. The distribution of "votes" is random and should not be seen as endorsements.
### Examples
Assuming you have downloaded `hw09files.zip` and have the files in your Python working directory:
```python
>>> count_votes('district_0z.csv', 'County Sheriff')
{'Shallan Davar': 1, 'Liz Lemon': 3, 'Ron Swanson': 1}
>>> count_votes('district_4b.csv', 'Mayor')
{'Shelly Carlson': 7, 'Donna Meagle': 2, 'Kevin Nese Shores': 5}
>>> count_votes('district_60b.csv', 'County Commissioner District 4')
{'Angela Conley': 50, 'Monkey D. Luffy': 1, 'Jobu Tupaki': 2, 'Leslie Knope': 1}
```
These examples show code usage for counting votes in different districts and offices, with fictional outcomes.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
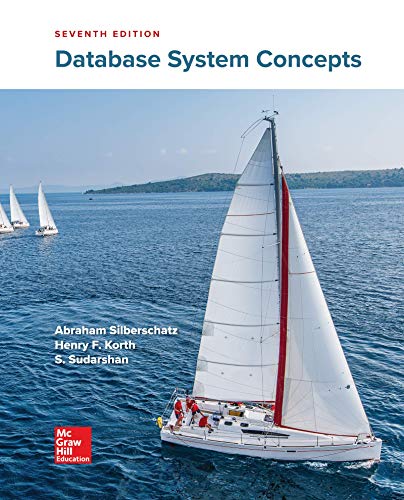
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
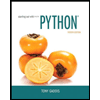
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
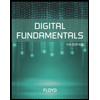
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
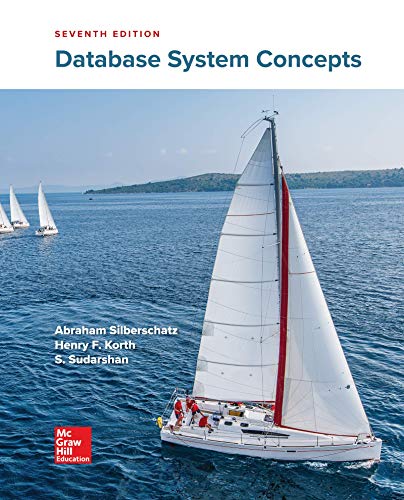
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
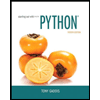
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
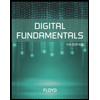
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
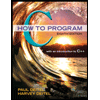
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
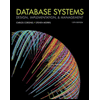
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
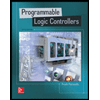
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education