Make the BinaryTree class generic so that it uses a generic type for value rather than String. Override the toString() method to return the toString() value of its value. Implement the _inOrder(), _preOrder(), and _postOrder() methods so they are recursive. import java.util.ArrayList; import java.util.Collections; import java.util.List; public class Main { /* * Expected output: * * [4, 2, 5, 1, 3] * [1, 2, 4, 5, 3] * [4, 5, 2, 3, 1] */ public static void main(String[] args) { BinaryTree one = new BinaryTree<>("1"); BinaryTree two = new BinaryTree<>("2"); BinaryTree three = new BinaryTree<>("3"); BinaryTree four = new BinaryTree<>("4"); BinaryTree five = new BinaryTree<>("5"); one.setLeft(two); two.setLeft(four); two.setRight(five); one.setRight(three); List values = one.inOrder(); System.out.println(values); values = one.preOrder(); System.out.println(values); values = one.postOrder(); System.out.println(values); } } _____________________________________ import java.util.ArrayList; import java.util.List; public class BinaryTree { private String value; private BinaryTree left; private BinaryTree right; public BinaryTree(String value) { this.value = value; left = null; right = null; } public BinaryTree(String value, BinaryTree left, BinaryTree right) { this.value = value; this.left = left; this.right = right; } public BinaryTree getLeft() { return left; } public void setLeft(BinaryTree left) { this.left = left; } public BinaryTree getRight() { return right; } public void setRight(BinaryTree right) { this.right = right; } public String getValue() { return value; } public void setVale(String value) { this.value = value; } public List inOrder() { List values = new ArrayList(); _inOrder(this, values); return values; } private void _inOrder(BinaryTree tree, List values) { } public List preOrder() { List values = new ArrayList(); _preOrder(this, values); return values; } private void _preOrder(BinaryTree tree, List values) { } public List postOrder() { List values = new ArrayList(); _postOrder(this, values); return values; } private void _postOrder(BinaryTree tree, List values) { } }
Make the BinaryTree class generic so that it uses a generic type for value rather than String. Override the toString() method to return the toString() value of its value. Implement the _inOrder(), _preOrder(), and _postOrder() methods so they are recursive.
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class Main {
/*
* Expected output:
*
* [4, 2, 5, 1, 3]
* [1, 2, 4, 5, 3]
* [4, 5, 2, 3, 1]
*/
public static void main(String[] args) {
BinaryTree<String> one = new BinaryTree<>("1");
BinaryTree<String> two = new BinaryTree<>("2");
BinaryTree<String> three = new BinaryTree<>("3");
BinaryTree<String> four = new BinaryTree<>("4");
BinaryTree<String> five = new BinaryTree<>("5");
one.setLeft(two);
two.setLeft(four);
two.setRight(five);
one.setRight(three);
List<String> values = one.inOrder();
System.out.println(values);
values = one.preOrder();
System.out.println(values);
values = one.postOrder();
System.out.println(values);
}
}
_____________________________________
import java.util.ArrayList;
import java.util.List;
public class BinaryTree {
private String value;
private BinaryTree left;
private BinaryTree right;
public BinaryTree(String value) {
this.value = value;
left = null;
right = null;
}
public BinaryTree(String value, BinaryTree left, BinaryTree right) {
this.value = value;
this.left = left;
this.right = right;
}
public BinaryTree getLeft() {
return left;
}
public void setLeft(BinaryTree left) {
this.left = left;
}
public BinaryTree getRight() {
return right;
}
public void setRight(BinaryTree right) {
this.right = right;
}
public String getValue() {
return value;
}
public void setVale(String value) {
this.value = value;
}
public List<String> inOrder() {
List<String> values = new ArrayList<String>();
_inOrder(this, values);
return values;
}
private void _inOrder(BinaryTree tree, List<String> values) {
}
public List<String> preOrder() {
List<String> values = new ArrayList<String>();
_preOrder(this, values);
return values;
}
private void _preOrder(BinaryTree tree, List<String> values) {
}
public List<String> postOrder() {
List<String> values = new ArrayList<String>();
_postOrder(this, values);
return values;
}
private void _postOrder(BinaryTree tree, List<String> values) {
}
}

Step by step
Solved in 4 steps with 2 images

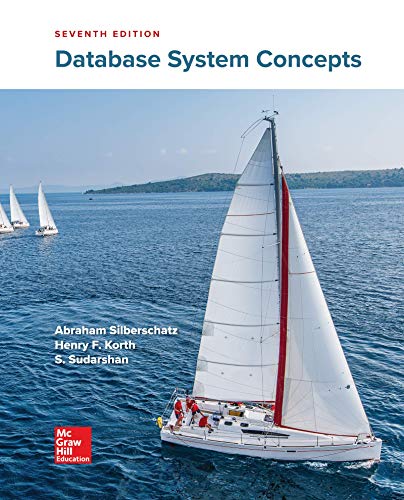
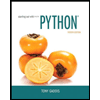
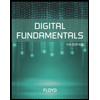
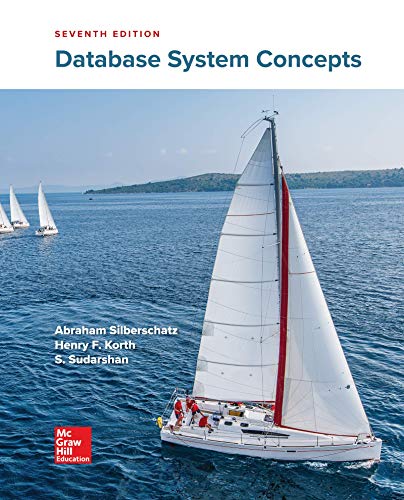
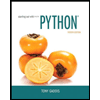
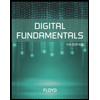
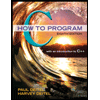
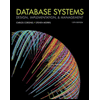
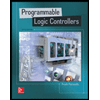