MATLAB/PYTHON: Parts (d) and (e) only Please show all the steps as much as possible Be sure to follow the instructions Matlab code: function root = find_root_trisection(f, a, b, tol, max_iter) if f(a) * f(b) > 0 error('The function does not change sign in the given interval [a, b].'); end for i = 1:max_iter dx = (b - a) / 3; x1 = a + dx; x2 = b - dx; if abs(f(x1)) < tol root = x1; return; end if abs(f(x2)) < tol root = x2; return; end if f(x1) * f(a) < 0 b = x1; else a = x1; end if f(x2) * f(b) < 0 a = x2; end end error('Failed to converge to a root after %d iterations.', max_iter); end --------------------------------------------------------------------------------------------------------------- --------------------------------------------------------------------------------------------------------------- Python code: def find_root_trisection(f, a, b, tol=1e-6, max_iter=100): if f(a) * f(b) > 0: raise ValueError("The function does not change sign in the given interval [a, b].") for i in range(max_iter): dx = (b - a) / 3 x1 = a + dx x2 = b - dx if abs(f(x1)) < tol: return x1 if abs(f(x2)) < tol: return x2 if f(x1) * f(a) < 0: b = x1 else: a = x1 if f(x2) * f(b) < 0: a = x2 raise Exception(f"Failed to converge to a root after {max_iter} iterations.")
MATLAB/PYTHON:
- Parts (d) and (e) only
- Please show all the steps as much as possible
- Be sure to follow the instructions
Matlab code:
function root = find_root_trisection(f, a, b, tol, max_iter)
if f(a) * f(b) > 0
error('The function does not change sign in the given interval [a, b].');
end
for i = 1:max_iter
dx = (b - a) / 3;
x1 = a + dx;
x2 = b - dx;
if abs(f(x1)) < tol
root = x1;
return;
end
if abs(f(x2)) < tol
root = x2;
return;
end
if f(x1) * f(a) < 0
b = x1;
else
a = x1;
end
if f(x2) * f(b) < 0
a = x2;
end
end
error('Failed to converge to a root after %d iterations.', max_iter);
end
---------------------------------------------------------------------------------------------------------------
---------------------------------------------------------------------------------------------------------------
Python code:
def find_root_trisection(f, a, b, tol=1e-6, max_iter=100):
if f(a) * f(b) > 0:
raise ValueError("The function does not change sign in the given interval [a, b].")
for i in range(max_iter):
dx = (b - a) / 3
x1 = a + dx
x2 = b - dx
if abs(f(x1)) < tol:
return x1
if abs(f(x2)) < tol:
return x2
if f(x1) * f(a) < 0:
b = x1
else:
a = x1
if f(x2) * f(b) < 0:
a = x2
raise Exception(f"Failed to converge to a root after {max_iter} iterations.")
![Problem 3:
I asked ChatGPT to produce code to perform the trisection
method - i.e. the bisection method from class, but splitting in to three intervals each time
rather than two. The produced code (in Python and Matlab) can be found on Blackboard.
(a) Let f(x) = x5-3x+1. Argue (theoretically) that there is a root of this polynomial
in [0, 1].
(b) Comment the code (in Python or Matlab, your pick) and specify which stopping
procedure(s) is/are being used. Check that no errors exist in the code. Submit your
commented code as part of your solution.
(c) Run the code (in Python or Matlab, your pick) to try to find a root of f in [0, 1]. You
can use the default stopping procedure(s) in the code.
(d) Which do you think is typically more efficient, the 'bisection method' or the 'trisection
method'. Why?
(e) Let g(x) = x³ +10.5x¹+2.6525x³-22.2375x²+12.24x-1.35. By plotting g(x) (you
don't have to submit this plot), argue why we might have to be careful with running
the bisection/trisection method on the interval [0, 1].](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4f3fcee3-df4f-43f5-a70c-56c5ba155c30%2F3379dd84-adf7-477d-aba6-73837c60d4cf%2F5azlqj9_processed.png&w=3840&q=75)

Step by step
Solved in 5 steps with 2 images

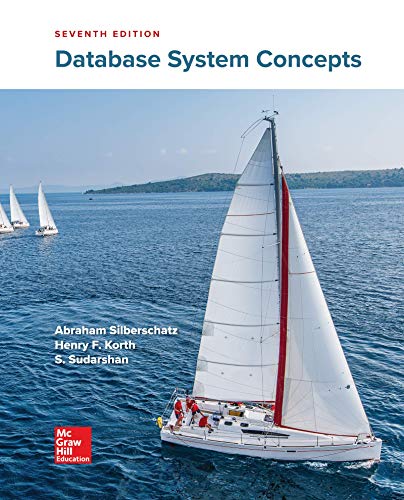
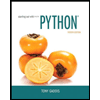
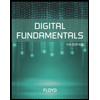
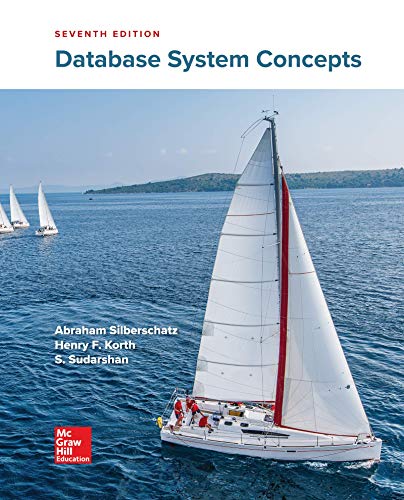
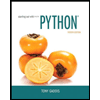
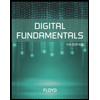
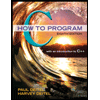
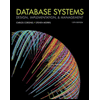
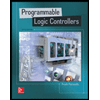