MAKE it no AI A private int data field named accountNum for the account (default 0). A private string data field named accountName for the account (default "none"). A private double data field named balance for the account (default 0). A private int data field named numTransactions that will count the number of deposit and withdraw transactions made to an account. A private Date data field named dateCreated that stores the date when the account was created. A no-arg constructor that creates a default account. A constructor that creates an account with the specified account number and name. The accessor and mutator methods for account number, name. The accessor method for balance and dateCreated. A method named withdraw that withdraws a specified amount from the account. A method named deposit that deposits a specified amount to the account. A method named displayAccountInfo that displays all the information in the Account object. A method named combine that takes another Account object as an argument and adds up balances and number of transactions to current Account object. package assignment2; public class Test { public static void main(String[] args) { Account account = new Account(1122, "my checking account"); account.deposit(3000); account.withdraw(2500); account.deposit(800); account.withdraw(200); account.displayAccountInfo(); if (account.getBalance() != 1100) { System.out.println("Something went wrong! " + account.getAccountName() + " should have 1100 CAD balance."); } System.out.println(); Account accountSavings = new Account(1133, "my savings account"); accountSavings.deposit(10000); accountSavings.deposit(2000); accountSavings.displayAccountInfo(); System.out.println(); account.combine(accountSavings); account.setAccountNum(1190); account.setAccountName("my all money at the bank"); account.displayAccountInfo(); } } "Test.java" and below output is expected: Account num: 1122Account name: my checking accountBalance: 1100.0 CAD4 transactions since created at Thu Jul 06 12:55:20 PDT 2023Account num: 1133Account name: my savings accountBalance: 12000.0 CAD2 transactions since created at Thu Jul 06 12:55:21 PDT 2023Account num: 1190Account name: my all money at the bankBalance: 13100.0 CAD6 transactions since created at Thu Jul 06 12:55:20 PDT 2023
MAKE it no AI A private int data field named accountNum for the account (default 0). A private string data field named accountName for the account (default "none"). A private double data field named balance for the account (default 0). A private int data field named numTransactions that will count the number of deposit and withdraw transactions made to an account. A private Date data field named dateCreated that stores the date when the account was created. A no-arg constructor that creates a default account. A constructor that creates an account with the specified account number and name. The accessor and mutator methods for account number, name. The accessor method for balance and dateCreated. A method named withdraw that withdraws a specified amount from the account. A method named deposit that deposits a specified amount to the account. A method named displayAccountInfo that displays all the information in the Account object. A method named combine that takes another Account object as an argument and adds up balances and number of transactions to current Account object. package assignment2; public class Test { public static void main(String[] args) { Account account = new Account(1122, "my checking account"); account.deposit(3000); account.withdraw(2500); account.deposit(800); account.withdraw(200); account.displayAccountInfo(); if (account.getBalance() != 1100) { System.out.println("Something went wrong! " + account.getAccountName() + " should have 1100 CAD balance."); } System.out.println(); Account accountSavings = new Account(1133, "my savings account"); accountSavings.deposit(10000); accountSavings.deposit(2000); accountSavings.displayAccountInfo(); System.out.println(); account.combine(accountSavings); account.setAccountNum(1190); account.setAccountName("my all money at the bank"); account.displayAccountInfo(); } } "Test.java" and below output is expected: Account num: 1122Account name: my checking accountBalance: 1100.0 CAD4 transactions since created at Thu Jul 06 12:55:20 PDT 2023Account num: 1133Account name: my savings accountBalance: 12000.0 CAD2 transactions since created at Thu Jul 06 12:55:21 PDT 2023Account num: 1190Account name: my all money at the bankBalance: 13100.0 CAD6 transactions since created at Thu Jul 06 12:55:20 PDT 2023
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
MAKE it no
- A private int data field named accountNum for the account (default 0).
- A private string data field named accountName for the account (default "none").
- A private double data field named balance for the account (default 0).
- A private int data field named numTransactions that will count the number of deposit and withdraw transactions made to an account.
- A private Date data field named dateCreated that stores the date when the account was created.
- A no-arg constructor that creates a default account.
- A constructor that creates an account with the specified account number and name.
- The accessor and mutator methods for account number, name.
- The accessor method for balance and dateCreated.
- A method named withdraw that withdraws a specified amount from the account.
- A method named deposit that deposits a specified amount to the account.
- A method named displayAccountInfo that displays all the information in the Account object.
- A method named combine that takes another Account object as an argument and adds up balances and number of transactions to current Account object.
package assignment2;
public class Test {
public static void main(String[] args) {
Account account = new Account(1122, "my checking account");
account.deposit(3000);
account.withdraw(2500);
account.deposit(800);
account.withdraw(200);
account.displayAccountInfo();
if (account.getBalance() != 1100) {
System.out.println("Something went wrong! " + account.getAccountName() + " should have 1100 CAD balance.");
}
System.out.println();
Account accountSavings = new Account(1133, "my savings account");
accountSavings.deposit(10000);
accountSavings.deposit(2000);
accountSavings.displayAccountInfo();
System.out.println();
account.combine(accountSavings);
account.setAccountNum(1190);
account.setAccountName("my all money at the bank");
account.displayAccountInfo();
}
}
"Test.java" and below output is expected:
Account num: 1122
Account name: my checking account
Balance: 1100.0 CAD
4 transactions since created at Thu Jul 06 12:55:20 PDT 2023
Account num: 1133
Account name: my savings account
Balance: 12000.0 CAD
2 transactions since created at Thu Jul 06 12:55:21 PDT 2023
Account num: 1190
Account name: my all money at the bank
Balance: 13100.0 CAD
6 transactions since created at Thu Jul 06 12:55:20 PDT 2023
Account name: my checking account
Balance: 1100.0 CAD
4 transactions since created at Thu Jul 06 12:55:20 PDT 2023
Account num: 1133
Account name: my savings account
Balance: 12000.0 CAD
2 transactions since created at Thu Jul 06 12:55:21 PDT 2023
Account num: 1190
Account name: my all money at the bank
Balance: 13100.0 CAD
6 transactions since created at Thu Jul 06 12:55:20 PDT 2023
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
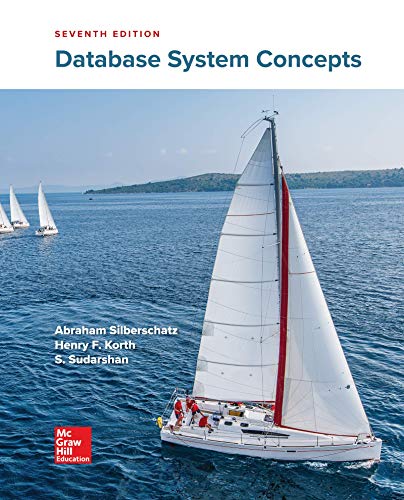
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
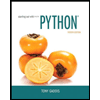
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
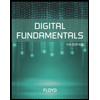
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
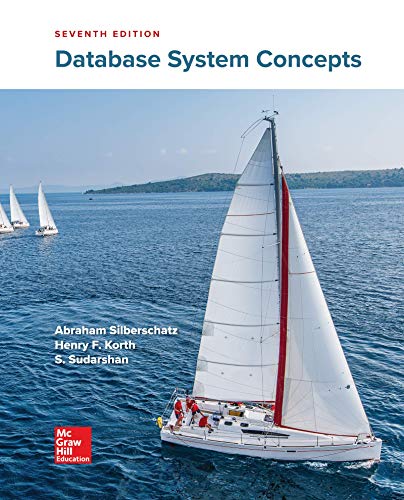
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
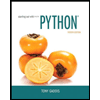
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
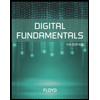
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
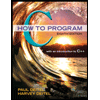
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
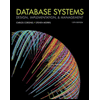
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
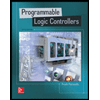
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education